diff --git "a/AVL Tree/AVL \355\212\270\353\246\254 \354\213\244\354\240\204 \354\230\210\354\240\234.md" "b/AVL Tree/AVL \355\212\270\353\246\254 \354\213\244\354\240\204 \354\230\210\354\240\234.md"
new file mode 100644
index 0000000..2511b31
--- /dev/null
+++ "b/AVL Tree/AVL \355\212\270\353\246\254 \354\213\244\354\240\204 \354\230\210\354\240\234.md"
@@ -0,0 +1,47 @@
+# AVL 트리 실전 예제
+AVL 트리 실전적인 풀이에서 신경 써야 할 부분은 딱 개이다.
+1. RL, LR 등의 판단은 처음 BF가 깨진 곳에서 판단.
+2. **BF가 깨진 곳이 부모-자식간에 이어서 여러 곳에서 발생했다면, 아래쪽을 선택해서 재분배한다**
+3. LR, RL 회전시 그냥 세 노드에 대해 **중간 값 노드를 루트로 해서, 그 노드를 기준으로 다시 서브트리를 만든다.**
+
+## 예제 1
+{MAR, MAY, NOV, AUG, APR, JAN, DEC, JUL, FEB, JUN, OCT, SEP}
+
+기본적으로 책에서 제공된 순서이다. 여러번 연습했다.
+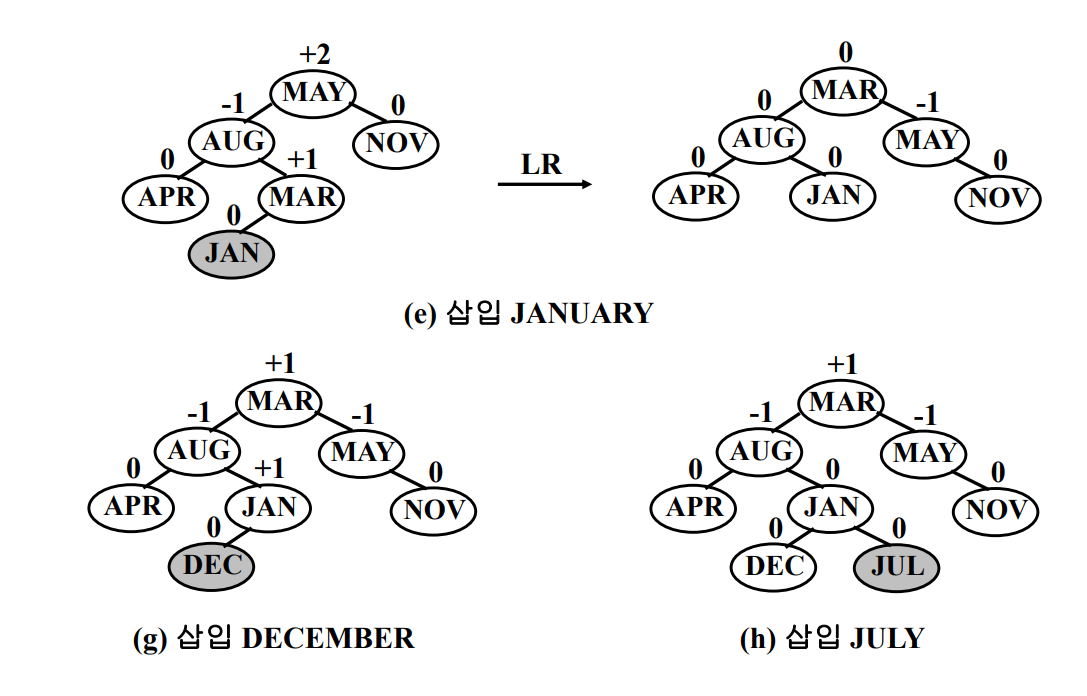
+
+
+
+1. 첫 LR 회전이다. **BF가 깨진 루트를 기준으로** LR로 판단한 것을 확인할 수 있다.
+2. MAY, AUG, MAR에 대해 중간값인 MAR이 루트가 되어 새로 트리가 짜인 것을 확인할 수 있다.
+3. 왜 MAY 부터 AUG, MAR로 골랐는가? **깨진 곳이 기준이니까**
+
+
+
+#### 여기도 중요하다. BF 깨진 곳이 두 곳!
+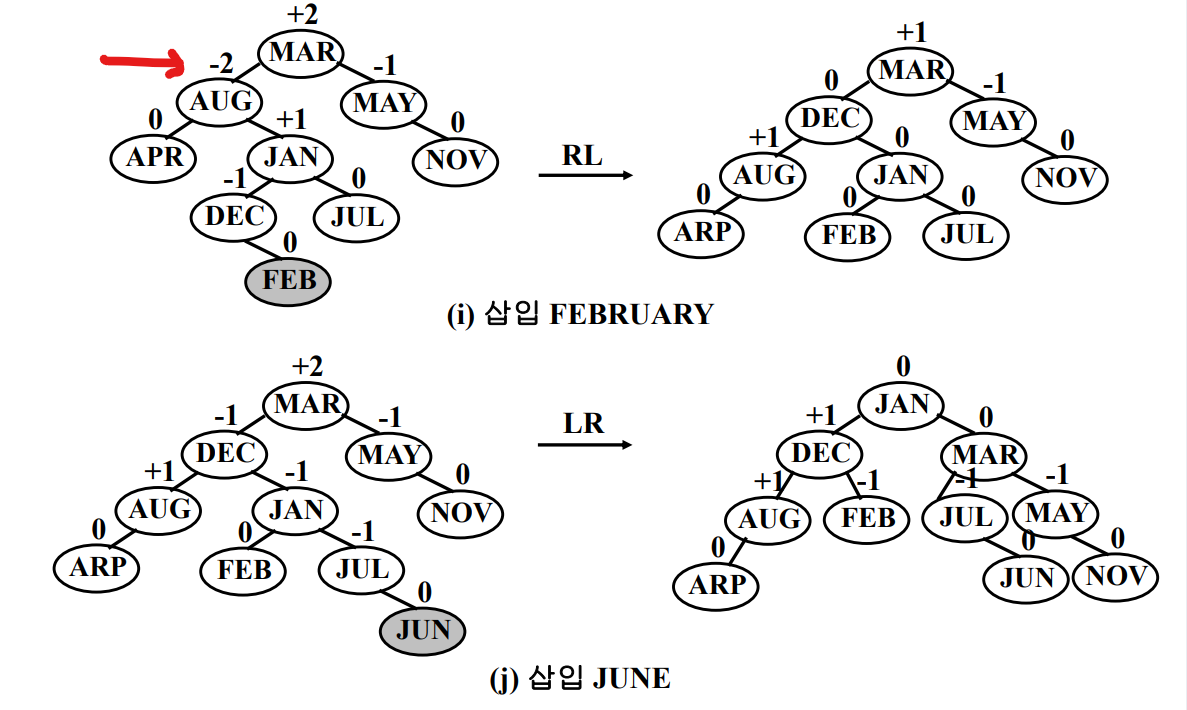
+
+
+1. **위에서 언급한 것 처럼 MAR, AUG 둘 다 깨졌지만, AUG를 기준으로 회전한다.**
+2. **둘 다 깨졌으면 자식쪽!!!** 그래서 **RL회전이라고 부른다!!**
+3. AUG, JAN, DEC를 기준으로 회전하는데, 중간값인 DEC를 루트로 새로 서브트리들을 작성한다
+
+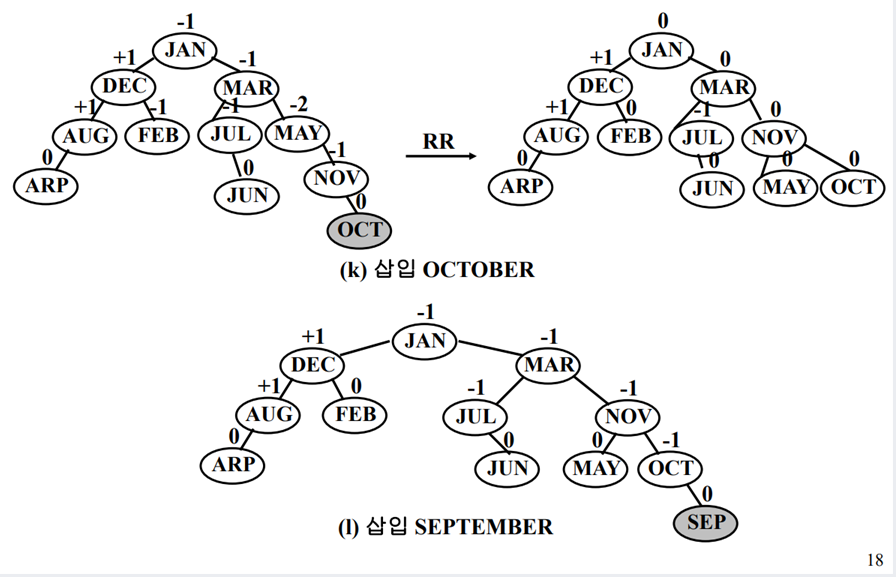
+
+완성된 모습..
