diff --git a/docs/source/conf.py b/docs/source/conf.py
index 770fdee6..fba76894 100644
--- a/docs/source/conf.py
+++ b/docs/source/conf.py
@@ -36,7 +36,7 @@
author = 'Marco Musy'
# The short X.Y version
-version = '2019.1.1'
+version = '2019.1.2'
# -- General configuration ---------------------------------------------------
diff --git a/examples/advanced/fitplanes.py b/examples/advanced/fitplanes.py
index 02474624..40f0977b 100644
--- a/examples/advanced/fitplanes.py
+++ b/examples/advanced/fitplanes.py
@@ -9,7 +9,7 @@
"""
from vtkplotter import *
-vp = Plotter(verbose=0, axes=0)
+vp = Plotter(verbose=0, axes=0, bg='w')
s = vp.load(datadir+"shapes/cow.vtk").alpha(0.3).subdivide() # remesh
diff --git a/examples/basic/annotations.py b/examples/basic/annotations.py
index ffd7f6cd..ed1d8523 100644
--- a/examples/basic/annotations.py
+++ b/examples/basic/annotations.py
@@ -7,6 +7,6 @@
with open("basic/align1.py") as fname:
t = fname.read()
-actor2d = Text(t, pos=3, s=1.2, bg="lb", font="courier")
+actor2d = Text(t, pos=3, s=1.2, c='k', bg="lb", font="courier")
show(actor2d, verbose=0, axes=0)
diff --git a/examples/basic/carcrash.py b/examples/basic/carcrash.py
index 66dad529..47a1216d 100644
--- a/examples/basic/carcrash.py
+++ b/examples/basic/carcrash.py
@@ -4,7 +4,7 @@
rate=10 limits the speed of the loop to maximum 10 fps
"""
from __future__ import division, print_function
-from vtkplotter import Plotter, Plane, Text
+from vtkplotter import Plotter, Plane, Text, datadir
vp = Plotter(interactive=0, axes=0)
diff --git a/examples/basic/rotateImage.py b/examples/basic/rotateImage.py
index 4d093510..60de2be7 100644
--- a/examples/basic/rotateImage.py
+++ b/examples/basic/rotateImage.py
@@ -2,7 +2,7 @@
Normal jpg/png images can be
loaded and rendered as any vtkImageActor
"""
-from vtkplotter import Plotter, Text
+from vtkplotter import Plotter, Text, datadir
vp = Plotter(axes=3, verbose=0)
diff --git a/examples/basic/texturecubes.py b/examples/basic/texturecubes.py
index 8804cb13..3adcd985 100644
--- a/examples/basic/texturecubes.py
+++ b/examples/basic/texturecubes.py
@@ -3,7 +3,7 @@
any jpg file can be used as texture.
"""
from vtkplotter import Plotter, Cube, Text
-from vtkplotter.utils import textures, textures_path
+from vtkplotter.settings import textures, textures_path
print(__doc__)
print(textures_path)
diff --git a/examples/basic/trail.py b/examples/basic/trail.py
index b780e730..1fe9ae65 100644
--- a/examples/basic/trail.py
+++ b/examples/basic/trail.py
@@ -1,5 +1,6 @@
"""
-Example usage of addTrail(). Add a triling line to a moving object.
+Example usage of addTrail().
+Add a trailing line to a moving object.
"""
print(__doc__)
from vtkplotter import Plotter, sin, Sphere, Point
diff --git a/examples/other/dolfin/README.md b/examples/other/dolfin/README.md
index 06031d91..5a297c34 100644
--- a/examples/other/dolfin/README.md
+++ b/examples/other/dolfin/README.md
@@ -32,3 +32,7 @@ python example.py # on mac OSX try 'pythonw' instead
| [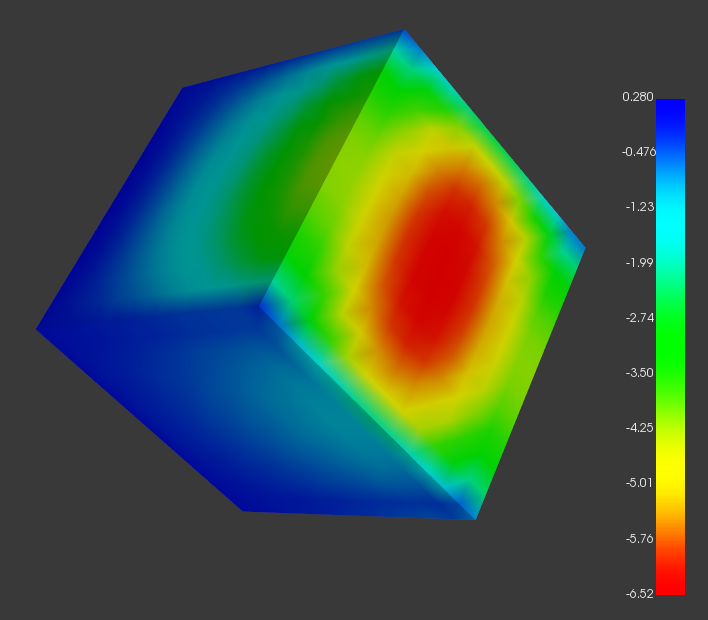](https://github.com/marcomusy/vtkplotter/blob/master/examples/other/dolfin/ex07_stokes-iterative.py)
