diff --git a/docs/01-getting-started.md b/docs/01-getting-started.md
new file mode 100644
index 0000000..9780f49
--- /dev/null
+++ b/docs/01-getting-started.md
@@ -0,0 +1,80 @@
+# Getting started with Java
+
+Prismic makes it easy to get started on a new Java project by providing a specific Java starter project.
+
+## Create a content repository
+
+A content repository is where you define, edit, and publish content.
+
+[**Create Repository**](https://prismic.io/dashboard/new-repository/)
+
+Once your repo is created, setup your custom types and create some content.
+
+## Download the starter project
+
+The Java starter project allows you to query and retrieve content from your Prismic repository and integrate it into your website templates. It's the easiest way to get started with a new project.
+
+[**Download SDK**](https://github.com/prismicio/java-springmvc-starter/archive/master.zip)
+
+## Configure and run your project
+
+Unzip the downloaded file in the desired location for your project.
+
+Replace "lesbonneschoses" in the repository url in your `src/main/webapp/WEB-INF/web.xml` file with your repository name.
+
+```html
+
+
+ endpoint
+ https://your-repo-name.cdn.prismic.io/api
+
+
+```
+
+Fire up a terminal (command prompt or similar on Windows), point it to your project location and run the following command. Note that you will need to have [Maven](https://maven.apache.org/) installed on your machine.
+
+```bash
+mvn jetty:run
+```
+
+You can now open your browser to [http://localhost:8080](http://localhost:8080) and see the project running. It will list your documents which you can click on to get a simple preview of the content.
+
+> **Pagination of API Results**
+>
+> When querying a Prismic repository, your results will be paginated. By default, there are 20 documents per page in the results. You can read more about how to manipulate the pagination in the [Pagination for Results](../02-query-the-api/14-pagination-for-results.md) page.
+
+## And your Prismic journey begins!
+
+Now you're all set to start building your website with Prismic content management. Here are the next steps you need to take.
+
+### Define your Custom Types
+
+First you'll need to model your pages, posts, events, etc. into your Custom Types. Refer to our user-guides to learn more about [constructing your Custom Types](https://user-guides.prismic.io/content-modeling-and-custom-types) using our easy drag-n-drop builder.
+
+### Query your documents
+
+After you've created and published some documents in your repository, you’ll be able to query the API to retrieve your content. We provide explanations and plenty of examples of queries in the documentation. Start by learning more on the [How to Query the API](../02-query-the-api/01-how-to-query-the-api.md) page.
+
+### Integrate content into your templates
+
+The last step is to integrate the content into your templates. Helper functions are provided for each content field type to make integration as easy as possible. Check out our [Templating documentation](../03-templating/01-the-document-object.md) to learn more.
+
+## Working with existing projects
+
+If you:
+
+- Already have a website you want to integrate Prismic to
+- Want to use a different framework than proposed in the SDK
+- or simply prefer to use your own tools to bootstrap the project
+
+You can simply add the library as a dependency as shown below and then follow the instructions in these documentation pages to get up and running.
+
+```html
+
+
+ io.prismic
+ java-kit
+ 1.5.0
+
+```
diff --git a/docs/02-query-the-api/01-how-to-query-the-api.md b/docs/02-query-the-api/01-how-to-query-the-api.md
new file mode 100644
index 0000000..0f8c4f1
--- /dev/null
+++ b/docs/02-query-the-api/01-how-to-query-the-api.md
@@ -0,0 +1,87 @@
+# How to query the API
+
+In order to retrieve the content from your repository, you will need to query the repository API. When you create your query you will specify exactly what it is you are looking for. You could query the repository for all the documents of certain type or retrieve the one specific document you need.
+
+Let's take a look at how to put together queries for whatever case you need.
+
+## The Basics
+
+When retrieving content from your Prismic repository, here's what a typical query looks like.
+
+```java
+Response response = api.query(Predicates.at("document.type", "blog-post"))
+ .orderings("my.blog-post.date desc")
+ .submit();
+List documents = response.getResults();
+```
+
+This is the basic format of a query. In the query you have two parts, the _Predicate_ and the _options_.
+
+## Predicates
+
+In the above example we had the following predicate.
+
+```java
+Predicates.at("document.type", "blog-post")
+```
+
+The predicate(s) will define which documents are retrieved from the content repository. This particular example will retrieve all of the documents of the type "blog-post".
+
+The first part, "document.type" is the *path*, or what the query will be looking for. The second part of the predicate in the above example is the _value_, which in this case is:\*\* \*\*"blog-post".
+
+You can combine more than one predicate together to refine your query. You just need to comma-separate your predicates in the query method as shown below.
+
+```java
+.query(
+ Predicates.at("document.type", "blog-post"),
+ Predicates.at("document.tags", Arrays.asList("featured"))
+)
+```
+
+This particular query will retrieve all the documents of the "blog-post" type that also have the tag "featured".
+
+## Options
+
+In the second part of the query, you can include the options needed for that query. In the above example we used _desc \_to retrieve the response in descending order, you can also use \_asc_ for ascending order.
+
+```java
+.orderings("my.blog-post.date desc")
+```
+
+The following options let you specify how the returned list of documents will be ordered. You can include more than one option, by adding al the needed options as shown below.
+
+```java
+.pageSize(10)
+.page(2)
+```
+
+You will find a list and description of all the available options on the [Query Options Reference](../02-query-the-api/03-query-options-reference.md) page.
+
+> **Pagination of API Results**
+>
+> When querying a Prismic repository, your results will be paginated. By default, there are 20 documents per page in the results. You can read more about how to manipulate the pagination in the [Pagination for Results](../02-query-the-api/14-pagination-for-results.md) page.
+
+## Submit the query
+
+After specifying the query and the options, you need to submit the query using the following method.
+
+```java
+.submit()
+```
+
+## Putting it all together
+
+Here's another example of a more advanced query with multiple predicates and multiple options:
+
+```java
+Response response = api.query(
+ Predicates.at("document.type", "blog-post"),
+ Predicates.at("document.tags", Arrays.asList("featured"))
+).orderings("my.blog-post.date desc")
+.pageSize(10)
+.page(1)
+.submit();
+List documents = response.getResults();
+```
+
+Whenever you query your content, you end up with the response object stored in the defined variable. In this example the variable was called _response_
diff --git a/docs/02-query-the-api/02-date-and-time-based-predicate-reference.md b/docs/02-query-the-api/02-date-and-time-based-predicate-reference.md
new file mode 100644
index 0000000..b32c53c
--- /dev/null
+++ b/docs/02-query-the-api/02-date-and-time-based-predicate-reference.md
@@ -0,0 +1,471 @@
+# Date & Time based Predicate Reference
+
+This page describes and gives examples for all the date and time based predicates you can use when creating queries with the Java development kit
+
+All of these predicates will work when used with either the Date or Timestamp fields, as well as the first and last publication dates.
+
+Note that when using any of these predicates with either a Date or Timestamp field, you will limit the results of the query to the specified custom type.
+
+## dateAfter
+
+The `dateAfter` predicate checks that the value in the path is after the date value passed into the predicate.
+
+This will not include anything with a date equal to the input value.
+
+```
+Predicates.dateAfter( path, date )
+```
+
+| Property | Description |
+| ------------------------------------------------------ | ---------------------------------------------------------------------------------------------------------- |
+| path accepted paths |
document.first_publication_date
document.last_publication_date
my.{custom-type}.{field}
|
+| date DateTime object |
new DateTime(year, month, day, hour, minute)
|
+
+Examples:
+
+```
+Predicates.dateAfter("document.first_publication_date", new DateTime(2020, 5, 18, 0, 0))
+Predicates.dateAfter("document.last_publication_date", new DateTime(2020, 7, 22, 0, 0))
+Predicates.dateAfter("my.article.release_date", new DateTime(2019, 1, 23, 0, 0))
+```
+
+Note that in order to use the DateTime object you need to include the DateTime class by including the following in your code.
+
+```javascript
+import org.joda.time.DateTime;
+```
+
+## dateBefore
+
+The `dateBefore` predicate checks that the value in the path is before the date value passed into the predicate.
+
+This will not include anything with a date equal to the input value.