+
+## 예제 2
+{'NOV', 'APR', 'OCT', 'MAY', 'DEC', 'AUG', 'SEP', 'JAN', 'JUL', 'JUN', 'MAR', 'FEB'}의 순서로 입력이 들어온 상황.
+
+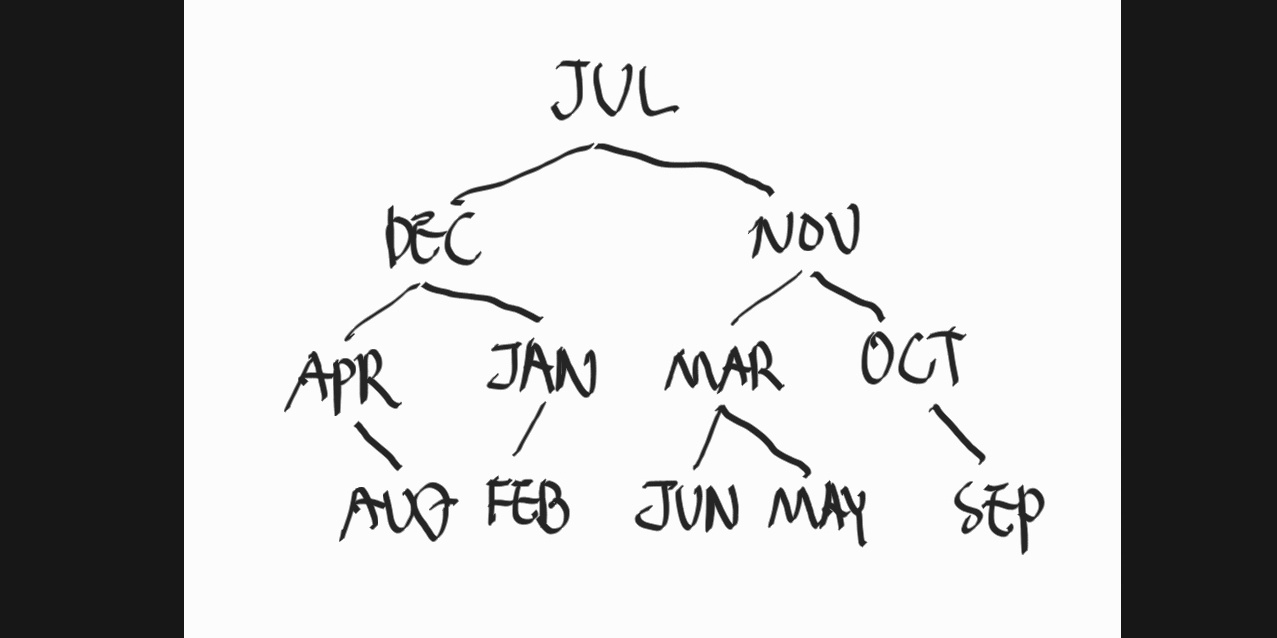
+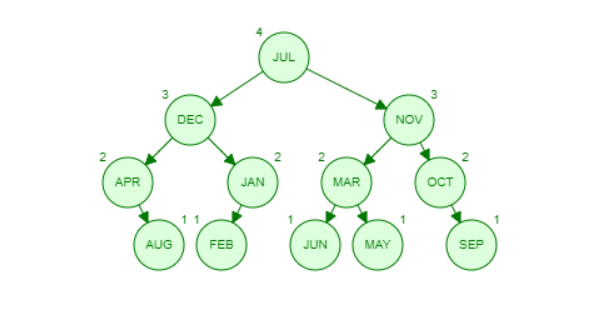
+
+위의 사진은 직접 그려본 것이고, 아래 사진은 visualizer를 사용한 결과이다. 같은 key값의 집합에 대해, 같은 결과를 만든 것을 확인할 수 있다.
+
+### 결론:
+1. 같은 정책 하의 같은 key 구성이여도 입력 순서가 다르면, 트리가 다를 수 있다.
+2. 동일 key값이 존재한다면 동일 key값 처리 정책에 따라 트리가 달라질 수 있다.
+
+## Reference
+- Fundamentals of Data Structures in C++ \
+- [AVL Tree Visualizer](https://www.cs.usfca.edu/~galles/visualization/AVLtree.html)
diff --git "a/AVL Tree/\354\275\224\353\223\234/AVL.cpp" "b/AVL Tree/\354\275\224\353\223\234/AVL.cpp"
new file mode 100644
index 0000000..5fbee17
--- /dev/null
+++ "b/AVL Tree/\354\275\224\353\223\234/AVL.cpp"
@@ -0,0 +1,139 @@
+//
+// Created by Jinho on 12/14/2022.
