|
| 1 | +Holder |
| 2 | +====== |
| 3 | + |
| 4 | + |
| 5 | + |
| 6 | +Holder uses SVG and `canvas` to render image placeholders on the client side. |
| 7 | + |
| 8 | +[Bootstrap](http://getbootstrap.com) uses Holder in documentation. |
| 9 | + |
| 10 | +You can install Holder using [Bower](http://bower.io/): `bower install holderjs` |
| 11 | + |
| 12 | +How to use it |
| 13 | +------------- |
| 14 | + |
| 15 | +Include ``holder.js`` in your HTML: |
| 16 | + |
| 17 | +```html |
| 18 | +<script src="holder.js"></script> |
| 19 | +``` |
| 20 | + |
| 21 | +Holder will then process all images with a specific ``src`` attribute, like this one: |
| 22 | + |
| 23 | +```html |
| 24 | +<img src="holder.js/200x300"> |
| 25 | +``` |
| 26 | + |
| 27 | +The above tag will render as a placeholder 200 pixels wide and 300 pixels tall. |
| 28 | + |
| 29 | +To avoid console 404 errors, you can use ``data-src`` instead of ``src``. |
| 30 | + |
| 31 | +Theming |
| 32 | +------- |
| 33 | + |
| 34 | +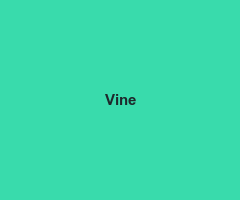 |
| 35 | + |
| 36 | +Holder includes support for themes, to help placeholders blend in with your layout. |
| 37 | + |
| 38 | +There are 6 default themes: ``sky``, ``vine``, ``lava``, ``gray``, ``industrial``, and ``social``. Use them like so: |
| 39 | + |
| 40 | +```html |
| 41 | +<img src="holder.js/200x300/sky"> |
| 42 | +``` |
| 43 | + |
| 44 | +Customizing themes |
| 45 | +------------------ |
| 46 | + |
| 47 | +Themes have 4 properties: ``foreground``, ``background``, ``size``, and ``font``. The ``size`` property specifies the minimum font size for the theme. You can create a sample theme like this: |
| 48 | + |
| 49 | +```js |
| 50 | +Holder.add_theme("dark", {background:"#000", foreground:"#aaa", size:11, font: "Monaco"}) |
| 51 | +``` |
| 52 | + |
| 53 | +Using custom themes |
| 54 | +------------------- |
| 55 | + |
| 56 | +There are two ways to use custom themes with Holder: |
| 57 | + |
| 58 | +* Include theme at runtime to render placeholders already using the theme name |
| 59 | +* Include theme at any point and re-render placeholders that are using the theme name |
| 60 | + |
| 61 | +The first approach is the easiest. After you include ``holder.js``, add a ``script`` tag that adds the theme you want: |
| 62 | + |
| 63 | +```html |
| 64 | +<script src="holder.js"></script> |
| 65 | +<script> Holder.add_theme("bright", { background: "white", foreground: "gray", size: 12 })</script> |
| 66 | +``` |
| 67 | + |
| 68 | +The second approach requires that you call ``run`` after you add the theme, like this: |
| 69 | + |
| 70 | +```js |
| 71 | +Holder.add_theme("bright", { background: "white", foreground: "gray", size: 12}).run() |
| 72 | +``` |
| 73 | + |
| 74 | +Using custom themes and domain on specific images |
| 75 | +------------------------------------------------- |
| 76 | + |
| 77 | +You can use Holder in different areas on different images with custom themes: |
| 78 | + |
| 79 | +```html |
| 80 | +<img data-src="example.com/100x100/simple" id="new"> |
| 81 | +``` |
| 82 | + |
| 83 | +```js |
| 84 | +Holder.run({ |
| 85 | + domain: "example.com", |
| 86 | + themes: { |
| 87 | + "simple":{ |
| 88 | + background:"#fff", |
| 89 | + foreground:"#000", |
| 90 | + size:12 |
| 91 | + } |
| 92 | + }, |
| 93 | + images: "#new" |
| 94 | + }) |
| 95 | +``` |
| 96 | + |
| 97 | +Using custom colors on specific images |
| 98 | +-------------------------------------- |
| 99 | + |
| 100 | +Custom colors on a specific image can be specified in the ``background:foreground`` format using hex notation, like this: |
| 101 | + |
| 102 | +```html |
| 103 | +<img data-src="holder.js/100x200/#000:#fff"> |
| 104 | +``` |
| 105 | + |
| 106 | +The above will render a placeholder with a black background and white text. |
| 107 | + |
| 108 | +Custom text |
| 109 | +----------- |
| 110 | + |
| 111 | +You can specify custom text using the ``text:`` operator: |
| 112 | + |
| 113 | +```html |
| 114 | +<img data-src="holder.js/200x200/text:hello world"> |
| 115 | +``` |
| 116 | + |
| 117 | +If you have a group of placeholders where you'd like to use particular text, you can do so by adding a ``text`` property to the theme: |
| 118 | + |
| 119 | +```js |
| 120 | +Holder.add_theme("thumbnail", { background: "#fff", text: "Thumbnail" }) |