`ex07_stokes-iterative.py` | Stokes equations with an iterative solver. |
| | |
| [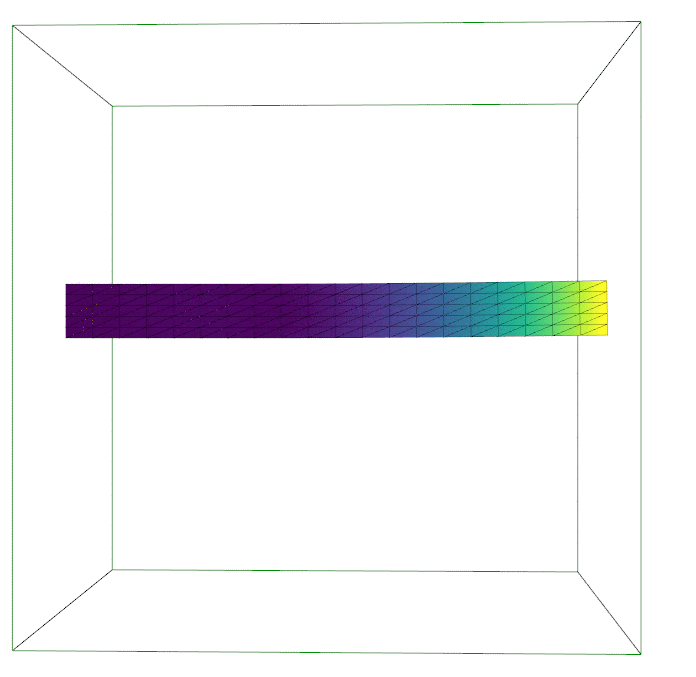](https://github.com/marcomusy/vtkplotter/blob/master/examples/other/dolfin/elastodynamics.py)
`elastodynamics.py` | Perform time integration of transient elastodynamics using the generalized-alpha method. |
+| | |
+| [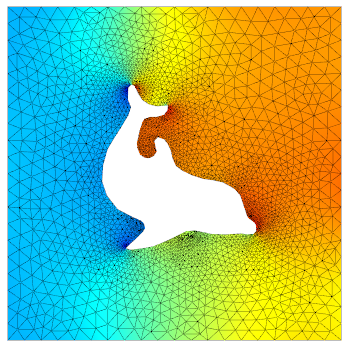](https://github.com/marcomusy/vtkplotter/blob/master/examples/other/dolfin/stokes.py)
`stokes.py` | Solve 2D navier-stokes equations with boundary conditions. |
+
+
diff --git a/examples/other/dolfin/ascalarbar.py b/examples/other/dolfin/ascalarbar.py
index 335934d5..e21469f8 100644
--- a/examples/other/dolfin/ascalarbar.py
+++ b/examples/other/dolfin/ascalarbar.py
@@ -19,11 +19,5 @@
################################## vtkplotter
from vtkplotter.dolfin import plot
-plot(u, mode='color', vmin=-6, vmax=6, style=1, text=__doc__)
+plot(u, mode='color', vmin=-3, vmax=3, style=1, text=__doc__)
-
-################################# pylab
-#import pylab as plt
-#c = plot(u, mode='color', vmin=-3, vmax=3)
-#plt.colorbar(c)
-#plt.show()
\ No newline at end of file
diff --git a/examples/other/dolfin/calc_surface_area.py b/examples/other/dolfin/calc_surface_area.py
new file mode 100644
index 00000000..8c6e2087
--- /dev/null
+++ b/examples/other/dolfin/calc_surface_area.py
@@ -0,0 +1,43 @@
+from dolfin import *
+import sympy as sp
+# Credits:
+# https://github.com/pf4d/fenics_scripts/calc_surface_area.py
+
+x, y = sp.symbols('x, y')
+
+# surface :
+def s(x,y): return sp.exp(x)
+
+# x-derivative of surface
+def dsdx(x,y): return s(x,y).diff(x, 1)
+
+# y-derivative of surface
+def dsdy(x,y): return s(x,y).diff(y, 1)
+
+# outward-pointing-normal-vector magnitude at surface :
+def n_mag_s(x,y): return sp.sqrt(1 + dsdx(x,y)**2 + dsdy(x,y)**2)
+
+# surface area of surface :
+def area(x,y): return sp.integrate(n_mag_s(x,y), (x,0,1), (y,0,1))
+
+A_exact = area(x,y)
+
+for n in [5,10,100,500]:
+ mesh = UnitSquareMesh(n,n)
+ Q = FunctionSpace(mesh, "CG", 1)
+ e = Expression('exp(x[0])', degree=2)
+ f = interpolate(e, Q)
+ A_num = assemble( sqrt(f.dx(0)**2 + f.dx(1)**2 + 1) * dx)
+ print('for n = %i -- error = %.2e' % (n, abs(A_exact.evalf()-A_num)))
+
+n = 10
+mesh = UnitSquareMesh(n,n)
+Q = FunctionSpace(mesh, "CG", 1)
+e = Expression('exp(x[0])', degree=2)
+f = interpolate(e, Q)
+A_vector = project( sqrt(f.dx(0)**2 + f.dx(1)**2 + 1), Q)
+
+
+from vtkplotter.dolfin import plot
+plot(A_vector)
+
diff --git a/examples/other/dolfin/elastodynamics.py b/examples/other/dolfin/elastodynamics.py
index d01468a8..fec96d73 100644
--- a/examples/other/dolfin/elastodynamics.py
+++ b/examples/other/dolfin/elastodynamics.py
@@ -2,6 +2,7 @@
Time-integration of the
elastodynamics equation
'''
+from __future__ import division, print_function
from dolfin import *
import numpy as np
diff --git a/examples/other/dolfin/ex04_mixed-poisson.py b/examples/other/dolfin/ex04_mixed-poisson.py
index 6bcd37e5..2756961b 100644
--- a/examples/other/dolfin/ex04_mixed-poisson.py
+++ b/examples/other/dolfin/ex04_mixed-poisson.py
@@ -2,6 +2,7 @@
Solving Poisson equation using
a mixed (two-field) formulation.