+//
+#include "AVL.h"
+
+Node::Node(int value) {
+ data = value;
+ leftChild = rightChild = NULL;
+ height = 1;
+ balanceFactor = 0;
+}
+
+void bstree::visit(Node* ptr) {
+ cout << ptr->data << "\t";
+ if (ptr->leftChild) cout << "left : " << ptr->leftChild->data << '\t';
+ else cout << "left : empty\t";
+ if (ptr->rightChild) cout << "right : " << ptr->rightChild->data << '\t';
+ else cout << "right : empty\t";
+ cout << '\n';
+}
+
+void bstree::show(Node* root) {
+ cout << '\n';
+ queue bfsQueue;
+ bfsQueue.push(root);
+ Node *nodeNow;
+ while (!bfsQueue.empty()) {
+ nodeNow = bfsQueue.front();
+ bfsQueue.pop();
+ visit(nodeNow);
+ if (nodeNow->leftChild) bfsQueue.push(nodeNow->leftChild);
+ if (nodeNow->rightChild) bfsQueue.push(nodeNow->rightChild);
+ }
+}
+
+Node *bstree::insert(const int value, Node *&nodeNow) {
+ if (!nodeNow) return nodeNow = new Node(value);
+ if (value == nodeNow->data) return nodeNow;
+ if (value < nodeNow->data) nodeNow->leftChild = insert(value, nodeNow->leftChild);
+ if (value > nodeNow->data) nodeNow->rightChild = insert(value, nodeNow->rightChild);
+
+ setBF(nodeNow);
+ if (nodeNow->balanceFactor < -1 || nodeNow->balanceFactor > 1) return rotation(nodeNow, value);
+ return nodeNow;
+}
+
+bool bstree::search(const int key, Node* root) {
+ cout << root->data;
+
+ if (root->data == key) {
+ cout << '\n';
+ return true;
+ }
+
+ cout << " -> ";
+
+ Node *nodeNext = key <= root->data ? root->leftChild : root->rightChild;
+ if (!nodeNext) {
+ cout << " X (there is no nodes that has value " << key << ") \n";
+ return false;
+ }
+
+ return search(key, nodeNext);
+}
+
+Node *bstree::del(const int key, Node* nodeNow) {
+ if (!nodeNow) return nodeNow;
+ if (key < nodeNow->data) nodeNow->leftChild = del(key, nodeNow->leftChild);
+ else if (key > nodeNow->data) nodeNow->rightChild = del(key, nodeNow->rightChild);
+ else {
+ // find the node
+ if (!nodeNow->leftChild && !nodeNow->rightChild) nodeNow = NULL;
+ else if (!nodeNow->leftChild) nodeNow = nodeNow->rightChild;
+ else if (!nodeNow->rightChild) nodeNow = nodeNow->leftChild;
+ else {
+ Node *ptr = nodeNow->leftChild;
+ while (ptr->rightChild) {
+ ptr = ptr->rightChild;
+ }
+ nodeNow->data = ptr->data;
+ del(nodeNow->data, nodeNow->leftChild);
+ }
+ }
+ if (!nodeNow) return nodeNow;
+
+ setBF(nodeNow);
+ if (nodeNow->balanceFactor < -1 || nodeNow->balanceFactor > 1) return rotation(nodeNow, key);
+ return nodeNow;
+}
+
+void bstree::setBF(Node* startNode) {
+ int leftHeight, rightHeight;
+ leftHeight = startNode->leftChild ? startNode->leftChild->height : 0;
+ rightHeight = startNode->rightChild ? startNode->rightChild->height : 0;
+ startNode->height = leftHeight < rightHeight ? rightHeight + 1 : leftHeight + 1;
+ startNode->balanceFactor = leftHeight - rightHeight;
+}
+
+Node *bstree::rotation(Node* startNode, int value) {
+ // 1. LL rotation
+ Node *tempNode;
+ if (startNode->leftChild && value <= startNode->leftChild->data) {
+ tempNode = startNode->leftChild;
+ rotation(startNode, startNode->leftChild);
+ return tempNode;
+ }
+
+ // 2. RR rotation
+ if (startNode->rightChild && startNode->rightChild->data < value) {
+ tempNode = startNode->rightChild;
+ rotation(startNode, startNode->rightChild);
+ return tempNode;
+ }
+
+ // 3. LR rotation
+ if (value <= startNode->data) {
+ tempNode = startNode->leftChild->rightChild;
+ rotation(startNode->leftChild, startNode->leftChild->rightChild);
+ rotation(startNode, startNode->leftChild);
+ }
+
+ // 4. RL rotation
+ if (startNode->data < value) {
+ tempNode = startNode->rightChild->leftChild;
+ rotation(startNode->rightChild, startNode->rightChild->leftChild);
+ rotation(startNode, startNode->rightChild);
+ }
+ return tempNode;
+}
+
+void bstree::rotation(Node* start, Node* end) {
+ if (start->leftChild == end) {
+ start->leftChild = end->rightChild;
+ end->rightChild = start;
+ } else {
+ start->rightChild = end->leftChild;
+ end->leftChild = start;
+ }
+}
diff --git "a/AVL Tree/\354\275\224\353\223\234/AVL.h" "b/AVL Tree/\354\275\224\353\223\234/AVL.h"
new file mode 100644
index 0000000..3f9098d
--- /dev/null
+++ "b/AVL Tree/\354\275\224\353\223\234/AVL.h"
@@ -0,0 +1,29 @@
+//
+// Created by Jinho on 12/14/2022.
+//
+
+#ifndef AVL_H
+#define AVL_H
+typedef struct Node* nodeptr;
+#include
+#include
+using namespace std;
+struct Node {
+ int data, height, balanceFactor;
+ Node *leftChild, *rightChild;
+ Node(int value);
+};
+
+class bstree {
+public:
+ void visit(Node* ptr);
+ Node *insert(const int value, Node*& root);
+ void show(Node* root);
+ bool search(const int key, Node* root);
+ Node *del(const int key, Node* root);
+ void setBF(Node* startNode);
+ void rotation(Node* start, Node* end);
+ Node *rotation(Node* startNode, int value);
+};
+
+#endif //ALGORITHEM_AVL_H
diff --git "a/AVL Tree/\354\275\224\353\223\234/hw12.cpp" "b/AVL Tree/\354\275\224\353\223\234/hw12.cpp"
new file mode 100644
index 0000000..2ba6c7a
--- /dev/null
+++ "b/AVL Tree/\354\275\224\353\223\234/hw12.cpp"
@@ -0,0 +1,60 @@
+#include "AVL.h"
+int main() {
+nodeptr root;
+ int a, choice, findele, delele;
+ bstree bst;
+ bool flag = true;
+ root = NULL;
+ bst.insert(19, root);
+ bst.insert(10, root);
+ bst.insert(46, root);
+ bst.insert(4, root);
+ bst.insert(14, root);
+ bst.insert(37, root);
+ bst.insert(55, root);
+ bst.insert(7, root);
+ bst.insert(12, root);
+ bst.insert(18, root);
+ bst.insert(28, root);
+ bst.insert(40, root);
+ bst.insert(51, root);
+ bst.insert(61, root);
+ bst.insert(21, root);
+ bst.insert(32, root);
+ bst.insert(49, root);
+ bst.insert(58, root);
+
+ while (flag) {
+ cout << "Enter the choice: (1 : search, 2 : add, 3 : delete, 4 : show, 0 : exit)";
+ cin >> choice;
+
+ switch (choice) {
+ case 1:
+ cout << "Enter node to search:";
+ cin >> findele;
+ if (root != NULL) bst.search(findele, root);
+ break;
+ case 2:
+ cout << "Enter a new value:";
+ cin >> a;
+ bst.insert(a, root);
+ bst.show(root);
+ break;
+ case 3:
+ cout << "Enter node to delete:";
+ cin >> delele;
+ bst.del(delele, root);
+ bst.show(root);
+ break;
+ case 4:
+ if (root != NULL) bst.show(root);
+ break;
+ case 0:
+ cout << "\n\tThank your for using AVL maxTree program\n" << endl;
+ flag = false;
+ break;
