Commit 38c08de
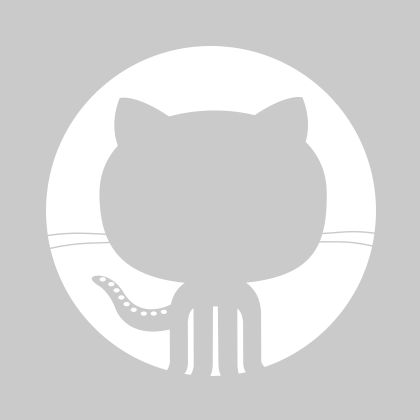
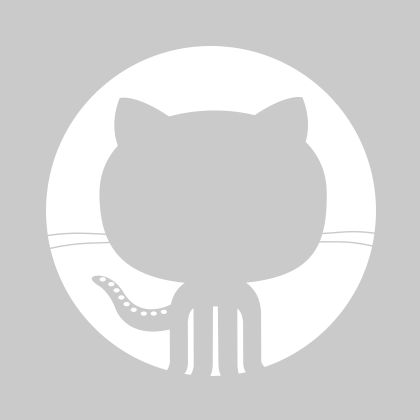
Doug Powers
Doug Powers
1 parent b330190 commit 38c08de
File tree
26 files changed
+126
-57
lines changed26 files changed
+126
-57
lines changedARPHandler.cpp
100755
100644
+1
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
72 | 72 |
| |
73 | 73 |
| |
74 | 74 |
| |
| 75 | + | |
75 | 76 |
| |
76 | 77 |
| |
77 | 78 |
| |
|
+1-1
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
2 | 2 |
| |
3 | 3 |
| |
4 | 4 |
| |
5 |
| - | |
| 5 | + | |
6 | 6 |
| |
7 | 7 |
| |
8 | 8 |
| |
|
+2-2
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
8 | 8 |
| |
9 | 9 |
| |
10 | 10 |
| |
11 |
| - | |
| 11 | + | |
12 | 12 |
| |
13 | 13 |
| |
14 | 14 |
| |
| |||
26 | 26 |
| |
27 | 27 |
| |
28 | 28 |
| |
29 |
| - | |
| 29 | + | |
30 | 30 |
| |
31 | 31 |
| |
32 | 32 |
| |
|
DNSHandler.cpp
100755
100644
+3-3
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
59 | 59 |
| |
60 | 60 |
| |
61 | 61 |
| |
62 |
| - | |
| 62 | + | |
63 | 63 |
| |
64 | 64 |
| |
65 | 65 |
| |
| |||
101 | 101 |
| |
102 | 102 |
| |
103 | 103 |
| |
104 |
| - | |
| 104 | + | |
105 | 105 |
| |
106 | 106 |
| |
107 | 107 |
| |
| |||
155 | 155 |
| |
156 | 156 |
| |
157 | 157 |
| |
158 |
| - | |
| 158 | + | |
159 | 159 |
| |
160 | 160 |
| |
161 | 161 |
| |
|
+2-2
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
37 | 37 |
| |
38 | 38 |
| |
39 | 39 |
| |
40 |
| - | |
| 40 | + | |
41 | 41 |
| |
42 | 42 |
| |
43 | 43 |
| |
44 | 44 |
| |
45 | 45 |
| |
46 |
| - | |
| 46 | + | |
47 | 47 |
| |
48 | 48 |
| |
49 | 49 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
29 | 29 |
| |
30 | 30 |
| |
31 | 31 |
| |
32 |
| - | |
| 32 | + | |
33 | 33 |
| |
34 | 34 |
| |
35 | 35 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
25 | 25 |
| |
26 | 26 |
| |
27 | 27 |
| |
28 |
| - | |
| 28 | + | |
29 | 29 |
| |
30 | 30 |
| |
31 | 31 |
| |
|
ENC28J60Driver.cpp
100755
100644
File mode changed.
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
37 | 37 |
| |
38 | 38 |
| |
39 | 39 |
| |
40 |
| - | |
| 40 | + | |
41 | 41 |
| |
42 | 42 |
| |
43 | 43 |
| |
|
ENC28J60Registers.h
100755
100644
File mode changed.
EtherControl.cpp
100755
100644
+6-2
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
3 | 3 |
| |
4 | 4 |
| |
5 | 5 |
| |
| 6 | + | |
| 7 | + | |
| 8 | + | |
| 9 | + | |
| 10 | + | |
| 11 | + | |
6 | 12 |
| |
7 | 13 |
| |
8 | 14 |
| |
| |||
79 | 85 |
| |
80 | 86 |
| |
81 | 87 |
| |
82 |
| - | |
83 | 88 |
| |
84 | 89 |
| |
85 | 90 |
| |
| |||
209 | 214 |
| |
210 | 215 |
| |
211 | 216 |
| |
212 |
| - | |
213 | 217 |
| |
214 | 218 |
| |
215 | 219 |
| |
|
EtherControl.h
100755
100644
File mode changed.
EthernetDriver.h
100755
100644
File mode changed.