+
+```
+Predicates.dateBefore( path, date )
+```
+
+| Property | Description |
+| ------------------------------------------------------ | ---------------------------------------------------------------------------------------------------------- |
+| path accepted paths |
document.first_publication_date
document.last_publication_date
my.{custom-type}.{field}
|
+| date DateTime object |
new DateTime(year, month, day, hour, minute)
|
+
+Examples:
+
+```
+Predicates.dateBefore("document.first_publication_date", new DateTime(2020, 9, 19, 0, 0))
+Predicates.dateBefore("document.last_publication_date", new DateTime(2020, 10, 15, 0, 0))
+Predicates.dateBefore("my.post.date", new DateTime(2020, 8, 24, 0, 0))
+```
+
+Note that in order to use the DateTime object you need to include the DateTime class by including the following in your code.
+
+```
+import org.joda.time.DateTime;
+```
+
+## dateBetween
+
+The `dateBetween` predicate checks that the value in the path is within the date values passed into the predicate.
+
+```
+Predicates.dateBetween( path, startDate, endDate )
+```
+
+| Property | Description |
+| ----------------------------------------------------------- | ---------------------------------------------------------------------------------------------------------- |
+| path accepted paths |
document.first_publication_date
document.last_publication_date
my.{custom-type}.{field}
|
+| startDate DateTime object |
new DateTime(year, month, day, hour, minute)
|
+| endDate DateTime object |
new DateTime(year, month, day, hour, minute)
|
+
+Examples:
+
+```
+Predicates.dateBetween("document.first_publication_date", new DateTime(2020, 1, 16, 0, 0), new DateTime(2017, 2, 16, 0, 0))
+Predicates.dateBetween("document.last_publication_date", new DateTime(2020, 1, 16, 0, 0), new DateTime(2017, 2, 16, 0, 0))
+Predicates.dateBetween("my.blog_post.post_date", new DateTime(2020, 6, 1, 0, 0), new DateTime(2020, 6, 30, 0, 0))
+```
+
+Note that in order to use the DateTime object you need to include the DateTime class by including the following in your code.
+
+```
+import org.joda.time.DateTime;
+```
+
+## dayOfMonth
+
+The `dayOfMonth` predicate checks that the value in the path is equal to the day of the month passed into the predicate.
+
+```
+Predicates.dayOfMonth( path, day )
+```
+
+| Property | Description |
+| ----------------------------------------------------- | ---------------------------------------------------------------------------------------------------------- |
+| path accepted paths |
document.first_publication_date
document.last_publication_date
my.{custom-type}.{field}
|
+| day integer |
Day of the month
|
+
+Examples:
+
+```
+Predicates.dayOfMonth("document.first_publication_date", 22)
+Predicates.dayOfMonth("document.last_publication_date", 30)
+Predicates.dayOfMonth("my.post.date", 14)
+```
+
+## dayOfMonthAfter
+
+The `dayOfMonthAfter` predicate checks that the value in the path is after the day of the month passed into the predicate.
+
+Note that this will return only the days after the specified day of the month. It will not return any documents where the day is equal to the specified day.
+
+```
+Predicates.dayOfMonthAfter( path, day )
+```
+
+| Property | Description |
+| ----------------------------------------------------- | ---------------------------------------------------------------------------------------------------------- |
+| path accepted paths |
document.first_publication_date
document.last_publication_date
my.{custom-type}.{field}
|
+| day integer |
Day of the month
|
+
+Examples:
+
+```
+Predicates.dayOfMonthAfter("document.first_publication_date", 10)
+Predicates.dayOfMonthAfter("document.last_publication_date", 15)
+Predicates.dayOfMonthAfter("my.event.date_and_time", 21)
+```
+
+## dayOfMonthBefore
+
+The `dayOfMonthBefore` predicate checks that the value in the path is before the day of the month passed into the predicate.
+
+Note that this will return only the days before the specified day of the month. It will not return any documents where the date is equal to the specified day.
+
+```
+Predicates.dayOfMonthBefore( path, day )
+```
+
+| Property | Description |
+| ----------------------------------------------------- | ---------------------------------------------------------------------------------------------------------- |
+| path accepted paths |
document.first_publication_date
document.last_publication_date
my.{custom-type}.{field}
|
+| day integer |
Day of the month
|
+
+Examples:
+
+```
+Predicates.dayOfMonthBefore("document.first_publication_date", 15)
+Predicates.dayOfMonthBefore("document.last_publication_date", 10)
+Predicates.dayOfMonthBefore("my.blog_post.release_date", 23)
+```
+
+## dayOfWeek
+
+The `dayOfWeek` predicate checks that the value in the path is equal to the day of the week passed into the predicate.
+
+```
+Predicates.dayOfWeek( path, weekDay )
+```
+
+| Property | Description |
+| ---------------------------------------------------------- | ---------------------------------------------------------------------------------------------------------- |
+| path accepted paths |
|
+
+Examples:
+
+```
+Predicates.dayOfWeek("document.first_publication_date", Predicates.DayOfWeek.MONDAY)
+Predicates.dayOfWeek("document.last_publication_date", Predicates.DayOfWeek.SUNDAY)
+Predicates.dayOfWeek("my.concert.show_date", Predicates.DayOfWeek.FRIDAY)
+```
+
+## dayOfWeekAfter
+
+The `dayOfWeekAfter` predicate checks that the value in the path is after the day of the week passed into the predicate.
+
+This predicate uses Monday as the beginning of the week:
+
+1. Monday
+1. Tuesday
+1. Wednesday
+1. Thursday
+1. Friday
+1. Saturday
+1. Sunday
+
+Note that this will return only the days after the specified day of the week. It will not return any documents where the day is equal to the specified day.
+
+```
+Predicates.dayOfWeekAfter( path, weekDay )
+```
+
+| Property | Description |
+| ---------------------------------------------------------- | ---------------------------------------------------------------------------------------------------------- |
+| path accepted paths |
|
+
+Examples:
+
+```
+Predicates.dayOfWeekAfter("document.first_publication_date", Predicates.DayOfWeek.FRIDAY)
+Predicates.dayOfWeekAfter("document.last_publication_date", Predicates.DayOfWeek.THURSDAY)
+Predicates.dayOfWeekAfter("my.blog_post.date", Predicates.DayOfWeek.TUESDAY)
+```
+
+## dayOfWeekBefore
+
+The `dayOfWeekBefore` predicate checks that the value in the path is before the day of the week passed into the predicate.
+
+This predicate uses Monday as the beginning of the week:
+
+1. Monday
+1. Tuesday
+1. Wednesday
+1. Thursday
+1. Friday
+1. Saturday
+1. Sunday
+
+Note that this will return only the days before the specified day of the week. It will not return any documents where the day is equal to the specified day.
+
+```
+Predicates.dayOfWeekBefore( path, weekDay )
+```
+
+| Property | Description |
+| ---------------------------------------------------------- | ---------------------------------------------------------------------------------------------------------- |
+| path accepted paths |
|
+
+Examples:
+
+```
+Predicates.month("document.first_publication_date", Predicates.Month.AUGUST)
+Predicates.month("document.last_publication_date", Predicates.Month.SEPTEMBER)
+Predicates.month("my.blog_post.date", Predicates.Month.JANUARY)
+```
+
+## monthAfter
+
+The `monthAfter` predicate checks that the value in the path occurs in any month after the value passed into the predicate.
+
+Note that this will only return documents where the date is after the specified month. It will not return any documents where the date is within the specified month.
+
+```
+Predicates.monthAfter( path, month )
+```
+
+| Property | Description |
+| ----------------------------------------------------- | ---------------------------------------------------------------------------------------------------------- |
+| path accepted paths |
|
+
+Examples:
+
+```
+Predicates.monthAfter("document.first_publication_date", Predicates.Month.FEBRUARY)
+Predicates.monthAfter("document.last_publication_date", Predicates.Month.JUNE)
+Predicates.monthAfter("my.article.date", Predicates.Month.OCTOBER)
+```
+
+## monthBefore
+
+The `monthBefore` predicate checks that the value in the path occurs in any month before the value passed into the predicate.
+
+Note that this will only return documents where the date is before the specified month. It will not return any documents where the date is within the specified month.
+
+```
+Predicates.monthBefore( path, month )
+```
+
+| Property | Description |
+| ----------------------------------------------------- | ---------------------------------------------------------------------------------------------------------- |
+| path accepted paths |
|
+
+Examples:
+
+```
+Predicates.monthBefore("document.first_publication_date", Predicates.Month.AUGUST)
+Predicates.monthBefore("document.last_publication_date", Predicates.Month.JUNE)
+Predicates.monthBefore("my.blog_post.release_date", Predicates.Month.SEPTEMBER)
+```
+
+## year
+
+The `year` predicate checks that the value in the path occurs in the year value passed into the predicate.