"""
+# needs python3
# https://fenicsproject.org/docs/dolfin/2018.1.0/python/demos/mixed-poisson
from dolfin import *
diff --git a/examples/other/dolfin/pi_estimate.py b/examples/other/dolfin/pi_estimate.py
new file mode 100644
index 00000000..b14523d4
--- /dev/null
+++ b/examples/other/dolfin/pi_estimate.py
@@ -0,0 +1,15 @@
+from dolfin import *
+from mshr import Circle, generate_mesh
+from vtkplotter.dolfin import plot, printc
+# Credits:
+# https://github.com/pf4d/fenics_scripts/pi_estimate.py
+
+domain = Circle(Point(0.0,0.0), 1.0)
+
+for res in [2**k for k in range(8)]:
+ mesh = generate_mesh(domain, res)
+ A = assemble(Constant(1) * dx(domain=mesh))
+ printc("resolution = %i, \t |A - pi| = %.5e" % (res, abs(A-pi)))
+printc('~pi is about', A, c='yellow')
+
+plot(mesh, style=1, axes=3)
diff --git a/examples/other/dolfin/run_all.sh b/examples/other/dolfin/run_all.sh
index 253f7bde..c701ed55 100755
--- a/examples/other/dolfin/run_all.sh
+++ b/examples/other/dolfin/run_all.sh
@@ -37,12 +37,20 @@ python ascalarbar.py
echo Running collisions.py
python collisions.py
+echo Running calc_surface_area.py
+python calc_surface_area.py
+
echo Running markmesh.py
python markmesh.py
echo Running elastodynamics.py
python elastodynamics.py
+echo Running pi_estimate.py
+python pi_estimate.py
+
+echo Running stokes.py
+python stokes.py
diff --git a/examples/other/dolfin/stokes.py b/examples/other/dolfin/stokes.py
new file mode 100644
index 00000000..5f3d3cbc
--- /dev/null
+++ b/examples/other/dolfin/stokes.py
@@ -0,0 +1,52 @@
+"""
+This demo solves the Stokes equations, using quadratic elements for
+the velocity and first degree elements for the pressure (Taylor-Hood elements).
+"""
+# Credits:
+# https://github.com/pf4d/fenics_scripts/blob/master/cbc_block/stokes.py
+from dolfin import *
+from time import time
+from vtkplotter.dolfin import plot, datadir
+
+t0 = time()
+print("calculating... please wait...")
