You signed in with another tab or window. Reload to refresh your session.You signed out in another tab or window. Reload to refresh your session.You switched accounts on another tab or window. Reload to refresh your session.Dismiss alert
Copy file name to clipboardexpand all lines: README.md
+42-40
Original file line number
Diff line number
Diff line change
@@ -1,9 +1,21 @@
1
-
HTML5 Drag and Drop for Dart
2
-
================
1
+
# HTML5 Drag and Drop for Dart
2
+
3
+
***
4
+
5
+
### Note: This library is not maintained any more.
6
+
7
+
* Use the [Dart drag and drop](http://code.makery.ch/library/dart-drag-and-drop/) library instead (better, without HTML5).
8
+
* Read the details about why I chose [Drag and Drop without HTML5](http://code.makery.ch/blog/drag-and-drop-without-html5/)
9
+
10
+
***
11
+
12
+
13
+
3
14
4
15
Helper library to simplify **HTML5 Drag and Drop** in Dart.
5
16
6
-
## Features ##
17
+
## Features
18
+
7
19
* Make any HTML Element `draggable`.
8
20
* Create `dropzones` and connect them with `draggables`.
9
21
* Rearrange elements with `sortables` (similar to jQuery UI Sortable).
@@ -14,33 +26,9 @@ Helper library to simplify **HTML5 Drag and Drop** in Dart.
14
26
This is the case for IE9 and partly for IE10 (when custom drag images are
15
27
used).
16
28
17
-
## Demo ##
18
-
See [HTML5 Drag and Drop in action](http://edu.makery.ch/projects/dart-html5-drag-and-drop)
19
-
(with code examples).
20
29
21
-
[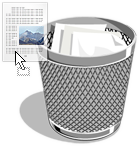](http://edu.makery.ch/projects/dart-html5-drag-and-drop)
30
+
## Usage
22
31
23
-
All examples are also available in the `example` directory on GitHub.
24
-
25
-
## Installation ##
26
-
27
-
### Add Dependency ###
28
-
Add the folowing to your **pubspec.yaml** and run **pub install**
29
-
```yaml
30
-
dependencies:
31
-
html5_dnd: any
32
-
```
33
-
34
-
### Import ###
35
-
Import the `html5_dnd` library in your Dart code.
36
-
37
-
```dart
38
-
import 'package:html5_dnd/html5_dnd.dart';
39
-
40
-
// ...
41
-
```
42
-
43
-
## Usage ##
44
32
See the demo page above or the `example` directory to see some live examples
45
33
with code.
46
34
@@ -54,14 +42,16 @@ elements in a `SortableGroup`. The `SortableGroup` will make the installed
54
42
elements both into draggables and dropzones and thus creates sortable behaviour.
55
43
56
44
57
-
### Disable Touch Support ###
45
+
### Disable Touch Support
46
+
58
47
There is a global property called `enableTouchEvents` which is `true` by
59
48
default. This means that touch events are automatically enabled on devices that
60
49
support it. If touch support should not be used even on touch devices, set this
61
50
flag to `false`.
62
51
63
52
64
-
### Draggables ###
53
+
### Draggables
54
+
65
55
Any HTML element can be made draggable. First we'll have to create a
66
56
`DraggableGroup` that manages draggable elements. The `DraggableGroup` holds
67
57
all options for dragging and provides event streams we can listen to.
0 commit comments