+
+```
+Predicates.year( path, year )
+```
+
+| Property | Description |
+| ----------------------------------------------------- | ---------------------------------------------------------------------------------------------------------- |
+| path accepted paths |
document.first_publication_date
document.last_publication_date
my.{custom-type}.{field}
|
+| year integer |
Year
|
+
+Examples:
+
+```
+Predicates.year("document.first_publication_date", 2020)
+Predicates.year("document.last_publication_date", 2021)
+Predicates.year("my.employee.birthday", 1986)
+```
+
+## hour
+
+The `hour` predicate checks that the value in the path occurs within the hour value passed into the predicate.
+
+This uses the 24 hour system, starting at 0 and going through 23.
+
+Note that this predicate will technically work for a Date field, but won’t be very useful. All date field values are automatically given an hour of 0.
+
+```
+Predicates.hour( path, hour )
+```
+
+| Property | Description |
+| ----------------------------------------------------- | ---------------------------------------------------------------------------------------------------------- |
+| path accepted paths |
document.first_publication_date
document.last_publication_date
my.{custom-type}.{field}
|
+| hour integer |
Hour between 0 and 23
|
+
+Examples:
+
+```
+Predicates.hour("document.first_publication_date", 12)
+Predicates.hour("document.last_publication_date", 8)
+Predicates.hour("my.event.date_and_time", 19)
+```
+
+## hourAfter
+
+The `hourAfter` predicate checks that the value in the path occurs after the hour value passed into the predicate.
+
+This uses the 24 hour system, starting at 0 and going through 23.
+
+> Note that this will only return documents where the timestamp is after the specified hour. It will not return any documents where the timestamp is within the specified hour.
+
+This predicate will technically work for a Date field, but won’t be very useful. All date field values are automatically given an hour of 0.
+
+```
+Predicates.hourAfter( path, hour )
+```
+
+| Property | Description |
+| ----------------------------------------------------- | ---------------------------------------------------------------------------------------------------------- |
+| path accepted paths |
document.first_publication_date
document.last_publication_date
my.{custom-type}.{field}
|
+| hour integer |
Hour between 0 and 23
|
+
+Examples:
+
+```
+Predicates.hourAfter("document.first_publication_date", 21)
+Predicates.hourAfter("document.last_publication_date", 8)
+Predicates.hourAfter("my.blog_post.release_date", 16)
+```
+
+## hourBefore
+
+The `hourBefore` predicate checks that the value in the path occurs before the hour value passed into the predicate.
+
+This uses the 24 hour system, starting at 0 and going through 23.
+
+> Note that this will only return documents where the timestamp is before the specified hour. It will not return any documents where the timestamp is within the specified hour.
+
+This predicate will technically work for a Date field, but won’t be very useful. All date field values are automatically given an hour of 0.
+
+```
+Predicates.hourBefore( path, hour )
+```
+
+| Property | Description |
+| ----------------------------------------------------- | ---------------------------------------------------------------------------------------------------------- |
+| path accepted paths |
document.first_publication_date
document.last_publication_date
my.{custom-type}.{field}
|
+| hour integer |
Hour between 0 and 23
|
+
+Examples:
+
+```
+Predicates.hourBefore("document.first_publication_date", 10)
+Predicates.hourBefore("document.last_publication_date", 14)
+Predicates.hourBefore("my.event.date_and_time", 12)
+```
diff --git a/docs/02-query-the-api/03-query-options-reference.md b/docs/02-query-the-api/03-query-options-reference.md
new file mode 100644
index 0000000..fdc7cfb
--- /dev/null
+++ b/docs/02-query-the-api/03-query-options-reference.md
@@ -0,0 +1,213 @@
+# Query options reference
+
+Here you will find a complete reference about the various query options.
+
+## Adding an option method
+
+It is simple to to add a query option to a query when using the prismic.io Java development kit. You just need to add the specific option method after the `query()` method and before the `submit()` method.
+
+Here is an example of a query that uses the query option method, `fetch()`.
+
+```
+Response response = api.query(Predicates.at("document.type", "page"))
+ .fetch("page.title")
+ .submit();
+List documents = response.getResults();
+```
+
+Now we will describe each available query option.
+
+## after
+
+The `after` option can be used along with the orderings option. It will remove all the documents except for those after the specified document in the list.
+
+To clarify, let’s say you have a query that return the following documents in this order:
+
+- `V9Zt3icAAAl8Uzob (Page 1)`
+- `PqZtvCcAALuRUzmO (Page 2)`
+- `VkRmhykAAFA6PoBj (Page 3)`
+- `V4Fs8rDbAAH9Pfow (Page 4)`
+- `G8ZtxQhAALuSix6R (Page 5)`
+- `Ww9yuAvdAhl87wh6 (Page 6)`
+
+If you add the `after` option and specify page 3, “VkRmhykAAFA6PoBj”, your query will return the following:
+
+- `V4Fs8rDbAAH9Pfow (Page 4)`
+- `G8ZtxQhAALuSix6R (Page 5)`
+- `Ww9yuAvdAhl87wh6 (Page 6)`
+
+By reversing the orderings in your query, you can use this same method to retrieve all the documents before the specified document.
+
+This option is useful when creating a navigation for a blog.
+
+```java
+.set("after", "VkRmhykAAFA6PoBj")
+```
+
+## fetch
+
+The `fetch` option is used to make queries faster by only retrieving the specified field(s).
+
+To retrieve a single field, simply specify the field as shown below.
+
+```java
+.fetch("product.title")
+```
+
+To retrieve more than one field, you just need to comma-separate all the fields you wish included as shown below.
+
+```java
+.fetch("product.title, product.price")
+```
+
+## fetchLinks
+
+The `fetchLinks` option allows you to retrieve a specific content field from a linked document and add it to the document response object.
+
+Note that this will only retrieve content of the following field types:
+
+- Color
+- Content Relationship
+- Date
+- Image
+- Key Text
+- Number
+- Rich Text (but only returns the first element)
+- Select
+- Timestamp
+- Title
+
+It is **not** possible to retrieve the following content field types:
+
+- Embed
+- GeoPoint
+- Link
+- Link to Media
+- Rich Text (anything other than the first element)
+- Any field in a Group or Slice
+
+The value you enter for the fetchLinks option needs to take the following format:
+
+```java
+.fetchLinks("{custom-type}.{field}")
+```
+
+| Property | Description |
+| ----------------------------------- | ---------------------------------------------------------------------------- |
+| {custom-type} |
The custom type API-ID of the linked document
|
+| {field} |
The API-ID of the field you wish to retrieve from the linked document
|
+
+Examples:
+
+```java
+.fetchLinks("author.full_name")
+.fetchLinks("author.first_name, author.last_name")
+```
+
+## lang
+
+The `lang` option defines the language code for the results of your query.
+
+### Specify a particular language/region
+
+You can use the *lang* option to specify a particular language/region you wish to query by. You just need to set the lang value to the desired language code, for example "en-us" for American English.
+
+If no *lang* option is provided, then the query will default to the master language of the repository.
+
+```java
+.lang("en-us")
+```
+
+### Query all languages
+
+You can also use the *lang* option to specify that you want to query documents in all available languages. Simply set the *lang* option to the wildcard value `*`.
+
+```java
+.lang("*")
+```
+
+To view a complete example of how this option works, view the examples on the [Query by Language](../02-query-the-api/15-query-by-language.md) page.
+
+## orderings
+
+The `orderings()` option orders the results by the specified field(s). You can specify as many fields as you want/need.
+
+| Property | Description |
+| --------------------------------------- | ---------------------------------------------------------------------------------------------- |
+| lowest to highest |
It will automatically order the field from lowest to highest
|
+| highest to lowest |
Use "desc" next to the field name to instead order it from greatest to lowest
|
+
+```java
+.orderings("my.product.price") // lowest to highest
+.orderings("my.product.price desc") // highest to lowest
+```
+
+### Multiple orderings
+
+You can specify more than one field to order your results by. To do so, simply comma-separate the orderings in the string.
+
+The results will be ordered by the first field in the array. If any of the results have the same value for that initial sort, they will then be sorted by the next specified field. And so on.
+
+Here is an example that first sorts the products by price from lowest to highest. If any of the products have the same price, then they will be sorted by their titles.
+
+```java
+.orderings("my.product.price, my.product.title")
+```
+
+### Sort by publication date
+
+It is also possible to order documents by their first or last publication dates.