+80-22
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
2 | 2 |
| |
3 | 3 |
| |
4 | 4 |
| |
5 |
| - | |
| 5 | + | |
6 | 6 |
| |
7 |
| - | |
| 7 | + | |
8 | 8 |
| |
9 | 9 |
| |
10 |
| - | |
11 |
| - | |
| 10 | + | |
12 | 11 |
| |
13 |
| - | |
| 12 | + | |
14 | 13 |
| |
15 | 14 |
| |
16 | 15 |
| |
| 16 | + | |
| 17 | + | |
| 18 | + | |
| 19 | + | |
| 20 | + | |
| 21 | + | |
| 22 | + | |
| 23 | + | |
| 24 | + | |
| 25 | + | |
| 26 | + | |
| 27 | + | |
| 28 | + | |
| 29 | + | |
| 30 | + | |
| 31 | + | |
| 32 | + | |
| 33 | + | |
| 34 | + | |
| 35 | + | |
| 36 | + | |
| 37 | + | |
| 38 | + | |
| 39 | + | |
| 40 | + | |
17 | 41 |
| |
18 |
| - | |
19 |
| - | |
20 | 42 |
| |
21 |
| - | |
22 |
| - | |
23 |
| - | |
24 |
| - | |
| 43 | + | |
| 44 | + | |
| 45 | + | |
| 46 | + | |
| 47 | + | |
| 48 | + | |
| 49 | + | |
| 50 | + | |
| 51 | + | |
| 52 | + | |
| 53 | + | |
| 54 | + | |
| 55 | + | |
| 56 | + | |
| 57 | + | |
| 58 | + | |
| 59 | + | |
| 60 | + | |
| 61 | + | |
| 62 | + | |
| 63 | + | |
| 64 | + | |
| 65 | + | |
| 66 | + | |
| 67 | + | |
| 68 | + | |
25 | 69 |
| |
26 | 70 |
| |
27 | 71 |
| |
28 |
| - | |
29 |
| - | |
30 |
| - | |
31 |
| - | |
32 |
| - | |
| 72 | + | |
| 73 | + | |
| 74 | + | |
| 75 | + | |
| 76 | + | |
| 77 | + | |
| 78 | + | |
| 79 | + | |
| 80 | + | |
| 81 | + | |
| 82 | + | |
33 | 83 |
| |
34 | 84 |
| |
35 | 85 |
| |
36 | 86 |
| |
37 | 87 |
| |
38 |
| - | |
| 88 | + | |
| 89 | + | |
39 | 90 |
| |
40 | 91 |
| |
41 | 92 |
| |
42 | 93 |
| |
43 |
| - | |
| 94 | + | |
| 95 | + | |
| 96 | + | |
| 97 | + | |
| 98 | + | |
| 99 | + | |
| 100 | + | |
| 101 | + | |
| 102 | + | |
| 103 | + | |
44 | 104 |
| |
45 | 105 |
| |
46 | 106 |
| |
47 |
| - | |
48 |
| - | |
49 |
| - | |
50 |
| - | |
| 107 | + | |
| 108 | + | |
51 | 109 |
|
+10-6
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
5 | 5 |
| |
6 | 6 |
| |
7 | 7 |
| |
8 |
| - | |
| 8 | + | |
| 9 | + | |
| 10 | + | |
| 11 | + | |
9 | 12 |
| |
10 |
| - | |
| 13 | + | |
11 | 14 |
| |
12 | 15 |
| |
13 | 16 |
| |
| |||
17 | 20 |
| |
18 | 21 |
| |
19 | 22 |
| |
20 |
| - | |
21 |
| - | |
22 |
| - | |
23 |
| - | |
| 23 | + | |
| 24 | + | |
| 25 | + | |
| 26 | + | |
| 27 | + | |
24 | 28 |
| |
25 | 29 |
| |
26 | 30 |
|
IPHandler.cpp
100755
100644
+1-1
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
126 | 126 |
| |
127 | 127 |
| |
128 | 128 |
| |
129 |
| - | |
| 129 | + | |
130 | 130 |
| |
131 | 131 |
| |
132 | 132 |
| |
|
+1-1
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
27 | 27 |
| |
28 | 28 |
| |
29 | 29 |
| |
30 |
| - | |
| 30 | + | |
31 | 31 |
| |
32 | 32 |
| |
33 | 33 |
| |
|
+1-1
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
16 | 16 |
| |
17 | 17 |
| |
18 | 18 |
| |
19 |
| - | |
| 19 | + | |
20 | 20 |
| |
21 | 21 |
| |
22 | 22 |
| |
|
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
56 | 56 |
| |
57 | 57 |
| |
58 | 58 |
| |
59 |
| - | |
| 59 | + | |
60 | 60 |
| |
61 | 61 |
| |
62 | 62 |
| |
|
+1-1
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
18 | 18 |
| |
19 | 19 |
| |
20 | 20 |
| |
21 |
| - | |
| 21 | + | |
22 | 22 |
| |
23 | 23 |
| |
24 | 24 |
| |
|
+4-2
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
49 | 49 |
| |
50 | 50 |
| |
51 | 51 |
| |
| 52 | + | |
| 53 | + | |
52 | 54 |
| |
53 | 55 |
| |
54 | 56 |
| |
| |||
111 | 113 |
| |
112 | 114 |
| |
113 | 115 |
| |
114 |
| - | |
| 116 | + | |
115 | 117 |
| |
116 | 118 |
| |
117 | 119 |
| |
118 | 120 |
| |
119 | 121 |
| |
120 | 122 |
| |
121 |
| - | |
| 123 | + | |
122 | 124 |
| |
123 | 125 |
| |
124 | 126 |
| |
|
+1-1
Original file line number | Diff line number | Diff line change | |
---|---|---|---|
| |||
23 | 23 |
| |
24 | 24 |
| |
25 | 25 |
| |
26 |
| - | |
| 26 | + | |
27 | 27 |
| |
28 | 28 |
| |
29 | 29 |
| |
|
0 commit comments