+ default: cout << "Sorry! wrong input\n" << endl; break;
+ }
+ }
+ return 0;
+}
diff --git a/Heap/Max Heap Code/hw9.cpp b/Heap/Max Heap Code/hw9.cpp
new file mode 100644
index 0000000..2c14b67
--- /dev/null
+++ b/Heap/Max Heap Code/hw9.cpp
@@ -0,0 +1,31 @@
+#include "maxheap.h"
+#include
+int main(int argc, char *argv[])
+{
+ int N, i, data;
+ Maxheap H(10);
+ if(argc != 2)
+ {
+ cerr << "wrong argument count" << endl; return 1;
+ }
+ fstream fin(argv[1]);
+ if(!fin)
+ {
+ cerr << argv[1] << " open failed" << endl;
+ return 1;
+ }
+ fin >> N; for(i=0; i> data;
+ H.Push(data);
+ }
+
+ cout << H; while(!H.IsEmpty())
+ {
+
+ cout << H.Top() << " " ; H.Pop();
+ }
+ cout << endl;
+ fin.close();
+ return 0;
+}
diff --git a/Heap/Max Heap Code/maxheap.h b/Heap/Max Heap Code/maxheap.h
new file mode 100644
index 0000000..2ae4a5f
--- /dev/null
+++ b/Heap/Max Heap Code/maxheap.h
@@ -0,0 +1,108 @@
+#ifndef MAXHEAP_H
+#define MAXHEAP_H
+#include
+
+using namespace std;
+
+template
+class Maxheap {
+private:
+ void ChangeSize1D(int);
+ T* heap;
+ int heapSize;
+ int capacity;
+public:
+ Maxheap(int);
+ void Push(const T &);
+
+ void Pop();
+ bool IsEmpty() {return heapSize == 0;}
+ T Top() {return heap[1];}
+ template friend ostream &operator <<(ostream &, Maxheap &);
+};
+
+template
+ostream &operator <<(ostream &os, Maxheap &H)
+{
+ os << " ";
+ int i;
+ for(i=1; i<=H.heapSize; i++)
+ os << i << ":" << H.heap[i] << " ";
+ os << endl;
+ return os;
+}
+
+template
+Maxheap::Maxheap(int _capacity = 10) : heapSize(0)
+{
+ if(_capacity < 1)
+ throw " Must be > 0";
+ capacity = _capacity;
+ heap = new T[capacity+1];
+}
+
+template
+void Maxheap::ChangeSize1D(int size)
+{
+ T temp[size+1];
+ for (int i = 1; i < capacity; i++) {
+ temp[i] = heap[i];
+ }
+ heap = temp;
+ capacity = size;
+}
+
+template
+void Maxheap::Push(const T &value) {
+ if (heapSize == capacity) ChangeSize1D(2*capacity);
+
+ heapSize++;
+ if (heapSize == 1) {
+ heap[1] = value;
+ } else {
+ T parent = heap[heapSize >> 1];
+
+ int idx = heapSize;
+ T child = heap[idx];
+ while (idx != 1 && heap[idx / 2] < heap[idx]) {
+ int temp = heap[idx / 2];
+ heap[idx / 2] = heap[idx];
+ heap[idx] = temp;
+
+ idx = idx / 2;
+ }
+ }
+}
+
+template
+void Maxheap::Pop() {
+ if(heapSize <= 0) {
+ return;
+ } else if (heapSize == 1) {
+ heapSize--;
+ return heap[1];
+ }
+ heap[1] = heap[heapSize];
+ heapSize--;
+
+ int idx = 1;
+ while(idx*2 <= heapSize) {
+ int child;
+ if (idx*2 + 1 > heapSize) {
+ child = idx * 2;
+ } else {
+ child = heap[idx * 2] > heap[idx * 2 + 1] ? idx * 2 : idx * 2 + 1;
+ }
+
+ if(heap[child] <= heap[idx]) break;
+ int temp = heap[child];
+ heap[child] = heap[idx];
+ heap[idx] = temp;
+
+ idx = child;
+ }
+}
+
+
+
+#endif
diff --git a/Linked List/list.cpp b/Linked List/list.cpp
new file mode 100644
index 0000000..f0b3176
--- /dev/null
+++ b/Linked List/list.cpp
@@ -0,0 +1,65 @@
+#include
+#include "list.h"
+
+ostream& operator<<(ostream& os, IntList& il) {
+ Node *ptr = il.first;
+ while (ptr != NULL) {
+ os << ptr->data << " "; ptr = ptr->link;
+ }
+ os << endl;
+ return os;
+}
+
+void IntList::Push_Back(int e) {
+ if (!first)
+ first = last = new Node(e);
+ else {
+ last->link = new Node(e);
+ last = last->link;
+ }
+}
+void IntList::Push_Front(int e) {
+ if (!first) {
+ first = last = new Node(e);
+ } else {
+ Node* new_first = new Node(e);
+ new_first->link = first;
+ first = new_first;
+ }
+}
+void IntList::Insert(int e) {
+ if (!first) {
+ first = last = new Node(e);
+ }
+ else if (first->data >= e){
+ Push_Front(e);
+ }
+ else{
+ Node *ptr = first;
+
+ while (ptr->link != NULL && ptr->link->data < e) {
+ ptr = ptr->link;
+ }
+
+ if (ptr->link != NULL) {
+ Node *new_node = new Node(e);
+ new_node->link = ptr->link;
+ ptr->link = new_node;
+ } else Push_Back(e);
+ }
+}
+
+void IntList::Delete(int e) {
+ if(first){
+ if(first->data == e) {
+ first = first->link;
+ } else{
+ Node *ptr = first;
+ while (ptr-> link != NULL && ptr->link->data != e) ptr = ptr->link;
+ if(ptr->link != NULL) {
+ if (ptr->link == last) last = ptr;
+ ptr->link = ptr->link->link;
+ }
+ }
+ }
+}
diff --git a/Linked List/list.h b/Linked List/list.h
new file mode 100644
index 0000000..063069c
--- /dev/null
+++ b/Linked List/list.h
@@ -0,0 +1,21 @@
+using namespace std;
+struct Node
+{
+ Node(int d = 0, Node* l = NULL) : data(d), link(l) { }
+ int data;
+ Node * link;
+};
+
+struct IntList
+{
+ IntList() { last = first = NULL; }
+
+ void Push_Back(int); // 리스트 뒤에 삽입 중복 허용
+ void Push_Front(int); // 리스트 앞에 삽입 중복 허용
+ void Insert(int); // 정렬되어 있다는 가정 하에 제 위치에 삽입
+ void Delete(int); // 리스트의 원소 삭제
+ Node *first; // 첫 노드를 가리킴
+ Node *last; // 마지막 노드를 가리킴
+};
+
+ostream& operator<< (ostream&, IntList&);
diff --git a/Linked List/main.cpp b/Linked List/main.cpp
new file mode 100644
index 0000000..84fd849
--- /dev/null
+++ b/Linked List/main.cpp
@@ -0,0 +1,34 @@
+#include
+#include"list.h"
+using namespace std;
+
+int main() {
+ IntList il;
+ int input;
+
+ cout<<"==============Input============="<>input;
+ if(input==-1) break;
+ il.Insert(input);
+ cout<>input;
+ if(input==-1) break;
+ il.Delete(input);
+ cout << il;
+ }
+
+ cout<<"===========Push_Front==========="<>input; il.Push_Front(input); cout<>input; il.Push_Front(input); cout<>input; il.Push_Back(input);cout << il;
+ cin>>input; il.Push_Back(input);cout << il;
+ return 0;
+}
diff --git "a/Permutations \354\213\244\354\240\204 \354\230\210\354\240\234.md" "b/Permutations \354\213\244\354\240\204 \354\230\210\354\240\234.md"
new file mode 100644
index 0000000..3d125c1
--- /dev/null
+++ "b/Permutations \354\213\244\354\240\204 \354\230\210\354\240\234.md"
@@ -0,0 +1,64 @@
+# Permutations 실전 예제
+모든 이진 트리는 **유일한 전위-중위 순서 쌍을 가진다.**
+따라서 전위-중위 순서쌍이 주어진다면 트리를 그릴 수 있다!
+전략은 **서브 트리의 루트를 파악하고, 서브 트리를 계속 그려나가는 것을 계속해서 반복한다!**
+1. 전위 순서를 통해 서브 트리의 루트를 확인한다.
+2. 중위 순서를 통해 앞서 찾아낸 루트의 왼쪽 서브 트리, 오른쪽 서브 트리를 파악한다.
+3. 2번에서 찾아낸 서브트리들의 루트를 전위 순서를 통해 확인한다.
+4. 무한 반복....
+
+(참고)
+- 전위 순열: 이진트리를 전위 순회에 따라 방문한 노드들의 순서
+- 중위 순열: 이진트리를 중위 순회에 따라 방문한 노드들의 순서
+
+
+## 실전 예제!
+주어진 전위 순서와 중위 순서로 원본 트리를 그려보자!
+- 전위 순서: A-B-C-D-E-F-G-H-I
+- 중위 순서: B-C-A-E-D-G-H-F-I
+
+1. **전위 순서를 통해 루트가 A임을 파악**
+2. **후위 순서에서 A를 찾는다!**
왼편에는 B, C로 구성된 서브 트리! 오른쪽에는 E, D, G, H, F, I로 구성된 서브트리가 있음을 추측할 수 있다.