+
+| Property | Description |
+| -------------------------------------------- | ------------------------------------------------------------------------------ |
+| first_publication_date |
The date that the document was originally published for the first time
|
+| last_publication_date |
The most recent date that the document has been published after editing
|
+
+```java
+.orderings("document.first_publication_date") // first publication date
+.orderings("document.last_publication_date") // last publication date
+```
+
+## page
+
+The `page` option defines the pagination for the result of your query.
+
+If unspecified, the pagination will default to "1", corresponding to the first page of results.
+
+| Property | Description |
+| ----------------------------------------------- | --------------------------------------------------- |
+| value integer |
page index (1 = 1st page, 2 = 2nd page, ...)
|
+
+```java
+.page(2)
+```
+
+## pageSize
+
+Your query results are always paginated.
+
+The `pageSize()` option defines the maximum number of documents per page for the pagination.
+
+If left unspecified, the page size will default to 20. The maximum page size is 100.
+
+| Property | Description |
+| ----------------------------------------------- | ----------------------------------------------- |
+| value integer |
pagination page size (between 1 and 100)
|
+
+```java
+.pageSize(100)
+```
+
+## ref
+
+The `ref` option defines which version of your content to query.
+
+By default the Prismic Java development kit will use the master ref to retrieve the currently published documents.
+
+| Property | Description |
+| ---------------------------------------------- | -------------------------------------------------- |
+| value string |
Master, Release, Experiment, or Preview ref
|
+
+```java
+.ref("Wst7PCgAAHUAvviX")
+```
diff --git a/docs/02-query-the-api/04-quick-query-helper-functions.md b/docs/02-query-the-api/04-quick-query-helper-functions.md
new file mode 100644
index 0000000..b4e4782
--- /dev/null
+++ b/docs/02-query-the-api/04-quick-query-helper-functions.md
@@ -0,0 +1,99 @@
+# Query Helper functions
+
+We've included helper functions to make creating certain queries quicker and easier when using the Java development kit. This page provides the description and examples for each of the helper functions.
+
+## getByUID
+
+The `getByUID` function is used to query the specified custom type by a certain UID. This requires that the custom type of the document contains the UID field.
+
+This function will only ever retrieve one document as there can only be one instance of a given UID for each custom type.
+
+```css
+api.getByUID( customType, uid, ref, lang )
+```
+
+| Property | Description |
+| --------------------------------------------------- | --------------------------------------------------------------------- |
+| customType String |
(required) The API ID of the custom type you are searching for
|
+| uid String |
(required) The UID of the document you want to retrieve
|
+| ref String |
(optional) The ref you wish to query
|
+| lang String |
(optional) The language code for the document you are querying
|
+
+Here is an example that queries a document of the type "page" by its UID "about-us".
+
+```javascript
+Document document = api.getByUID("page", "about-us");
+// document contains the document content
+```
+
+Here is an example that shows how to pass a ref and a language option into the query.
+
+```javascript
+String ref = api.getMaster().getRef();
+Document document = api.getByUID("page", "about-us", ref, "en-us");
+// document contains the document content
+```
+
+## getByID
+
+The `getByID` function is used to query a certain document by its id. Every document is automatically assigned a unique id when it is created. The id will look something like this: "WAjgAygABN3B0a-a".
+
+This function will only ever retrieve one document as each document has a unique id value.
+
+```css
+api.getByID( id, ref, lang )
+```
+
+| Property | Description |
+| --------------------------------------------- | --------------------------------------------------------------------- |
+| id String |
(required) The ID of the document you want to retrieve
|
+| ref String |
(optional) The ref you wish to query
|
+| lang String |
(optional) The language code for the document you are querying
|
+
+Here is a simple example that retrieves the document with the ID "WAjgAygABN3B0a-a".
+
+```javascript
+Document document = api.getByID("WAjgAygABN3B0a-a");
+// document contains the document content
+```
+
+Here is an example that shows how to pass a ref and a language option into the query.
+
+```javascript
+String ref = api.getMaster().getRef();
+Document document = api.getByID("WAjgAygABN3B0a-a", ref, "en-us");
+// document contains the document content
+```
+
+## getByIDs
+
+The `getByIDs` function is used to query multiple documents by their ids.
+
+This will return the documents in the same order specified in the array, unless options are added to sort them otherwise.
+
+```css
+api.getByIDs( ids, ref, lang )
+```
+
+| Property | Description |
+| ------------------------------------------------------------ | ---------------------------------------------------------------------------------------- |
+| ids Iterable<String> |
(required) An array of strings with the ids of the documents you want to retrieve
|
+| ref String |
(optional) The ref you wish to query
|
+| lang String |
(optional) The language code for the documents you are querying
|
+
+Here is an example that queries multiple documents by their ids.
+
+```javascript
+List ids = Arrays.asList("WAjgAygAAN3B0a-a", "WC7GECUAAHBHQd-Y", "WEE_gikAAC2feA-z");
+Response response = api.getByIDs(ids).submit();
+List documents = response.getResults();
+```
+
+Here is an example that shows how to pass a ref and a language option into the query.
+
+```javascript
+List ids = Arrays.asList("WAjgAygAAN3B0a-a", "WC7GECUAAHBHQd-Y", "WEE_gikAAC2feA-z");
+String ref = api.getMaster().getRef();
+Response response = api.getByIDs(ids, ref, "en-us").submit();
+List documents = response.getResults();
+```
diff --git a/docs/02-query-the-api/05-query-single-type.md b/docs/02-query-the-api/05-query-single-type.md
new file mode 100644
index 0000000..4a58e04
--- /dev/null
+++ b/docs/02-query-the-api/05-query-single-type.md
@@ -0,0 +1,31 @@
+# Query a Single Type document
+
+Here we discuss how to query a Single Type document
+
+> **Querying by Language**
+>
+> Note that if you are trying to query a document that isn't in the master language of your repository, you will need to specify the language code. You can read how to do this on the [Query by Language page](../02-query-the-api/15-query-by-language.md).
+
+## Without Query Options
+
+In this example we are querying for the single instance of the custom type "homepage".
+
+```
+Response response = api.query(
+ Predicates.at("document.type", "homepage")
+).submit();
+Document document = response.getResults().get(0);
+// document contains the document content
+```
+
+## With Query Options
+
+You can perform the same query and add query options. Here we again query the single document of the type "homepage" and add a fetchLinks option.
+
+```
+Response response = api.query(
+ Predicates.at("document.type", "homepage")
+).fetchLinks("page.title").submit();
+Document document = response.getResults().get(0);
+// document contains the document content
+```
diff --git a/docs/02-query-the-api/06-query-by-ids-or-uids.md b/docs/02-query-the-api/06-query-by-ids-or-uids.md
new file mode 100644
index 0000000..d51971f
--- /dev/null
+++ b/docs/02-query-the-api/06-query-by-ids-or-uids.md
@@ -0,0 +1,104 @@
+# Query by ID or UID
+
+You can retrieve either multiple documents or a single one by their document ID or UID.
+
+> **Querying by Language**
+>
+> Note that if you are trying to query a document that isn't in the master language of your repository, you will need to specify the language code or wildcard language value. You can read how to do this on the [Query by Language page](../02-query-the-api/15-query-by-language.md).
+>
+> If you are using one of the query helper functions below, you do not need to do this.
+
+## Query a document by ID
+
+We've created a helper function that makes it easy to query by ID, but it is also possible to do this without the helper function.
+
+### getByID helper function
+
+Here is an example that shows how to query a document by its ID using the `getByID` helper function.
+
+```javascript
+Document document = api.getByID("WAjgAygAAN3B0a-a");
+// document contains the document content
+```
+
+### Without the helper function
+
+Here we perform the same query for a document by its ID without using the helper function. This allows us to add a query option, in this case limiting the results to just the "page.title" field.
+
+```javascript
+Response response = api.query(Predicates.at("document.id", "WAjgAygAAN3B0a-a"))
+ .fetch("page.title")
+ .submit();
+Document document = response.getResults().get(0);
+// document contains the document content
+```
+
+## Query multiple documents by IDs
+
+We've created a helper function that makes it easy to query multiple documents by IDs.
+
+### getByIDs helper function
+
+Here is an example of querying multiple documents by their ids using the `getByIDs` helper function.
+
+```javascript
+List ids = Arrays.asList("WAjgAygAAN3B0a-a", "WC7GECUAAHBHQd-Y", "WEE_gikAAC2feA-z");
+Response response = api.getByIDs(ids).submit();
+List documents = response.getResults();
+```
+
+### Without the helper function
+
+Here is an example of how to perform the same query as above, but this time without using the helper function. This allows us to add query options, in this case limiting the content to just the "page.title" field.