+
+# Load mesh and subdomains
+mesh = Mesh(datadir+"dolfin_fine.xml")
+sub_domains = MeshFunction("size_t", mesh,
+ datadir+"dolfin_fine_subdomains.xml.gz")
+
+# Define function spaces
+P2 = VectorElement("Lagrange", mesh.ufl_cell(), 2)
+P1 = FiniteElement("Lagrange", mesh.ufl_cell(), 1)
+TH = P2 * P1
+W = FunctionSpace(mesh, TH)
+
+# No-slip boundary condition for velocity
+noslip = Constant((0, 0))
+bc0 = DirichletBC(W.sub(0), noslip, sub_domains, 0)
+
+# Inflow boundary condition for velocity
+inflow = Expression(("-sin(x[1]*pi)", "0.0"), degree=2)
+bc1 = DirichletBC(W.sub(0), inflow, sub_domains, 1)
+bcs = [bc0, bc1]
+
+# Define variational problem
+(u, p) = TrialFunctions(W)
+(v, q) = TestFunctions(W)
+f = Constant((0, 0))
+a = (inner(grad(u), grad(v)) - div(v)*p + q*div(u))*dx
+L = inner(f, v)*dx
+w = Function(W)
+
+solve(a == L, w, bcs, solver_parameters={'linear_solver' : 'mumps'})
+tf = time()
+print("time to solve:", tf-t0)
+
+# Split the mixed solution using a shallow copy
+(u, p) = w.split()
+
+##################################
+plot(u, at=0, N=2, text="velocity", mode='mesh and arrows',
+ scale=.03, wire=1, scalarbar=False, style=1)
+plot(p, at=1, text="pressure", cmap='jet')
diff --git a/setup.py b/setup.py
index 85bbb8af..ea51693b 100644
--- a/setup.py
+++ b/setup.py
@@ -2,7 +2,7 @@
setup(
name='vtkplotter',
- version='2019.1.1', # change also in vtkplotter/__init__.py and docs/source/conf.py
+ version='2019.1.2', # change also in vtkplotter/__init__.py and docs/source/conf.py
packages=['vtkplotter'],
scripts=['bin/vtkplotter', 'bin/vtkconvert'],
install_requires=['vtk'],
@@ -78,8 +78,7 @@
# open build/html/index.html
#
# mount_staging
-# cp to ~/Projects/StagingServer/var/www/html/vtkplotter.embl.es
-#
+# cp -r build/html/* ~/Projects/StagingServer/var/www/html/vtkplotter.embl.es/
diff --git a/vtkplotter/__init__.py b/vtkplotter/__init__.py
index a690cbf6..f75f4a6e 100644
--- a/vtkplotter/__init__.py
+++ b/vtkplotter/__init__.py
@@ -46,7 +46,7 @@
__email__ = "marco.musy@embl.es"
__status__ = "dev"
__website__ = "https://github.com/marcomusy/vtkplotter"
-__version__ = "2019.1.1" ### defined also above, in setup.py and docs/source/conf.py
+__version__ = "2019.1.2" ### defined also above, in setup.py and docs/source/conf.py
from vtkplotter.plotter import *
from vtkplotter.analysis import *
@@ -74,175 +74,3 @@
settings._init()
###############
-
-#####################
-def _deprecated_msg(cls):
- print('\nDeprecated in version > 8.9:',
- cls+'(). Use '+ cls.capitalize()+ '() with capital letter instead.\n')
-
-def point(*args, **kwargs):
- """Deprecated. Use capital letter."""
- _deprecated_msg("point")
- return Point(*args, **kwargs)
-
-
-def points(*args, **kwargs):
- """Deprecated. Use capital letter."""
- _deprecated_msg("points")
- return Points(*args, **kwargs)
-
-
-def glyph(*args, **kwargs):
- """Deprecated. Use capital letter."""
- _deprecated_msg("glyph")
- return Glyph(*args, **kwargs)
-
-
-def line(*args, **kwargs):
- """Deprecated. Use capital letter."""
- _deprecated_msg("line")
- return Line(*args, **kwargs)
-
-
-def tube(*args, **kwargs):
- """Deprecated. Use capital letter."""
- _deprecated_msg("tube")
- return Tube(*args, **kwargs)
-
-
-def lines(*args, **kwargs):
- """Deprecated. Use capital letter."""
- _deprecated_msg("lines")
- return Lines(*args, **kwargs)
-
-
-def ribbon(*args, **kwargs):
- """Deprecated. Use capital letter."""
- _deprecated_msg("ribbon")
- return Ribbon(*args, **kwargs)
-
-
-def arrow(*args, **kwargs):
- """Deprecated. Use capital letter."""
- _deprecated_msg("arrow")
- return Arrow(*args, **kwargs)
-
-
-def arrows(*args, **kwargs):
- """Deprecated. Use capital letter."""
- _deprecated_msg("arrows")
- return Arrows(*args, **kwargs)
-
-
-def polygon(*args, **kwargs):
- """Deprecated. Use capital letter."""
- _deprecated_msg("polygon")
- return Polygon(*args, **kwargs)
-
-
-def rectangle(*args, **kwargs):
- """Deprecated. Use capital letter."""
- _deprecated_msg("rectangle")
- return Rectangle(*args, **kwargs)
-
-
-def disc(*args, **kwargs):
- """Deprecated. Use capital letter."""
- _deprecated_msg("disc")
- return Disc(*args, **kwargs)
-
-
-def sphere(*args, **kwargs):
- """Deprecated. Use capital letter."""
- _deprecated_msg("sphere")
- return Sphere(*args, **kwargs)
-
-
-def spheres(*args, **kwargs):
- """Deprecated. Use capital letter."""
- _deprecated_msg("spheres")
- return Spheres(*args, **kwargs)
-
-
-def earth(*args, **kwargs):
- """Deprecated. Use capital letter."""
- _deprecated_msg("earth")
- return Earth(*args, **kwargs)
-
-
-def ellipsoid(*args, **kwargs):
- """Deprecated. Use capital letter."""
- _deprecated_msg("ellipsoid")
- return Ellipsoid(*args, **kwargs)
-
-
-def grid(*args, **kwargs):
- """Deprecated. Use capital letter."""
- _deprecated_msg("grid")
- return Grid(*args, **kwargs)
-
-
-def plane(*args, **kwargs):
- """Deprecated. Use capital letter."""