+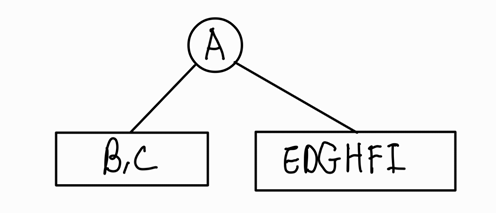
+
+
+
+3. 이후 B-C의 배치를 추측하는데, **전위 순서에서 B가 먼저 나오므로 B가 왼쪽 서브트리의 루트임**
+
+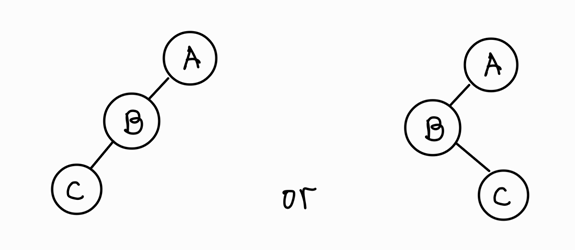
+4. **중위 순서에서 B-C로 발견되므로, 왼쪽을 틀림! 오른쪽과 같이 구성된다는 것을 알 수 있다!**
+
+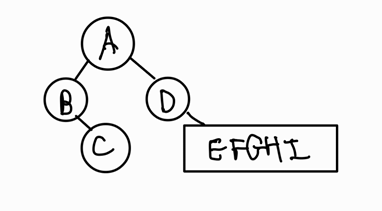
+완성된 모습...
+
+
+5. **전위 순서를 통해 E-D-G-H-F-I의 루트가 D임을 확인!**
+6. 그리고 **중위 순서를 통해 D를 루트로 왼쪽은 E 하나만 있고, 오른쪽은 F-G-H-I가 있음을 추측 가능!**
+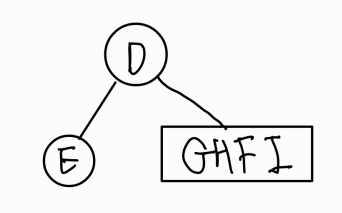
+
+
+
+7. **전위 순서를 통해 F가 루트임을 확인, 중위 순서를 통해 왼쪽에 G, H 오른 쪽에 I가 옴을 확인**
+8. **G-H는 전위 순서를 통해 G가 루트임을 알 수 있었고, 중위 순서를 통해 H는 오른쪽 서브트리에 위치함을 추측 가능하다.**
+
+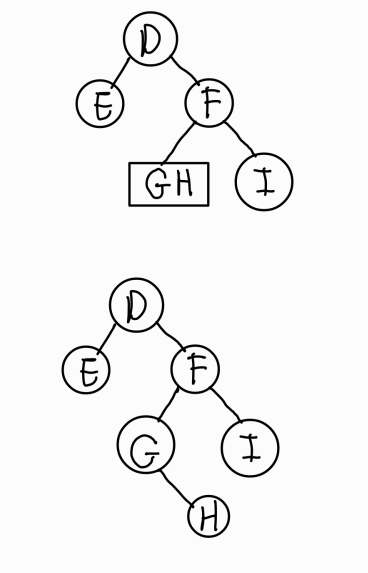
+
+
+
+
+이렇게 전부 합쳐주면 트리가 완성된다!
+
+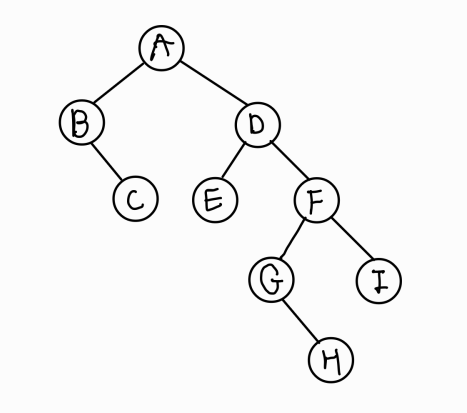
+
+
+## 중위 순열의 갯수
+1. **n개의 노드를 가진 서로 다른 이진트리의 수**
+2. **1, 2, ..., n의 전위 순열을 가지는 이진트리로 부터 얻을 수 있는 중위 순열의 수**
+3. 1 부터 n까지의 수를 스택에 넣었다가 가능한 모든 방법으로 삭제하여 만들 수 있는 상이한 순열의 수
+
+
+**노하우는, N개의 노드를 가진 이진 트리가 생길 수 있는 모양꼴을 전부 떠올려 본 다음 숫자를 채워 넣는 것이다. 3개의 경우 5개 정도 된다..**
+
+
+## Reference
+- Fundamentals of Data Structures in C++ \
diff --git a/Polynomial/polya.cpp b/Polynomial/polya.cpp
new file mode 100644
index 0000000..16651c3
--- /dev/null
+++ b/Polynomial/polya.cpp
@@ -0,0 +1,92 @@
+#include "polya.h"
+#include
+using namespace std;
+
+istream& operator>> (istream& is, Polynomial& p) {
+ int noofterms; float coef; int exp;
+ is >> noofterms;
+ for (int i = 0; i < noofterms; i++) {
+ is >> coef >> exp;
+ p.NewTerm(coef, exp);
+ }
+ return is;
+}
+
+
+ostream& operator<<(ostream& os, Polynomial& p) {
+ string str;
+ for(int i = 0; i < p.terms; i++) {
+ Term termNow = p.termArray[i];
+ if (i != 0) {
+ if(termNow.coef > 0) str += "+";
+ else str += "-";
+ }
+ if (termNow.coef != 1 && termNow.coef != -1) str += (abs((int) termNow.coef)) + '0';
+ if(i != p.terms - 1) str += "x";
+ if (termNow.exp != 1 && termNow.exp != 0) {
+ str += "^";
+ if (termNow.exp < 0) {
+ str += "(-";
+ str += (abs((int) termNow.exp)) + '0';
+ str += ")";
+ } else {
+ str += (abs((int) termNow.exp)) + '0';
+ }
+ }
+ str += " ";
+ }
+ return os << str << endl;
+}
+
+Polynomial::Polynomial() :capacity(1), terms(0) {
+ termArray = new Term[capacity];
+}
+
+void Polynomial::NewTerm(const float theCoeff, const int theExp) {
+ if (terms == capacity) {
+ capacity *= 2;
+ Term* temp = new Term[capacity];
+ copy(termArray, termArray + terms, temp);
+ delete[] termArray;
+ termArray = temp;
+ }
+ termArray[terms].coef = theCoeff;
+ termArray[terms++].exp = theExp;
+}
+
+Polynomial Polynomial::operator+(Polynomial& b) {
+ Polynomial c;
+ int aPos = 0, bPos = 0;
+ while ((aPos < terms) && (bPos < b.terms)) {
+ if (termArray[aPos].exp == b.termArray[bPos].exp) {
+ float t = termArray[aPos].coef + b.termArray[bPos].coef;
+ if (t) {
+ c.NewTerm(t, termArray[aPos].exp);
+ aPos++;
+ bPos++;
+ }
+ }
+ else if (termArray[aPos].exp < b.termArray[bPos].exp) {
+ c.NewTerm(b.termArray[bPos].coef, b.termArray[bPos].exp);
+ bPos++;
+ }
+ else {
+ c.NewTerm(termArray[aPos].coef, termArray[aPos].exp);
+ aPos++;
+ }
+ }
+ for (; aPos < terms; aPos++) {
+ c.NewTerm(termArray[aPos].coef, termArray[aPos].exp);
+ }
+ for (; bPos < b.terms; bPos++) {
+ c.NewTerm(b.termArray[bPos].coef, b.termArray[bPos].exp);
+ }
+ return c;
+}
+
+int main() {
+ Polynomial p1, p2;
+ cin >> p1 >> p2;
+ Polynomial p3 = p1 + p2;
+ cout << p1 << p2 << p3;
+}
diff --git a/Polynomial/polya.h b/Polynomial/polya.h
new file mode 100644
index 0000000..1fbb1ba
--- /dev/null
+++ b/Polynomial/polya.h
@@ -0,0 +1,30 @@
+#ifndef POLYNOMIAL_H
+#define POLYNOMIAL_H
+#include
+
+using namespace std;
+
+class Polynomial;
+class Term {
+ friend class Polynomial;
+ friend ostream& operator<<(ostream&, Polynomial&);
+ friend istream& operator>>(istream&, Polynomial&);
+private:
+ float coef;
+ int exp;
+};
+
+class Polynomial {
+public:
+ Polynomial();
+ Polynomial operator+(Polynomial&);
+ void NewTerm(const float, const int);
+ friend ostream& operator<<(ostream&, Polynomial&);
+ friend istream& operator>>(istream&, Polynomial&);
+private:
+ Term *termArray;
+ int capacity;
+ int terms;
+};
+#endif
+
diff --git a/Postfix Traversal/hw5.cpp b/Postfix Traversal/hw5.cpp
new file mode 100644
index 0000000..ebaba8f
--- /dev/null
+++ b/Postfix Traversal/hw5.cpp
@@ -0,0 +1,18 @@
+#include
+#include
+#include
+#include "post.h"
+
+int main(int argc, char *argv[]) {
+ char line[MAXLEN];
+ cout << "[B715152 Jinho Lee hw5 Postfix Result]" << '\n';
+ while (cin.getline(line, MAXLEN, '\n')) {
+ Expression e(line);
+ try {
+ Postfix(e);
+ }
+ catch (char const *msg) {
+ cerr << "Exception: " << msg << endl;
+ }
+ }
+}
diff --git a/Postfix Traversal/post.cpp b/Postfix Traversal/post.cpp
new file mode 100644
index 0000000..9402125
--- /dev/null
+++ b/Postfix Traversal/post.cpp
@@ -0,0 +1,186 @@
+#include
+#include
+#include "post.h"
+using namespace std;
+
+bool Token::operator==(char b) { return len == 1 && str[0] == b; }
+bool Token::operator!=(char b) { return len != 1 || str[0] != b; }
+Token::Token() {}
+
+Token::Token(char c) : len(1), type(c) { str = new char[1]; str[0] = c; } // default type = c itself
+Token::Token(char c, char c2, int type) : len(2), type(type) { str = new char[2]; str[0] = c; str[1] = c2; }
+Token::Token(char* arr, int l, int type = ID) : len(l), type(type){
+ str = new char[len + 1];
+ for (int i = 0; i < len; i++) str[i] = arr[i];
+ str[len] = '\0';
+
+ if (type == NUM) {
+ ival = arr[0] - '0';