+
+```javascript
+List ids = Arrays.asList("WAjgAygAAN3B0a-a", "WC7GECUAAHBHQd-Y", "WEE_gikAAC2feA-z");
+Response response = api.query(Predicates.in("document.id", ids))
+ .fetch("page.title")
+ .submit();
+List documents = response.getResults();
+```
+
+## Query a document by its UID
+
+If you have added the UID field to a custom type, you can query a document by its UID.
+
+### getByUID helper function
+
+Here is an example showing how to query a document of the type "page" by its UID "about-us" using the `getByUID` helper function.
+
+```javascript
+Document document = api.getByUID("page", "about-us");
+// document contains the document content
+```
+
+### Without the helper function
+
+Here is an example of the same query without using the helper function. It will query the document of the type "page" that contains the UID "about us".
+
+This allows us to add a query option, in this case limiting the results to just the "page.title" field.
+
+```javascript
+Response response = api.query(Predicates.at("my.page.uid", "about-us"))
+ .fetch("page.title")
+ .submit();
+Document document = response.getResults().get(0);
+// document contains the document content
+```
+
+### Redirecting old UIDs
+
+When querying a document by UID, older UID values will also return your document. This ensures that existing links are not broken when the UID is changed.
+
+For better search engines ranking (SEO), you may want to redirect old URLs to the latest URL rather than serving the same content on both old and new:
+
+```javascript
+// uid is a string
+Document document = api.getByUID("page", uid);
+if (document.getUid() != uid) {
+ // Redirect to the URL corresponding to the actual UID
+ response.sendRedirect("/" + document.getUid());
+} else {
+ // Render content
+}
+```
diff --git a/docs/02-query-the-api/07-query-all-documents.md b/docs/02-query-the-api/07-query-all-documents.md
new file mode 100644
index 0000000..660fb77
--- /dev/null
+++ b/docs/02-query-the-api/07-query-all-documents.md
@@ -0,0 +1,25 @@
+# Query all documents
+
+This page shows you how to query all the documents in your repository.
+
+## Without query options
+
+Here is an example that will query your repository for all documents by using an empty query.
+
+By default, the API will paginate the results, with 20 documents per page.
+
+```
+Response response = api.query().submit();
+List documents = response.getResults();
+```
+
+## With query options
+
+You can add options to this query. In the following example we allow 100 documents per page for the query response.
+
+```
+Response response = api.query()
+ .pageSize(100)
+ .submit();
+List documents = response.getResults();
+```
diff --git a/docs/02-query-the-api/08-query-by-type.md b/docs/02-query-the-api/08-query-by-type.md
new file mode 100644
index 0000000..6a351b8
--- /dev/null
+++ b/docs/02-query-the-api/08-query-by-type.md
@@ -0,0 +1,41 @@
+# Query by Type
+
+Here we discuss how to query all the documents of a certain custom type.
+
+## By One Type
+
+### Example 1
+
+This first example shows how to query all of the documents of the type "blog-post". The option included in this query will sort the results by their "date" field (from most recent to the oldest).
+
+```
+Response response = api.query(
+ Predicates.at("document.type", "blog-post")
+).orderings("my.blog-post.date desc").submit();
+List documents = response.getResults();
+```
+
+### Example 2
+
+The following example shows how to query all of the documents of the type "video-game". The options will make it so that the results are sorted alphabetically, limited to 10 games per page, and showing the second page of results.
+
+```
+Response response = api.query(
+ Predicates.at("document.type", "video-game")
+ ).pageSize(10)
+ .page(2)
+ .orderings("my.video-game.title")
+ .submit();
+List documents = response.getResults();
+```
+
+## By Multiple Types
+
+This example shows how to query all of the documents of two different types: "article" and "blog_post".
+
+```
+Response response = api.query(
+ Predicates.any("document.type", Arrays.asList("article", "blog_post"))
+ ).submit();
+List documents = response.getResults();
+```
diff --git a/docs/02-query-the-api/09-query-by-tag.md b/docs/02-query-the-api/09-query-by-tag.md
new file mode 100644
index 0000000..e6180fe
--- /dev/null
+++ b/docs/02-query-the-api/09-query-by-tag.md
@@ -0,0 +1,25 @@
+# Query by Tag
+
+Here we show how to query all of the documents with a certain tag.
+
+## Query by a single tag
+
+This example shows how to query all the documents with the tag "Featured" by using the `at` predicate.
+
+```
+Response response = api.query(
+ Predicates.at("document.tags", Arrays.asList("Featured"))
+).submit();
+List documents = response.getResults();
+```
+
+## Query multiple tags
+
+The following example shows how to query all of the documents with either the tag "Tag 1" or "Tag 2" by using the `any` predicate.
+
+```
+Response response = api.query(
+ Predicates.any("document.tags", Arrays.asList("Tag 1", "Tag 2"))
+).submit();
+List documents = response.getResults();
+```
diff --git a/docs/02-query-the-api/10-query-by-date.md b/docs/02-query-the-api/10-query-by-date.md
new file mode 100644
index 0000000..ed7deaf
--- /dev/null
+++ b/docs/02-query-the-api/10-query-by-date.md
@@ -0,0 +1,48 @@
+# Query by date
+
+This page shows multiple ways to query documents based on a date fields.
+
+Here we use a few predicates that can query based on Date or Timestamp fields. Feel free to explore the [Date & Time based Predicate Reference](../02-query-the-api/02-date-and-time-based-predicate-reference.md) page to learn more about the available Time & Date predicates.
+
+## Query by an exact date
+
+The following is an example that shows how to query for all the documents of the type "article" with the date field ("release_date") equal to January 22, 2020.
+
+The date must be entered as a string as shown below.
+
+> Note that this type of query will only work for the Date Field, not the Timestamp field.
+
+```
+Response response = api.query(
+ Predicates.at("document.type", "article"),
+ Predicates.at("my.article.release_date", "2020-01-22")
+).submit();
+List documents = response.getResults();
+```
+
+## Query by month and year
+
+Here is an example of a query for all documents of the type "blog_post" whose "release_date" field is in the month of May in the year 2020.
+
+```
+Response response = api.query(
+ Predicates.month("my.blog_post.release_date", Predicates.Month.MAY),
+ Predicates.year("my.blog_post.release_date", 2020)
+).submit();
+List documents = response.getResults();
+```
+
+## Query by publication date
+
+You can also query documents by their first or last publication dates.
+
+Here is an example of a query for all documents of the type "blog_post" whose original publication date is in the month of March in the year 2020.
+
+```
+Response response = api.query(
+ Predicates.at("document.type", "blog_post"),
+ Predicates.month("document.first_publication_date", Predicates.Month.MARCH),
+ Predicates.year("document.first_publication_date", 2020)
+).submit();
+List documents = response.getResults();
+```
diff --git a/docs/02-query-the-api/11-query-by-content-relationship.md b/docs/02-query-the-api/11-query-by-content-relationship.md
new file mode 100644
index 0000000..cd91770
--- /dev/null
+++ b/docs/02-query-the-api/11-query-by-content-relationship.md
@@ -0,0 +1,33 @@
+# Query by Content Relationship
+
+To query by a particular Content Relationship field, you must use the ID of the document you are looking for.
+
+> **You must use the document ID**
+>
+> Note that you must use the document ID to make this query. It does not work if you try to query using a UID value.
+
+## By a Content Relationship field
+
+The following example queries all the documents of the type "blog_post" with the Content Relationship field with the API ID of "category_link" equal to the a document with the ID of "WNje3SUAAEGBu8bc".
+
+```
+Response response = api.query(
+ Predicates.at("document.type", "blog_post"),
+ Predicates.at("my.blog_post.category_link", "WNje3SUAAEGBu8bc")
+).submit();
+List documents
+```
+
+## By a Content Relationship field in a Group
+
+If your Content Relationship field is inside a Group, you just need to specify the Group field, then the Content Relationship field.
+
+Here is an example that queries all the documents of the type "blog_post with the Content Relationship field with the API ID of "category_link" equal to the a document with the ID of "WNje3SUAAEGBu8bc". In this case, the Content Relationship field is inside a Group field with the API ID of "categories".
+
+```
+Response response = api.query(
+ Predicates.at("document.type", "blog_post"),
+ Predicates.at("my.blog_post.categories.category_link", "WNje3SUAAEGBu8bc")
+).submit();
+List documents
+```
diff --git a/docs/02-query-the-api/12-fulltext-search.md b/docs/02-query-the-api/12-fulltext-search.md
new file mode 100644
index 0000000..dafffae
--- /dev/null
+++ b/docs/02-query-the-api/12-fulltext-search.md
@@ -0,0 +1,39 @@
+# Fulltext search
+
+You can use the Fulltext predicate to search a document or a specific field for a given term or terms.