- _deprecated_msg("plane")
- return Plane(*args, **kwargs)
-
-
-def box(*args, **kwargs):
- """Deprecated. Use capital letter."""
- _deprecated_msg("box")
- return Box(*args, **kwargs)
-
-
-def cube(*args, **kwargs):
- """Deprecated. Use capital letter."""
- _deprecated_msg("cube")
- return Cube(*args, **kwargs)
-
-
-def helix(*args, **kwargs):
- """Deprecated. Use capital letter."""
- _deprecated_msg("helix")
- return Helix(*args, **kwargs)
-
-
-def cylinder(*args, **kwargs):
- """Deprecated. Use capital letter."""
- _deprecated_msg("cylinder")
- return Cylinder(*args, **kwargs)
-
-
-def cone(*args, **kwargs):
- """Deprecated. Use capital letter."""
- _deprecated_msg("cone")
- return Cone(*args, **kwargs)
-
-
-def pyramid(*args, **kwargs):
- """Deprecated. Use capital letter."""
- _deprecated_msg("pyramid")
- return Pyramid(*args, **kwargs)
-
-
-def torus(*args, **kwargs):
- """Deprecated. Use capital letter."""
- _deprecated_msg("torus")
- return Pyramid(*args, **kwargs)
-
-
-def paraboloid(*args, **kwargs):
- """Deprecated. Use capital letter."""
- _deprecated_msg("paraboloid")
- return Paraboloid(*args, **kwargs)
-
-
-def hyperboloid(*args, **kwargs):
- """Deprecated. Use capital letter."""
- _deprecated_msg("hyperboloid")
- return Hyperboloid(*args, **kwargs)
-
-
-def text(*args, **kwargs):
- """Deprecated. Use capital letter."""
- _deprecated_msg("text")
- return Text(*args, **kwargs)
diff --git a/vtkplotter/actors.py b/vtkplotter/actors.py
index ecbbebc7..a7ea0abe 100644
--- a/vtkplotter/actors.py
+++ b/vtkplotter/actors.py
@@ -1209,11 +1209,12 @@ def shrink(self, fraction=0.85):
"""Shrink the triangle polydata in the representation of the input mesh.
Example:
+ .. code-block:: python
- >>> from vtkplotter import load, Sphere, show
- >>> pot = load('data/shapes/teapot.vtk').shrink(0.75)
- >>> s = Sphere(r=0.2).pos(0,0,-0.5)
- >>> show([pot, s])
+ from vtkplotter import load, Sphere, show
+ pot = load('data/shapes/teapot.vtk').shrink(0.75)
+ s = Sphere(r=0.2).pos(0,0,-0.5)
+ show(pot, s)
|shrink| |shrink.py|_
"""
@@ -1698,10 +1699,11 @@ def addCurvatureScalars(self, method=0, lut=None):
:param float lut: optional look up table.
:Example:
-
- >>> from vtkplotter import *
- >>> t = Torus().addCurvatureScalars()
- >>> show(t)
+ .. code-block:: python
+
+ from vtkplotter import *
+ t = Torus().addCurvatureScalars()
+ show(t)
|curvature|
"""
diff --git a/vtkplotter/colors.py b/vtkplotter/colors.py
index 9cb10fc4..be294c11 100644
--- a/vtkplotter/colors.py
+++ b/vtkplotter/colors.py
@@ -301,10 +301,12 @@ def colorMap(value, name="jet", vmin=None, vmax=None):
.. tip:: Can also use directly a matplotlib color map:
:Example:
- >>> from vtkplotter import colorMap
- >>> import matplotlib.cm as cm
- >>> print( colorMap(0.2, cm.flag, 0, 1) )
- (1.0, 0.809016994374948, 0.6173258487801733)
+ .. code-block:: python
+
+ from vtkplotter import colorMap
+ import matplotlib.cm as cm
+ print( colorMap(0.2, cm.flag, 0, 1) )
+ (1.0, 0.809016994374948, 0.6173258487801733)
"""
if not _mapscales:
print("-------------------------------------------------------------------")
@@ -568,6 +570,32 @@ def _has_colors(stream):
"~orangesquare": u"\U0001F538",
"~bluesquare": u"\U0001F537",
"~zzz": u"\U0001F4a4",
+ "~alpha": u"\U000003B1",
+ "~beta": u"\U000003B2",
+ "~gamma": u"\U000003B3",
+ "~delta": u"\U000003B4",
+ "~epsilon": u"\U000003B5",
+ "~eta": u"\U000003B7",
+ "~theta": u"\U000003B8",
+ "~kappa": u"\U000003BA",
+ "~lambda": u"\U000003BB",
+ "~mu": u"\U000003BC",
+ "~nu": u"\U000003BD",
+ "~xi": u"\U000003BE",
+ "~pi": u"\U000003C0",
+ "~rho": u"\U000003C1",
+ "~sigma": u"\U000003C3",
+ "~tau": u"\U000003C4",
+ "~phi": u"\U000003C6",
+ "~chi": u"\U000003C7",
+ "~psi": u"\U000003C8",
+ "~omega": u"\U000003C9",
+ "~Gamma": u"\U00000393",
+ "~Delta": u"\U00000394",
+ "~Lambda": u"\U0000039B",
+ "~Pi": u"\U000003A0",
+ "~Sigma": u"\U000003A3",