+ for (int i = 1; i < len; i++) ival = ival * 10 + arr[i] - '0';
+ } else if (type == ID) ival = 0;
+ else throw "must be ID or NUM";
+}
+
+bool Token::IsOperand() { return type == ID || type == NUM; }
+
+
+ostream& operator<<(ostream& os, Token token) {
+ if (token.type == UMINUS) os << "-u";
+ else if (token.type == NUM) os << token.ival;
+ else for (int i = 0; i < token.len; i++) os << token.str[i];
+ os << " ";
+ return os;
+}
+
+bool GetID(Expression& expression, Token& token) {
+ char expString[MAXLEN]; int idLen = 0;
+ char c = expression.str[expression.pos];
+
+ if (!(c >= 'a' && c <= 'z' || c >= 'A' && c <= 'Z')) return false;
+ expString[idLen++] = c;
+ expression.pos++;
+ while ((c = expression.str[expression.pos])
+ >= 'a' && c <= 'z' || c >= 'A' && c <= 'Z' || c >= '0' && c <= '9') {
+ expString[idLen++] = c;
+ expression.pos++;
+ }
+ expString[idLen] = '\0';
+ token = Token(expString, idLen, ID); // return an ID
+ return true;
+}
+
+bool GetInt(Expression& expression, Token& token) {
+ char expIntString[MAXLEN]; int intLen = 0;
+ char c = expression.str[expression.pos];
+
+ if (!('0' <= c && c <= '9')) return false;
+ expIntString[intLen++] = c;
+ expression.pos++;
+ while ('0' <= (c = expression.str[expression.pos]) && c <= '9') {
+ expIntString[intLen++] = c;
+ expression.pos++;
+ }
+ expIntString[intLen] = '\0';
+ token = Token(expIntString, intLen, NUM);
+ return true;
+}
+
+
+void SkipBlanks(Expression& e) {
+ char c;
+ while (e.pos < e.len && ((c = e.str[e.pos]) == ' ' || c == '\t')){
+ e.pos++;
+ }
+}
+
+bool TwoCharOp(Expression& expression, Token& token) {
+ char c = expression.str[expression.pos];
+ char c2 = expression.str[expression.pos + 1];
+
+ int op = -1; // LE GE EQ NE AND OR UMINUS
+ if(c2 == '=') {
+ if (c == '<') op = LE;
+ else if (c == '>') op = GE;
+ else if (c == '=') op = EQ;
+ else if (c == '!') op = NE;
+ }
+ else if (c == '&' && c2 == '&') op = AND;
+ else if (c == '|' && c2 == '|') op = OR;
+ else if (c2 == '-') op = UMINUS;
+
+ if(op == -1) return false;
+
+ token = Token(c, c2, op); expression.pos += 2;
+ return true;
+}
+
+bool OneCharOp(Expression& e, Token& tok) {
+ char c = e.str[e.pos];
+ if (c == '-' || c == '!' || c == '*' || c == '/' || c == '%' ||
+ c == '+' || c == '<' || c == '>' || c == '(' || c == ')' || c == '=') {
+ tok = Token(c);
+ e.pos++;
+ return true;
+ }
+ return false;
+}
+
+
+Token NextToken(Expression& expression, bool INFIX = true) {
+ static bool oprrFound = true;
+ Token token;
+ SkipBlanks(expression); // skip blanks if any
+ if (expression.pos == expression.len) { // No more token left in this expression
+ if (INFIX) oprrFound = true;
+ return Token('#');
+ }
+
+ if (GetID(expression, token) || GetInt(expression, token)) {
+ if (INFIX) oprrFound = false;
+ return token;
+ }
+
+ if (TwoCharOp(expression, token) || OneCharOp(expression, token)) {
+ if (token.type == '-' && INFIX && oprrFound) {
+ token = Token('-', 'u', UMINUS);
+ }
+ if (INFIX) oprrFound = true;
+ return token;
+ }
+ throw "Illegal Character Found";
+}
+
+int icp(Token& t) { // in-coming priority
+ switch (t.type) {
+ case '(': return 0;
+ case '!': case UMINUS: return 1;
+ case '*': case '/': case '%': return 2;
+ case '+': case '-': return 3;
+ case '<': case '>': case LE: case GE: return 4;
+ case EQ: case NE: return 5;
+ case AND: return 6;
+ case OR: return 7;
+ case '=': return 8;
+ case '#': return 9;
+ }
+ return 0;
+}
+
+int isp(Token& t) { // in-stack priority
+ int type = t.type; //stack 에서의 우선순위 결정
+ switch (t.type) {
+ case '!': case UMINUS: return 1;
+ case '*': case '/': case '%': return 2;
+ case '+': case '-': return 3;
+ case '<': case '>': case LE: case GE: return 4;
+ case EQ: case NE: return 5;
+ case AND: return 6;
+ case OR: return 7;
+ case '=': return 8;
+ case '#': return 9;
+ case '(': return 10;
+ }
+ return 0;
+}
+
+void Postfix(Expression expression) {
+ stack st;
+ st.push(Token('#'));
+ Token tokenNow = NextToken(expression);
+ while(tokenNow.type != '#') {
+ if(tokenNow.type == NUM || tokenNow.type == ID) cout << tokenNow;
+ else if (tokenNow.type == '(') st.push(tokenNow);
+ else if (tokenNow.type == ')') {
+ while(st.top().type != '#' && st.top().type != '(') { cout << st.top(); st.pop(); }
+ st.pop();
+ }
+ else {
+ int priorityNow = icp(tokenNow);
+ while (st.top().type != '#' && isp(st.top()) <= priorityNow) { cout << st.top(); st.pop(); }
+ st.push(tokenNow);
+ }
+ tokenNow = NextToken(expression);
+ }
+ while (st.top().type != '#') { cout << st.top(); st.pop(); }
+ cout << '\n';
+}
diff --git a/Postfix Traversal/post.h b/Postfix Traversal/post.h
new file mode 100644
index 0000000..523788a
--- /dev/null
+++ b/Postfix Traversal/post.h
@@ -0,0 +1,68 @@
+
+#ifndef POSTFIX_H
+#define POSTFIX_H
+
+// token types for non one-char tokens
+#define ID 257
+
+#define NUM 258
+
+#define EQ 259
+
+#define NE 260
+
+#define GE 261
+
+#define LE 262
+
+#define AND 263
+
+#define OR 264
+
+
+#define UMINUS 265
+
+#define MAXLEN 80
+struct Expression {
+
+Expression(char* s) : str(s), pos(0)
+
+{ for (len = 0; str[len] != '\0'; len++); }
+char* str;
+
+int pos;
+int len;
+
+};
+
+struct Token {
+
+bool operator==(char);
+bool operator!=(char);
+
+Token();
+
+Token(char); // 1-char token: type equals the token itself
+Token(char, char, int); // 2-char token(e.g. <=) & its type(e.g.LE)
+
+Token(char*, int, int); //operand with its length & type(defaulted to ID)