+
+The `fulltext` predicate searches the term in any of the following fields:
+
+- Rich Text
+- Title
+- Key Text
+- UID
+- Select
+
+> Note that the fulltext search is not case sensitive.
+
+## Search the entire document
+
+This example shows how to query for all the documents of the type "blog_post" that contain the word "art".
+
+```
+Response response = api.query(
+ Predicates.at("document.type", "blog_post"),
+ Predicates.fulltext("document", "art")
+).submit();
+List documents = response.getResults();
+```
+
+## Search a specific field
+
+The `fulltext` predicate can also be used to search a specific Rich Text, Key Text, UID, or Select field for a given term.
+
+The following example shows how to query for all the documents of the custom type "article" whose "title" field contains the word "galaxy".
+
+```
+Response response = api.query(
+ Predicates.at("document.type", "article"),
+ Predicates.fulltext("my.article.title", "galaxy")
+).submit();
+List documents = response.getResults();
+```
diff --git a/docs/02-query-the-api/13-order-your-results.md b/docs/02-query-the-api/13-order-your-results.md
new file mode 100644
index 0000000..a250944
--- /dev/null
+++ b/docs/02-query-the-api/13-order-your-results.md
@@ -0,0 +1,70 @@
+# Order your results
+
+This page shows how to order the results of a query.
+
+## orderings
+
+The `orderings()` option orders the results by the specified field(s). You can specify as many fields as you want/need.
+
+| Property | Description |
+| --------------------------------------- | ---------------------------------------------------------------------------------------------- |
+| lowest to highest |
It will automatically order the field from lowest to highest
|
+| highest to lowest |
Use "desc" next to the field name to instead order it from greatest to lowest
|
+
+```
+.orderings("my.product.price") // lowest to highest
+.orderings("my.product.price desc") // highest to lowest
+```
+
+### Multiple orderings
+
+You can specify more than one field to order your results. To do so, simply comma-separate the orderings in the string.
+
+The results will be ordered by the first field in the array. If any of the results have the same value for that initial sort, they will then be sorted by the next specified field. And so on.
+
+Here is an example that first sorts the products by price from lowest to highest. If any of the products have the same price, then they will be sorted by their title.
+
+```
+.orderings("my.product.price, my.product.title")
+```
+
+### Sort by publication date
+
+It is also possible to order documents by their first or last publication dates.
+
+| Property | Description |
+| -------------------------------------------- | ------------------------------------------------------------------------------ |
+| first_publication_date |
The date that the document was originally published for the first time
|
+| last_publication_date |
The most recent date that the document has been published after editing
|
+
+```
+.orderings("document.first_publication_date") // first publication date
+.orderings("document.last_publication_date") // last publication date
+```
+
+## after
+
+The `after()` option can be used along with the orderings option. It will remove all the documents except for those after the specified document in the list.
+
+To clarify, let’s say you have a query that return the following documents in this order (these are document IDs):
+
+- `V9Zt3icAAAl8Uzob (Page 1)`
+- `PqZtvCcAALuRUzmO (Page 2)`
+- `VkRmhykAAFA6PoBj (Page 3)`
+- `V4Fs8rDbAAH9Pfow (Page 4)`
+- `G8ZtxQhAALuSix6R (Page 5)`
+- `Ww9yuAvdAhl87wh6 (Page 6)`
+
+If you add the `after()` option and specify page 3, “VkRmhykAAFA6PoBj”, your query will return the following:
+
+- `V4Fs8rDbAAH9Pfow (Page 4)`
+- `G8ZtxQhAALuSix6R (Page 5)`
+- `Ww9yuAvdAhl87wh6 (Page 6)`
+
+By reversing the orderings in your query, you can use this same method to retrieve all the documents before the specified document.
+
+This option is useful when creating a navigation for a blog.
+
+```
+.set("after", "VkRmhykAAFA6PoBj")
+```
diff --git a/docs/02-query-the-api/14-pagination-for-results.md b/docs/02-query-the-api/14-pagination-for-results.md
new file mode 100644
index 0000000..8ad0adc
--- /dev/null
+++ b/docs/02-query-the-api/14-pagination-for-results.md
@@ -0,0 +1,33 @@
+# Pagination for results
+
+The results retrieved from your repository API will automatically be paginated. Here you will find an explanation for how to modify the pagination parameters.
+
+## pageSize
+
+The `pageSize` option defines the maximum number of documents that the API will return for your query.
+
+If left unspecified, the pagination will default to 20. The maximum value allowed is 100.
+
+Here is an example that shows how to query all of the documents of the type "recipe," allowing 100 documents per page.
+
+```
+Response response = api.query(
+ Predicates.at("document.type", "recipe")
+).pageSize(100).submit();
+List documents = response.getResults();
+```
+
+## page
+
+The `page` option defines the pagination for the results of your query. If left unspecified, it will default to 1, which corresponds to the first page.
+
+Here is an example that show how to query all of the documents of the type "recipe". The options entered will limit the results to 30 recipes per page, and will display the third page of results.
+
+```
+Response response = api.query(
+ Predicates.at("document.type", "recipe")
+).pageSize(30)
+.page(3)
+.submit();
+List documents = response.getResults();
+```
diff --git a/docs/02-query-the-api/15-query-by-language.md b/docs/02-query-the-api/15-query-by-language.md
new file mode 100644
index 0000000..a43b809
--- /dev/null
+++ b/docs/02-query-the-api/15-query-by-language.md
@@ -0,0 +1,27 @@
+# Query by language
+
+Here we show how to query documents by their language.
+
+## Query a specific language
+
+You simply need to add the `lang()` query option and set it to the language code you need (example, "en-us" for American English). If you don't specify a "lang" the query will automatically query the documents in your master language.
+
+Here is an example of how to query for all the documents of the type "blog_post" in French (language code "fr-fr").
+
+```
+Response response = api.query(
+ Predicates.at("document.type", "blog_post")
+).lang("fr-fr").submit();
+List documents = response.getResults();
+```
+
+## Query for all languages
+
+If you want to query all the document in all languages you can add the wildcard, `*` as your lang option. This example shows how to query all documents of the type "blog_post" in all languages.
+
+```
+Response response = api.query(
+ Predicates.at("document.type", "blog_post")
+).lang("*").submit();
+List documents = response.getResults();
+```
diff --git a/docs/03-templating/01-the-document-object.md b/docs/03-templating/01-the-document-object.md
new file mode 100644
index 0000000..e3e6295
--- /dev/null
+++ b/docs/03-templating/01-the-document-object.md
@@ -0,0 +1,80 @@
+# The Document object
+
+Here we will discuss the document object when using the Java development kit.
+
+> **Before Reading**
+>
+> This article assumes that you have queried your API and saved the document object in a variable named `document`.
+
+## Accessing Document Fields
+
+Here is how to access a document's information fields.
+
+### ID
+
+```
+document.getId()
+```
+
+### UID
+
+```
+document.getUid()
+```
+
+### Type
+
+```
+document.getType()
+```
+
+### API URL
+
+```
+document.getHref()
+```
+
+### Tags
+
+```
+document.getTags()
+// returns a Set
+```
+
+### First Publication Date
+
+```
+document.getFirstPublicationDate()
+```
+
+### Last Publication Date
+
+```
+document.getLastPublicationDate()
+```
+
+### Language
+
+```
+document.getLang()
+```
+
+### Alternate Language Versions
+
+```
+document.getAlternateLanguages()
+// returns a list
+```
+
+You can read more about this in the [Multi-language page.](../03-templating/11-multi-language-info.md)
+
+## Document Content
+
+To retrieve the content fields from the document you must specify the API ID of the field. Here is an example that retrieves a Date field's content from the document. Here the Date field has the API ID of `date`.
+
+```
+// Assuming the document is of the type "page"
+document.getDate("page.date").getValue()
+```
+
+Refer to the specific templating documentation for each field to learn how to add content fields to your pages.
diff --git a/docs/03-templating/02-boolean.md b/docs/03-templating/02-boolean.md
new file mode 100644
index 0000000..09562e5
--- /dev/null
+++ b/docs/03-templating/02-boolean.md
@@ -0,0 +1,5 @@
+# Templating the Boolean field
+
+The Boolean field will add a switch for a true or false values for the content authors to pick from.