+ "~Omega": u"\U000003A9",
}
@@ -591,11 +619,12 @@ def printc(*strings, **keys):
:param end: end character to be printed [return]
:Example:
+ .. code-block:: python
- >>> from vtkplotter.colors import printc
- >>> printc('anything', c='red', bold=False, end='' )
- >>> printc('anything', 455.5, vtkObject, c='green')
- >>> printc(299792.48, c=4) # 4 is blue
+ from vtkplotter.colors import printc
+ printc('anything', c='red', bold=False, end='' )
+ printc('anything', 455.5, vtkObject, c='green')
+ printc(299792.48, c=4) # 4 is blue
.. hint:: |colorprint| |colorprint.py|_
"""
@@ -731,23 +760,26 @@ def printHistogram(data, bins=10, height=10, logscale=False,
:param bool char: use boldface
:param str title: histogram title
- :Example:
+ :Example:
+ .. code-block:: python
- >>> from vtkplotter import printHistogram
- >>> import numpy as np
- >>> d = np.random.normal(size=1000)
- >>> printHistogram(d, c='blue', logscale=True, title='my scalars')
- >>> printHistogram(d, c=1, horizontal=1)
+ from vtkplotter import printHistogram
+ import numpy as np
+ d = np.random.normal(size=1000)
+ printHistogram(d, c='blue', logscale=True, title='my scalars')
+ printHistogram(d, c=1, horizontal=1)
+ |printhisto|
"""
- # Adapted from
- # http://pyinsci.blogspot.com/2009/10/ascii-histograms.html
+ # Adapted from http://pyinsci.blogspot.com/2009/10/ascii-histograms.html
if not horizontal: # better aspect ratio
bins *= 2
h = np.histogram(data, bins=bins)
+ if sys.version_info[0] < 3 and char == u"\U00002589":
+ char = "*" # python2 hack
if char == u"\U00002589" and horizontal:
char = u"\U00002586"
diff --git a/vtkplotter/data/dolfin_fine_subdomains.xml.gz b/vtkplotter/data/dolfin_fine_subdomains.xml.gz
new file mode 100644
index 00000000..65cdc4cc
Binary files /dev/null and b/vtkplotter/data/dolfin_fine_subdomains.xml.gz differ
diff --git a/vtkplotter/docs.py b/vtkplotter/docs.py
index 7c2789f7..549e7899 100644
--- a/vtkplotter/docs.py
+++ b/vtkplotter/docs.py
@@ -723,5 +723,17 @@ def tips():
:target: flatarrow.py_
:alt: flatarrow.py
+.. |printhisto| image:: https://user-images.githubusercontent.com/32848391/55073046-03732780-508d-11e9-9bf9-c5de8631dd73.png
+ :width: 250 px
+
+.. |pmatrix| image:: https://user-images.githubusercontent.com/32848391/55098070-6da3c080-50bd-11e9-8f2b-be94a3f01831.png
+ :width: 250 px
+
+.. |stokes.py| replace:: stokes.py
+.. _stokes.py: https://github.com/marcomusy/vtkplotter/blob/master/examples/other/dolfin/stokes.py
+.. |stokes| image:: https://user-images.githubusercontent.com/32848391/55098209-aba0e480-50bd-11e9-8842-42d3f0b2d9c8.png
+ :width: 250 px
+ :target: stokes.py_
+ :alt: stokes.py
"""
diff --git a/vtkplotter/dolfin.py b/vtkplotter/dolfin.py
index 68495e53..c95ae4ca 100644
--- a/vtkplotter/dolfin.py
+++ b/vtkplotter/dolfin.py
@@ -1,23 +1,35 @@
+#
+#
from __future__ import division, print_function
+
+from vtk.util.numpy_support import numpy_to_vtk
+
import numpy as np
import vtkplotter.utils as utils
-from vtkplotter import docs
+import vtkplotter.docs as docs
-from vtkplotter import shapes, show, clear, Plotter, Text, datadir
-from vtkplotter import Actor, buildPolyData, load, ProgressBar
-from vtkplotter.utils import mag
-from vtkplotter import colors, settings
+import vtkplotter.colors as colors
from vtkplotter.colors import printc, printHistogram
-from vtkplotter.vtkio import screenshot
-from vtk.util.numpy_support import numpy_to_vtk
+
+import vtkplotter.settings as settings
+from vtkplotter.settings import datadir
+
+from vtkplotter.actors import Actor
+
+import vtkplotter.vtkio as vtkio
+from vtkplotter.vtkio import load, ProgressBar, screenshot
+
+import vtkplotter.shapes as shapes
+from vtkplotter.shapes import Text
+
+from vtkplotter.plotter import show, clear, Plotter, plotMatrix
# NB: dolfin does NOT need to be imported at module level
__doc__ = (
"""
FEniCS/Dolfin support submodule.