| 121 | +``` |
| 122 | + |
| 123 | +Fluid placeholders |
| 124 | +------------------ |
| 125 | + |
| 126 | +Specifying a dimension in percentages creates a fluid placeholder that responds to media queries. |
| 127 | + |
| 128 | +```html |
| 129 | +<img data-src="holder.js/100%x75/social"> |
| 130 | +``` |
| 131 | + |
| 132 | +By default, the fluid placeholder will show its current size in pixels. To display the original dimensions, i.e. 100%x75, set the ``textmode`` flag to ``literal`` like so: `holder.js/100%x75/textmode:literal`. |
| 133 | + |
| 134 | +Automatically sized placeholders |
| 135 | +-------------------------------- |
| 136 | + |
| 137 | +If you'd like to avoid Holder enforcing an image size, use the ``auto`` flag like so: |
| 138 | + |
| 139 | +```html |
| 140 | +<img data-src="holder.js/200x200/auto"> |
| 141 | +``` |
| 142 | + |
| 143 | +The above will render a placeholder without any embedded CSS for height or width. |
| 144 | + |
| 145 | +To show the current size of an automatically sized placeholder, set the ``textmode`` flag to ``exact`` like so: `holder.js/200x200/auto/textmode:exact`. |
| 146 | + |
| 147 | +Background placeholders |
| 148 | +----------------------- |
| 149 | + |
| 150 | +Holder can render placeholders as background images for elements with the `holderjs` class, like this: |
| 151 | + |
| 152 | +```css |
| 153 | +#sample {background:url(?holder.js/200x200/social) no-repeat} |
| 154 | +``` |
| 155 | + |
| 156 | +```html |
| 157 | +<div id="sample" class="holderjs"></div> |
| 158 | +``` |
| 159 | + |
| 160 | +The Holder URL in CSS should have a `?` in front. You can change the default class by specifying a selector as the `bgnodes` property when calling `Holder.run`. |
| 161 | + |
| 162 | +Customizing only the settings you need |
| 163 | +-------------------------------------- |
| 164 | + |
| 165 | +Holder extends its default settings with the settings you provide, so you only have to include those settings you want changed. For example, you can run Holder on a specific domain like this: |
| 166 | + |
| 167 | +```js |
| 168 | +Holder.run({domain:"example.com"}) |
| 169 | +``` |
| 170 | + |
| 171 | +Using custom settings on load |
| 172 | +----------------------------- |
| 173 | + |
| 174 | +You can prevent Holder from running its default configuration by executing ``Holder.run`` with your custom settings right after including ``holder.js``. However, you'll have to execute ``Holder.run`` again to render any placeholders that use the default configuration. |
| 175 | + |
| 176 | +Inserting an image with optional custom theme |
| 177 | +--------------------------------------------- |
| 178 | + |
| 179 | +You can add a placeholder programmatically by chaining Holder calls: |
| 180 | + |
| 181 | +```js |
| 182 | +Holder.add_theme("new",{foreground:"#ccc", background:"#000", size:10}).add_image("holder.js/200x100/new", "body").run() |
| 183 | +``` |
| 184 | + |
| 185 | +The first argument in ``add_image`` is the ``src`` attribute, and the second is a CSS selector of the parent element. |
| 186 | + |
| 187 | +Changing rendering engine |
| 188 | +------------------------- |
| 189 | + |
| 190 | +By default, Holder renders placeholders using SVG, however it has a fallback `canvas` engine that it uses in case the browser lacks SVG support. If you'd like to force `canvas` rendering, you can do it like so: |
| 191 | + |
| 192 | +```js |
| 193 | +Holder.run({use_canvas:true}) |
| 194 | +``` |
| 195 | + |
| 196 | +Using with ``lazyload.js`` |
| 197 | +-------------------------- |
| 198 | + |
| 199 | +Holder is compatible with ``lazyload.js`` and works with both fluid and fixed-width images. For best results, run `.lazyload({skip_invisible:false})`. |
| 200 | + |
| 201 | +Browser support |
| 202 | +--------------- |
| 203 | + |
| 204 | +* Chrome |
| 205 | +* Firefox 3+ |
| 206 | +* Safari 4+ |
| 207 | +* Internet Explorer 6+ (with fallback for older IE) |
| 208 | +* Android (with fallback) |
| 209 | + |
| 210 | +License |
| 211 | +------- |
| 212 | + |
| 213 | +Holder is provided under the [MIT License](http://opensource.org/licenses/MIT). |
| 214 | + |
| 215 | +Credits |
| 216 | +------- |
| 217 | + |
| 218 | +Holder is a project by [Ivan Malopinsky](http://imsky.co). |
0 commit comments