+bool IsOperand(); // true if the token type is ID or NUM
+
+int type; // ascii code for 1-char op; predefined for other tokens
+char* str; // token value
+
+int len; // length of str
+
+int ival; // used to store an integer for type NUM; init to 0 for ID
+
+};
+
+using namespace std;
+
+ostream& operator<<(ostream&, Token t);
+
+Token NextToken(Expression&, bool); // 2nd arg = true for infix expression
+
+void Postfix(Expression expression);
+#endif
+
diff --git "a/Sorting Algorithm/\353\213\244\354\226\221\355\225\234 sorting algorithms cpp code.cpp" "b/Sorting Algorithm/\353\213\244\354\226\221\355\225\234 sorting algorithms cpp code.cpp"
new file mode 100644
index 0000000..d24c98a
--- /dev/null
+++ "b/Sorting Algorithm/\353\213\244\354\226\221\355\225\234 sorting algorithms cpp code.cpp"
@@ -0,0 +1,237 @@
+//
+// Created by Jinho on 2023-05-13.
+//
+
+#include
+#include
+#include
+#include
+#include
+#include
+#include
+
+
+#define MAX_ARRAY_NUMBER 100
+using namespace std;
+
+class SortingAlgorithm {
+public:
+ SortingAlgorithm(int _size) {
+ size = _size;
+ }
+
+ void selectionSort();
+ void insertionSort();
+ void bubbleSort();
+ void shellSort();
+ void mergeSort();
+ void quickSort();
+ void radixSort();
+
+ void printArray();
+
+private:
+ int size;
+ int *arr;
+ void generateRandomArray();
+ void mergeSort(int start, int end);
+ void quickSort(int start, int end);
+};
+
+void SortingAlgorithm::generateRandomArray() {
+ arr = new int[size];
+ for (int i = 0; i < size; i++) {
+ arr[i] = rand() % MAX_ARRAY_NUMBER;
+ }
+ printArray();
+}
+
+void SortingAlgorithm::selectionSort() {
+ cout << "============ selectionSort ============\n";
+ generateRandomArray();
+
+ int minNumber, minIdx;
+ for (int select = 0; select < size; select++) {
+ minNumber = MAX_ARRAY_NUMBER;
+ for (int i = select; i < size; i++) {
+ if (minNumber > arr[i]) {
+ minNumber = arr[i];
+ minIdx = i;
+ }
+ }
+ swap(arr[select], arr[minIdx]);
+ }
+
+ printArray();
+}
+
+void SortingAlgorithm::printArray() {
+ for (int i = 0; i < size; i++) {
+ cout << arr[i] << " ";
+ }
+ cout << "\n\n";
+}
+
+void SortingAlgorithm::insertionSort() {
+ cout << "============ insertionSort ============\n";
+ generateRandomArray();
+
+ int j, temp;
+ for (int i = 1; i < size; i++) {
+ temp = arr[i];
+ for (j = i - 1; j >= 0; j--) {
+ if (arr[j] > temp) {
+ arr[j + 1] = arr[j];
+ continue;
+ }
+ break;
+ }
+ arr[j + 1] = temp;
+ }
+
+ printArray();
+}
+
+void SortingAlgorithm::bubbleSort() {
+ cout << "============ bubbleSort ============\n";
+ generateRandomArray();
+
+ for (int i = 0; i < size; i++) {
+ for (int j = 0; j < size - 1; j++) {
+ if (arr[j] > arr[j + 1]) {
+ swap(arr[j], arr[j + 1]);
+ }
+ }
+ }
+
+ printArray();
+}
+
+void SortingAlgorithm::shellSort() {
+ cout << "============ shellSort ============\n";
+ generateRandomArray();
+
+ int gap;
+ for (gap = size / 2; gap > 0; gap /= 2) {
+ if (gap % 2 == 0) {
+ gap++;
+ }
+
+ for (int k = 0; k < gap; k++) {
+ int j, temp;
+ for (int i = k + gap; i < size; i += gap) {
+ temp = arr[i];
+ for (j = i - gap; j >= k && temp < arr[j]; j -= gap) {
+ arr[j + gap] = arr[j];
+ }
+ arr[j + gap] = temp;
+ }
+ }
+ }
+
+ printArray();
+}
+
+void SortingAlgorithm::mergeSort() {
+ cout << "============ mergeSort ============\n";
+ generateRandomArray();
+
+ mergeSort(0, size - 1);
+
+ printArray();
+}
+
+void SortingAlgorithm::mergeSort(int start, int end) {
+ if (start == end) return;
+
+ int mid = (start + end) / 2;
+ mergeSort(start, mid);
+ mergeSort(mid + 1, end);
+
+ int *tempArr = new int[size];
+ int i = start, j = mid + 1, k = start;
+ while (i <= mid && j <= end) {
+ if (arr[i] < arr[j]) {
+ tempArr[k++] = arr[i++];
+ } else {
+ tempArr[k++] = arr[j++];
+ }
+ }
+
+ while (i <= mid) {
+ tempArr[k++] = arr[i++];
+ }
+ while (j <= end) {
+ tempArr[k++] = arr[j++];
+ }
+
+ for (i = start; i <= end; i++) {
+ arr[i] = tempArr[i];
+ }
+}
+
+void SortingAlgorithm::quickSort() {
+ cout << "============ quickSort ============\n";
+ generateRandomArray();
+
+ quickSort(0, size - 1);
+ printArray();
+}
+
+void SortingAlgorithm::quickSort(int start, int end) {
+ if (start >= end) return;
+
+ int pivotValue = arr[start];
+ int pivot = start;
+
+ for (int j = start + 1; j <= end; j++) {
+ if (arr[j] < pivotValue) {
+ swap(arr[j], arr[++pivot]);
+ }
+ }
+ swap(arr[start], arr[pivot]);
+ quickSort(start, pivot - 1);
+ quickSort(pivot + 1, end);
+}
+
+void SortingAlgorithm::radixSort() {
+ cout << "============ radixSort ============\n";
+ generateRandomArray();
+
+ int temp = MAX_ARRAY_NUMBER, ten = 0;
+ while (temp > 0) {
+ temp /= 10;
+ ten++;
+ }
+ queue que[10];
+
+ // 자릿수
+ for (int idx = 0; idx < ten; idx++) {
+ for (int j = 0; j < size; j++) {
+ que[(arr[j] / (int) pow((int) 10, (int) idx)) % 10].push(arr[j]);
+ }
+ int newIdx = 0;
+ for (int j = 0; j < 10; j++) {
+ while (!que[j].empty()) {
+ arr[newIdx++] = que[j].front();
+ que[j].pop();
+ }
+ }
+ }
+
+ printArray();
+}
+
+
+int main() {
+ srand((unsigned int) (time(0)));
+
+ SortingAlgorithm sortingAlgorithm = SortingAlgorithm(10);
+ sortingAlgorithm.selectionSort();
+ sortingAlgorithm.insertionSort();
+ sortingAlgorithm.bubbleSort();
+ sortingAlgorithm.shellSort();
+ sortingAlgorithm.mergeSort();
+ sortingAlgorithm.quickSort();
+ sortingAlgorithm.radixSort();
+}
diff --git a/Subway Problem/hw10.cpp b/Subway Problem/hw10.cpp