-`(only tested on python 3.6, dolfin 2018.1.0)`
Install with commands (e.g. in Anaconda):
@@ -37,7 +49,6 @@
plot(mesh)
-
.. image:: https://user-images.githubusercontent.com/32848391/53026243-d2d31900-3462-11e9-9dde-518218c241b6.jpg
Find many more examples in
@@ -61,8 +72,8 @@
"ProgressBar",
"Text",
"datadir",
- "shapes",
"screenshot",
+ "plotMatrix",
]
@@ -342,7 +353,8 @@ def plot(*inputobj, **options):
delta = [u(p) for p in mesh.coordinates()]
#delta = u.compute_vertex_values(mesh) # needs reshape
if u.value_rank() > 0: # wiil show the size of the vector
- actor.pointColors(mag(delta), cmap=cmap, bands=bands, vmin=vmin, vmax=vmax)
+ actor.pointColors(utils.mag(delta),
+ cmap=cmap, bands=bands, vmin=vmin, vmax=vmax)
else:
actor.pointColors(delta, cmap=cmap, bands=bands, vmin=vmin, vmax=vmax)
if scbar and c is None:
@@ -400,7 +412,13 @@ def plot(*inputobj, **options):
else:
bgc = (0.1, 0.1, 0.1)
actors.append(Text(text, c=bgc, font=font))
-
+
+ if 'at' in options.keys() and not 'interactive' in options.keys():
+ if settings.plotter_instance:
+ N = settings.plotter_instance.shape[0]*settings.plotter_instance.shape[1]
+ if options['at'] == N-1:
+ options['interactive'] = True
+
vp = show(actors, **options)
return vp
@@ -410,12 +428,18 @@ class MeshActor(Actor):
"""MeshActor, a vtkActor derived object for dolfin support."""
def __init__(
- self, *inputobj, c="gold", alpha=1, wire=True, bc=None, computeNormals=False
+ self, *inputobj, **options # c="gold", alpha=1, wire=True, bc=None, computeNormals=False
):
+ c = options.pop("c", "gold")
+ alpha = options.pop("alpha", 1)
+ wire = options.pop("wire", True)
+ bc = options.pop("bc", None)
+ computeNormals = options.pop("computeNormals", False)
+
mesh, u = _inputsort(inputobj)
- poly = buildPolyData(mesh)
+ poly = vtkio.buildPolyData(mesh)
Actor.__init__(
self,
@@ -446,7 +470,7 @@ def __init__(
self.addPointScalars(dispsizes, "u_values")
-def MeshPoints(*inputobj, r=5, c="gray", alpha=1):
+def MeshPoints(*inputobj, **options):
"""
Build a point ``Actor`` for a list of points.
@@ -455,6 +479,10 @@ def MeshPoints(*inputobj, r=5, c="gray", alpha=1):
:type c: int, str, list
:param float alpha: transparency in range [0,1].
"""
+ r = options.pop("r", 5)
+ c = options.pop("c", "gray")
+ alpha = options.pop("alpha", 1)
+
mesh, u = _inputsort(inputobj)
plist = mesh.coordinates()
if u:
@@ -480,7 +508,7 @@ def MeshPoints(*inputobj, r=5, c="gray", alpha=1):
return actor
-def MeshLines(*inputobj, scale=1, lw=1, c=None, alpha=1):
+def MeshLines(*inputobj, **options):
"""
Build the line segments between two lists of points `startPoints` and `endPoints`.
`startPoints` can be also passed in the form ``[[point1, point2], ...]``.
@@ -490,6 +518,11 @@ def MeshLines(*inputobj, scale=1, lw=1, c=None, alpha=1):
:param float scale: apply a rescaling factor to the length
"""
+ scale = options.pop("scale", 1)
+ lw = options.pop("lw", 1)
+ c = options.pop("c", None)
+ alpha = options.pop("alpha", 1)
+
mesh, u = _inputsort(inputobj)
startPoints = mesh.coordinates()
u_values = np.array([u(p) for p in mesh.coordinates()])
@@ -512,12 +545,18 @@ def MeshLines(*inputobj, scale=1, lw=1, c=None, alpha=1):
return actor
-def MeshArrows(*inputobj, s=None, scale=1, c="gray", alpha=1, res=12):
+def MeshArrows(*inputobj, **options):
"""
Build arrows representing displacements
:param float s: cross-section size of the arrow
:param float rescale: apply a rescaling factor to the length
"""
+ s = options.pop("s", None)
+ scale = options.pop("scale", 1)
+ c = options.pop("c", "gray")
+ alpha = options.pop("alpha", 1)
+ res = options.pop("res", 12)
+
mesh, u = _inputsort(inputobj)
startPoints = mesh.coordinates()
u_values = np.array([u(p) for p in mesh.coordinates()])
@@ -539,17 +578,14 @@ def MeshArrows(*inputobj, s=None, scale=1, c="gray", alpha=1, res=12):
return actor
-def MeshTensors(*inputobj, c="m", alpha=1, tipSize=1, tipWidth=1):
+def MeshTensors(*inputobj, **options):
"""Not yet implemented."""