+
+## We don't yet support the Boolean field for Java
diff --git a/docs/03-templating/03-color.md b/docs/03-templating/03-color.md
new file mode 100644
index 0000000..140a3d8
--- /dev/null
+++ b/docs/03-templating/03-color.md
@@ -0,0 +1,12 @@
+# Templating the Color field
+
+The Color field allows content writers to select a color through a variety of color pickers as well as having the option to manually input a hex value.
+
+## Get the hex color value
+
+Here's how to get the hex value of a Color field using the `getHexValue()` method.
+
+```
+
+
Colorful Title
+```
diff --git a/docs/03-templating/04-date.md b/docs/03-templating/04-date.md
new file mode 100644
index 0000000..5724626
--- /dev/null
+++ b/docs/03-templating/04-date.md
@@ -0,0 +1,27 @@
+# Templating the Date field
+
+The Date field allows content writers to add a date that represents a calendar day.
+
+## Get & output the date value
+
+Here's how to get the value of a Date field and output it in a simple format.
+
+```
+
+ ${document.getDate("post.date").getValue()}
+
+// Outputs in the following format: 2016-01-23
+```
+
+## Other date formats
+
+You can control the format of the date value by using `asText()` method and passing in the formatting options as shown below.
+
+```
+
+ ${document.getDate("post.date").asText("EEEE MMMM dd, yyyy")}
+
+// Outputs in the following format: Saturday January 23, 2016
+```
+
+For more formatting options, explore the [Java documentation for date formatting options](https://docs.oracle.com/javase/8/docs/api/java/time/format/DateTimeFormatter.html#patterns).
diff --git a/docs/03-templating/05-embed.md b/docs/03-templating/05-embed.md
new file mode 100644
index 0000000..ce79683
--- /dev/null
+++ b/docs/03-templating/05-embed.md
@@ -0,0 +1,29 @@
+# Templating the Embed field
+
+The Embed field will let content authors paste an oEmbed supported service resource URL (YouTube, Vimeo, Soundcloud, etc.), and add the embeded content to your website.
+
+## Display as HTML
+
+Here's an example of how to integrate the Embed field into your templates using the `asHtml()` method.
+
+```
+
${document.getEmbed("page.embed").asHtml()}
+```
+
+## Get the Embed type
+
+The following shows how to retrieve the Embed type from an Embed field using the `getType()` method.
+
+```
+document.getEmbed("page.embed").getType()
+// For example this might return: video
+```
+
+## Get the Embed provider
+
+The following shows how to retrieve the Embed provider from an Embed field using the `getProvider()` method.
+
+```
+document.getEmbed("page.embed").getProvider()
+// For example this might return: YouTube
+```
diff --git a/docs/03-templating/06-geopoint.md b/docs/03-templating/06-geopoint.md
new file mode 100644
index 0000000..3cb670f
--- /dev/null
+++ b/docs/03-templating/06-geopoint.md
@@ -0,0 +1,12 @@
+# Templating the GeoPoint Field
+
+The GeoPoint field is used for Geolocation coordinates. It works by adding coordinates or by pasting a Google Maps URL.
+
+## Get the latitude and longitude values
+
+Here's an example of how to retrieve the latitude the longitude values from the GeoPoint field using the `getLatitude()` and `getLongitude()` methods.
+
+```
+
+
+```
diff --git a/docs/03-templating/07-group.md b/docs/03-templating/07-group.md
new file mode 100644
index 0000000..ce567d4
--- /dev/null
+++ b/docs/03-templating/07-group.md
@@ -0,0 +1,58 @@
+# Templating the Group Field
+
+The Group field is used to create a repeatable collection of fields.
+
+## Repeatable Group
+
+### Looping through the Group content
+
+To integrate a Group field into your templates, loop through each item in the group as shown in the following example.
+
+```
+
+```
+
+### Another Example
+
+Here's another example that shows how to integrate a group of images (e.g. a photo gallery) into a page.
+
+```
+
+
+
+
+
+ ${photo.getText("caption")}
+
+
+
+
+```
+
+## Non-repeatable Group
+
+Even if the group is non-repeatable, the Group field will be an array. You simply need to get the first (and only) group in the array and you can retrieve the fields in the group like any other.
+
+Here is an example showing how to integrate the fields of a non-repeatable Group into your templates.
+
+```
+
+
+
+
+
+
+
+```
diff --git a/docs/03-templating/08-image.md b/docs/03-templating/08-image.md
new file mode 100644
index 0000000..e88bcf9
--- /dev/null
+++ b/docs/03-templating/08-image.md
@@ -0,0 +1,94 @@
+# Templating the Image field
+
+The Image field allows content writers to upload an image that can be configured with size constraints and responsive image views.
+
+## Get the image url
+
+The easiest way to integrate an image is to retrieve and add the image url to an image element.
+
+The following integrates a page's illustration image field.
+
+```
+
+```
+
+## Output as HTML
+
+The `asHtml()` method will convert and output the Image as an HTML image element.
+
+```
+${document.getImage("page.featured_image").asHtml(linkResolver)}
+```
+
+## Get a responsive image view
+
+The `getView()` method allows you to retrieve and use your responsive image views. Simply pass the name of the view into the method and use it like any other image fragment.
+
+Here is how to add responsive images using the HTML picture element.
+
+```
+
+
+
+
+
+
+
+
+
+
+```
+
+## Add alt or copyright text to your image
+
+### The main image
+
+If you added an alt or copyright text value to your image, you retrieve and apply it as follows using the `getAlt()` and `getCopyright()` methods.
+
+```
+
+
+
+
+
+```
+
+### An image view
+
+Here's how to retrieve the alt or copyright text for a responsive image view. Note that the alt and copyright text will be the same for all views.
+
+```
+
+
+
+
+
+
+```
+
+## Get the image width & height
+
+### The main image
+
+You can retrieve the main image's width or height by using the `getWidth()` and `getHeight()` methods as shown below.
+
+```
+
+
+
+
+
+```
+
+### An image view
+
+Here is how to retrieve the width and height for a responsive image view.
+
+```
+
+
+
+
+
+
+```
diff --git a/docs/03-templating/09-key-text.md b/docs/03-templating/09-key-text.md
new file mode 100644
index 0000000..2c6f5ff
--- /dev/null
+++ b/docs/03-templating/09-key-text.md
@@ -0,0 +1,11 @@
+# Templating the Key Text field
+
+The Key Text field allows content writers to enter a single string.
+
+## Get the text value
+
+Here's an example that shows how to retrieve the Key Text value and output the string into your template using the `getText()` method.
+
+```
+
${document.getText("blog_post.title")}
+```
diff --git a/docs/03-templating/10-link-content-relationship.md b/docs/03-templating/10-link-content-relationship.md
new file mode 100644
index 0000000..dc47cd9
--- /dev/null
+++ b/docs/03-templating/10-link-content-relationship.md
@@ -0,0 +1,46 @@
+# Templating Links & Content Relationship fields
+
+The Link field is used for adding links to the web, to documents in your prismic.io repository, or to files in your prismic.io media library. The Content Relationship field is a Link field specifically used to link to a Document.
+
+## Link to the Web
+
+Here's the basic integration of a Link to the Web into your templates. In this example, the Link field has the API ID of `external_link`.
+
+```
+
+
+
+
+ Open in a new tab
+
+
+ Click here
+
+
+```
+
+Note that the example above uses a Link Resolver. If your link field has been set up so that it can only take a Link to the Web, then this is not needed. You only need a Link Resolver if the Link field might contain a Link to a Document.
+
+## Link to a Document / Content Relationship
+
+When integrating a Link to a Document in your repository, a Link Resolver is necessary as shown below.
+
+In the following example the Content Relationship (Link to a Document) field has the API ID of `document_link`.
+
+```
+
+Go to page
+```
+
+Note that the example above uses a Link Resolver. A Link Resolver is required when retrieving the url for a Link to a Document.
+
+## Link to a Media Item
+
+The following shows how to retrieve the url for a Link to a Media Item. In this example, the Link field has the API ID of `media_link`.
+
+```
+
+View Image
+```
+
+Note that the example above uses a Link Resolver. If your link field has been set up so that it can only link to a Media Item, then this is not needed. You only need a Link Resolver if the Link field might contain a Link to a Document.
diff --git a/docs/03-templating/11-multi-language-info.md b/docs/03-templating/11-multi-language-info.md
new file mode 100644
index 0000000..7ac8eb4
--- /dev/null
+++ b/docs/03-templating/11-multi-language-info.md
@@ -0,0 +1,24 @@
+# Templating Multi-language info
+
+This page shows you how to access the language code and alternate language versions of a document.