new file mode 100644
index 0000000..2003635
--- /dev/null
+++ b/Subway Problem/hw10.cpp
@@ -0,0 +1,38 @@
+#include "subway.h"
+
+int main(int argc, char *argv[])
+{
+ argc = 3;
+ int numLine, line1, line2, cost;
+ string src, dst;
+
+ if(argc != 3) {
+ cerr << "Argument Count is " << argc << endl << "Argument must be " << argc << endl;
+ return 1;
+ }
+
+ fstream fin(argv[1]);
+ if(!fin) {
+ cerr << argv[1] << " open failed " << endl;
+ return 1;
+ }
+
+ fin >> numLine;
+ while (numLine--) {
+ fin >> line1 >> src >> line2 >> dst;
+ setStation(line1, src, line2, dst);
+ }
+ fin.close();
+
+ fstream fin2(argv[2]);
+ if(!fin2) {
+ cerr << argv[2] << " open failed" << endl;
+ return 1;
+ }
+ fin2 >> line1 >> src;
+ fin2 >> line2 >> dst;
+ fin2.close();
+
+ initDijkstra(line1, src, line2, dst);
+ return 0;
+}
diff --git a/Subway Problem/subway.cpp b/Subway Problem/subway.cpp
new file mode 100644
index 0000000..d844ed4
--- /dev/null
+++ b/Subway Problem/subway.cpp
@@ -0,0 +1,151 @@
+#include "subway.h"
+
+map stationToIdx;
+vector connected[110];
+int costTo[2][110];
+Station idxToStation[110];
+int stationCnt = 0;
+
+int getAbs(int num) {
+ return num < 0 ? num*(-1) : num;
+}
+
+string toString(int num) {
+ int ten = num / 10;
+ int left = num % 10;
+ string numStr;
+ numStr += (char) (ten + '0');
+ numStr += (char) (left + '0');
+ return numStr;
+}
+
+int getStationIdx(int line, string &src) {
+ if (stationToIdx.find(src) == stationToIdx.end() || stationToIdx[src].line[line] == -1) {
+ idxToStation[stationCnt].line = line;
+ idxToStation[stationCnt].station = src;
+ idxToStation[stationCnt].prevIdx = -1;
+ stationToIdx[src].line[line] = stationCnt++;
+ }
+
+ return stationToIdx[src].line[line];
+}
+
+void setStation(int line1, string &src, int line2, string &dst) {
+ int station1 = getStationIdx(line1, src);
+ int station2 = getStationIdx(line2, dst);
+ if (line1 != line2) return;
+ connected[station1].push_back(station2);
+ connected[station2].push_back(station1);
+}
+
+void Heap::push(Node *node) {
+ heap.push_back(node);
+ if (size() == 1) return;
+ temp = size();
+ while (temp != 1 && heap[temp / 2 - 1]->costNow < heap[temp - 1]->costNow) {
+ swap(heap[temp / 2 - 1], heap[temp - 1]);
+ temp /= 2;
+ }
+}
+
+void Heap::pop() {
+ if (empty()) return;
+ swap(heap.front(), heap.back());
+ heap.pop_back();
+ if (empty()) return;
+ temp = 1;
+ int child;
+
+ while (temp * 2 < size()) {
+ child = temp * 2;
+ if (temp * 2 + 1 < size() && heap[temp * 2]->costNow < heap[temp * 2 + 1]->costNow) child++;
+ if (heap[child] <= heap[temp]) break;
+ swap(heap[child], heap[temp]);
+ temp = child;
+ }
+}
+
+
+void traceStation(int dstIdx) {
+ stack st;
+ Station *stationNow;
+ while (dstIdx != -1) {
+ stationNow = &idxToStation[dstIdx];
+ if (st.empty() || st.top() != stationNow->station) st.push(stationNow->station);
+ dstIdx = stationNow->prevIdx;
+ }
+ while (!st.empty()) {
+ cerr << st.top() << endl;
+ st.pop();
+ }
+}
+
+void dijkstra(int sourceIdx, int destinationIdx, int step) {
+ Heap *heapTree = new Heap;
+ heapTree->push(new Node(sourceIdx, 0, -1));
+
+ int idxNow;
+ Station *stationNow;
+
+ while (!heapTree->empty()) {
+ Node *nodeNow = heapTree->top();
+ heapTree->pop();
+ idxNow = nodeNow->stationIdx;
+
+ if (costTo[step][idxNow] <= nodeNow->costNow) continue;
+ costTo[step][idxNow] = nodeNow->costNow;
+ stationNow = &idxToStation[idxNow];
+ stationNow->prevIdx = nodeNow->stationPrev;
+
+ if (idxNow == destinationIdx) continue;
+
+ // �ֱ�
+ for (int line = 1; line < 10; line++) {
+ if (stationToIdx[stationNow->station].line[line] == -1) continue;
+ if (line == stationNow->line) continue;
+ heapTree->push(new Node(stationToIdx[stationNow->station].line[line], nodeNow->costNow + 30, idxNow));
+ }
+ for (int i = 0; i < connected[idxNow].size(); i++) {
+ heapTree->push(new Node(connected[idxNow][i], nodeNow->costNow + 60, idxNow));
+ }
+ }
+}
+
+void printTimeNoNewline(int time) {
+ int second = time % 60;
+ cerr << time / 60 << ":" << toString(second) << endl;
+}
+
+void getMiddleStation() {
+ int ans = INF;
+ int cost[2];
+ string stationName;
+ for (int i = 0; i < stationCnt; i++) {
+ for (int j = 0; j < stationCnt; j++) {
+ if (idxToStation[i].station != idxToStation[j].station) continue;
+ if (ans < getAbs(costTo[0][i] - costTo[1][j])) continue;
+ ans = getAbs(costTo[0][i] - costTo[1][j]);
+ cost[0] = costTo[0][i];
+ cost[1] = costTo[1][j];
+ stationName = idxToStation[i].station;
+ }
+ }
+ cerr << stationName << " //middle point" << endl;
+
+ int first = cost[0] >= cost[1] ? 0 : 1;
+ printTimeNoNewline(cost[first]);
+ printTimeNoNewline(cost[1 - first]);
+}
+
+void initDijkstra(int line1, string &src, int line2, string &dst) {
+ for (int i = 0; i < 2; i++) for (int j = 0; j < 110; j++) costTo[i][j] = INF;
+
+ int sourceIdx = stationToIdx[src].line[line1];
+ int destinationIdx = stationToIdx[dst].line[line2];
+ dijkstra(sourceIdx, destinationIdx, 0);
+ traceStation(destinationIdx);
+ printTimeNoNewline(costTo[0][destinationIdx]);
+
+ dijkstra(destinationIdx, sourceIdx, 1);
+ getMiddleStation();
+}
diff --git a/Subway Problem/subway.h b/Subway Problem/subway.h
new file mode 100644
index 0000000..f961588
--- /dev/null
+++ b/Subway Problem/subway.h
@@ -0,0 +1,53 @@
+//
+// Created by Jinho on 11/18/2022.
+//
+
+#ifndef SUBWAY_H
+#define SUBWAY_H
+#define INF 98765432
+
+#include
+#include
+#include
+#include