- mesh, u = _inputsort(inputobj)
+# c = options.pop("c", "gray")
+# alpha = options.pop("alpha", 1)
+# mesh, u = _inputsort(inputobj)
return
-
-
-
-
-
# -*- coding: utf-8 -*-
# Copyright (C) 2008-2012 Joachim B. Haga and Fredrik Valdmanis
#
diff --git a/vtkplotter/plotter.py b/vtkplotter/plotter.py
index 82b07f88..14ff1e51 100644
--- a/vtkplotter/plotter.py
+++ b/vtkplotter/plotter.py
@@ -66,23 +66,25 @@ def show(*actors, **options
See e.g.: |readVolumeAsIsoSurface.py|_
:return: the current ``Plotter`` class instance.
- .. note:: With multiple renderers, keyword ``at`` can become a `list`, e.g.:
-
- >>> from vtkplotter import *
- >>> s = Sphere()
- >>> c = Cube()
- >>> p = Paraboloid()
- >>> show(s, c, at=[0, 1], shape=(3,1))
- >>> show(p, at=2, interactive=True)
- >>> #
- >>> # is equivalent to:
- >>> vp = Plotter(shape=(3,1))
- >>> s = Sphere()
- >>> c = Cube()
- >>> p = Paraboloid()
- >>> vp.show(s, at=0)
- >>> vp.show(p, at=1)
- >>> vp.show(c, at=2, interactive=True)
+ .. note:: With multiple renderers, keyword ``at`` can become a `list`, e.g.
+
+ .. code-block:: python
+
+ from vtkplotter import *
+ s = Sphere()
+ c = Cube()
+ p = Paraboloid()
+ show(s, c, at=[0, 1], shape=(3,1))
+ show(p, at=2, interactive=True)
+ #
+ # is equivalent to:
+ vp = Plotter(shape=(3,1))
+ s = Sphere()
+ c = Cube()
+ p = Paraboloid()
+ vp.show(s, at=0)
+ vp.show(p, at=1)
+ vp.show(c, at=2, interactive=True)
"""
at = options.pop("at", None)
shape = options.pop("shape", (1, 1))
@@ -205,6 +207,48 @@ def clear(actor=()):
return settings.plotter_instance
+def plotMatrix(M, title='matrix', continuous=True, cmap='Greys'):
+ """
+ Plot a matrix using `matplotlib`.
+
+ :Example:
+ .. code-block:: python
+
+ from vtkplotter.dolfin import plotMatrix
+ import numpy as np
+
+ M = np.eye(9) + np.random.randn(9,9)/4
+
+ plotMatrix(M)
+
+ |pmatrix|
+ """
+ import matplotlib.pyplot as plt
+ import matplotlib as mpl
+ from mpl_toolkits.axes_grid1 import make_axes_locatable
+
+ M = numpy.array(M)
+ m,n = numpy.shape(M)
+ M = M.round(decimals=2)
+
+ fig = plt.figure()
+ ax = fig.add_subplot(111)
+ cmap = mpl.cm.get_cmap(cmap)
+ if not continuous:
+ unq = numpy.unique(M)
+ im = ax.imshow(M, cmap=cmap, interpolation='None')
+ divider = make_axes_locatable(ax)
+ cax = divider.append_axes("right", size="5%", pad=0.05)
+ dim = r'$%i \times %i$ ' % (m,n)
+ ax.set_title(dim + title)
+ ax.axis('off')
+ cb = plt.colorbar(im, cax=cax)
+ if not continuous:
+ cb.set_ticks(unq)
+ cb.set_ticklabels(unq)
+ plt.show()
+
+
########################################################################
class Plotter:
"""
diff --git a/vtkplotter/settings.py b/vtkplotter/settings.py
index ad301d0a..2939d314 100644
--- a/vtkplotter/settings.py
+++ b/vtkplotter/settings.py
@@ -11,6 +11,7 @@
'usingQt',
'renderPointsAsSpheres',
'textures',
+ 'textures_path',
'datadir',
]
diff --git a/vtkplotter/vtkio.py b/vtkplotter/vtkio.py
index 580ae969..2cdf7747 100644
--- a/vtkplotter/vtkio.py
+++ b/vtkplotter/vtkio.py
@@ -850,14 +850,15 @@ class ProgressBar:
Class to print a progress bar with optional text message.
:Example:
+ .. code-block:: python
+
+ import time
+ pb = ProgressBar(0,400, c='red')
+ for i in pb.range():
+ time.sleep(.1)
+ pb.print('some message') # or pb.print(counts=i)
- >>> import time
- >>> pb = ProgressBar(0,400, c='red')
- >>> for i in pb.range():
- >>> time.sleep(.1)
- >>> pb.print('some message') # or pb.print(counts=i)
-
- |progbar|
+ |progbar|
"""
def __init__(self, start, stop, step=1, c=None, ETA=True, width=24, char="="):