+
+## Get the document language code
+
+Here is how to access the language code of a document queried from your prismic.io repository. This might give "en-us" (American english), for example.
+
+```
+document.getLang()
+```
+
+## Get the alternate language versions
+
+Next we will access the information about a document's alternate language versions. You can retrieve them using the `getAlternateLanguages()` method and loop through the List to access the id, uid, type, and language code of each as shown below.
+
+```
+
+ id: ${altLang.getId()}
+ uid: ${altLang.getUid()}
+ Type: ${altLang.getType()}
+ Lang: ${altLang.getLang()}
+
+```
diff --git a/docs/03-templating/12-number.md b/docs/03-templating/12-number.md
new file mode 100644
index 0000000..19fad41
--- /dev/null
+++ b/docs/03-templating/12-number.md
@@ -0,0 +1,13 @@
+# Templating the Number field
+
+The Number field allows content writers to enter or select a number. You can set set max and min values for the number.
+
+## Get the number value
+
+Here is an example of how to retrieve the value of a Number field and insert it into your template using the `getValue()` method.
+
+```
+
+ ${document.getNumber("article.statistic").getValue()}
+
+```
diff --git a/docs/03-templating/13-rich-text-and-title.md b/docs/03-templating/13-rich-text-and-title.md
new file mode 100644
index 0000000..5b62a60
--- /dev/null
+++ b/docs/03-templating/13-rich-text-and-title.md
@@ -0,0 +1,102 @@
+# Templating the Rich Text & Title fields
+
+The Rich Text field is a configurable text field with formatting options. This field provides content writers with a WYSIWYG editor where they can define the text as a header or paragraph, make it bold, add links, etc.
+
+The Title field is a specific Rich Text field used for titles.
+
+## Output as HTML
+
+The basic usage of the Rich Text / Title field is to use the `asHtml()` method to transform the field into HTML code.
+
+The following is an example that would display the title of a blog post.
+
+```
+${document.getStructuredText("blog_post.title").asHtml(linkResolver)}
+```
+
+In the previous example when calling the `asHtml()` method, you need to pass in a Link Resolver function. A Link Resolver is a function that determines what the url of a document link will be.
+
+### Example 2
+
+The following example shows how to display the rich text body content of a blog post.
+
+```java
+${document.getStructuredText("blog_post.body").asHtml(linkResolver)}
+```
+
+### Changing the HTML Output
+
+You can customize the HTML output by passing an HTML serializer to the method as shown below.
+
+This example will edit how an image in a Rich Text field is displayed while leaving all the other elements in their default output.
+
+```java
+// In your controller
+HtmlSerializer serializer = new HtmlSerializer() {
+ @Override
+ public String serialize(Fragment.StructuredText.Element element, String content) {
+ if (element instanceof Fragment.StructuredText.Block.Image) {
+ Fragment.StructuredText.Block.Image image = (Fragment.StructuredText.Block.Image)element;
+ return (image.getView().asHtml(linkResolver));
+ }
+ return null;
+ }
+};
+
+// In JSP
+${doc.getStructuredText("blog_post.body").asHtml(linkResolver, serializer)}
+```
+
+## Output as plain text
+
+The `getText()` method will convert and output the text in the Rich Text / Title field as a string. You need to specify which text block you wish to output.
+
+### Get the first Rich Text block
+
+In the example below we use the `getBlocks()` method to get all the blocks then the `get()` select the first block. Note you will need to do this even if there is only one block.
+
+```
+
+```
+
+### Get the first heading
+
+The `getTitle()` method will find the first heading block in the Rich Text field.
+
+Here's an example of how to integrate this.
+
+```
+
+```
+
+### Get the first paragraph
+
+The `getFirstParagraph()` method will find the first paragraph block in the Rich Text field.
+
+Here's an example of how to integrate this.
+
+```
+
+```
+
+### Get the first preformatted block
+
+The `getFirstPreformatted()` method will find the first preformatted block in the Rich Text field.
+
+Here's an example of how to integrate this.
+
+```
+
+```
+
+### Get the first image
+
+The `getFirstImage()` method will find the first image block in the Rich Text field.
+
+You can then use the `getUrl()` method to display the image as shown here.
+
+```
+
+```
diff --git a/docs/03-templating/14-select.md b/docs/03-templating/14-select.md
new file mode 100644
index 0000000..40e95a1
--- /dev/null
+++ b/docs/03-templating/14-select.md
@@ -0,0 +1,13 @@
+# Templating the Select field
+
+The Select field will add a dropdown select box of choices for the content authors to pick from.
+
+## Get the Select field text value
+
+The following example shows how to retrieve the value of a Select field using the `getText()` method.
+
+```
+
+ ${document.getText("article.category")}
+
+```
diff --git a/docs/03-templating/15-slices.md b/docs/03-templating/15-slices.md
new file mode 100644
index 0000000..358a567
--- /dev/null
+++ b/docs/03-templating/15-slices.md
@@ -0,0 +1,142 @@
+# Templating Slices
+
+The Slices field is used to define a dynamic zone for richer page layouts.
+
+## Example 1
+
+You can retrieve Slices from your documents by using the `getSliceZone()` method.
+
+Here is a simple example that shows how to add slices to your templates. In this example, we have two slice options: a text slice and an image gallery slice.
+
+### Text slice
+
+The "text" slice is simple and only contains one field, which is non-repeatable.
+
+| Property | Description |
+| ------------------------------------ | ------------------------------------------------------------------- |
+| non-repeatable |
- A Rich Text field with the API ID of "rich_text"
+```
+
+## Example 2
+
+The following is a more advanced example that shows how to use Slices for a landing page. In this example, the Slice choices are FAQ question/answers, featured items, and text sections.
+
+### FAQ slice
+
+The "faq" slice takes advantage of both the repeatable and non-repeatable slice sections.
+
+| Property | Description |
+| ------------------------------------ | ------------------------------------------------------------------------------------------------------------------------------ |
+| non-repeatable |
- A Title field with the API ID of "faq_title"
|
+| repeatable |
- A Title field with the API ID of "question"
- A Rich Text field with the API ID of "answer"
|
+
+### Featured Items slice
+
+The "featured_items" slice contains a repeatable set of an image, title, and summary fields.
+
+| Property | Description |
+| ------------------------------------ | ---------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- |
+| non-repeatable |
None
|
+| repeatable |
- An Image field with the API ID of "image"
- A Title field with the API ID of "title"
- A Rich Text field with the API ID of "summary"
|
+
+### Text slice
+
+The "text" slice contains only a Rich Text field in the non-repeatable section.
+
+| Property | Description |
+| ------------------------------------ | ------------------------------------------------------------------- |
+| non-repeatable |
- A Rich Text field with the API ID of "rich_text"
|
+| repeatable |
None
|
+
+### Integration
+
+Here is an example of how to integrate these slices into a landing page.
+
+```
+
+```
diff --git a/docs/03-templating/16-timestamp.md b/docs/03-templating/16-timestamp.md
new file mode 100644
index 0000000..cecdf7a
--- /dev/null
+++ b/docs/03-templating/16-timestamp.md
@@ -0,0 +1,27 @@
+# Templating the Timestamp field
+
+The Timestamp field allows content writers to add a date and time.
+
+## Get & output the timestamp value
+
+Here's how to get the value of a Timestamp field and output it in a simple format.
+
+```
+
+ ${document.getTimestamp("event.date").getValue()}
+
+// Outputs in the following format: 2016-01-23T13:30:00.000+00:00
+```
+
+## Other date & time formats
+
+You can control the format of the Timestamp value by using `asText()` method and passing in the formatting options as shown below.
+
+```
+
+ ${document.getTimestamp("event.date").asText("hh:mm a - EE MMM dd")}
+
+// Outputs in the following format: 01:30 PM - Sat Jan 23
+```
+
+For more formatting options, explore the [Java documentation for date formatting options](https://docs.oracle.com/javase/8/docs/api/java/time/format/DateTimeFormatter.html#patterns).
diff --git a/docs/03-templating/17-uid.md b/docs/03-templating/17-uid.md
new file mode 100644
index 0000000..0c874df
--- /dev/null
+++ b/docs/03-templating/17-uid.md
@@ -0,0 +1,11 @@
+# Templating the UID field
+
+The UID field is a unique identifier that can be used specifically to create SEO-friendly website URLs.
+
+## Get the UID Value
+
+You can get the UID value from the retrieved document object by using the `getUid` method.
+
+```
+document.getUid()
+```
diff --git a/docs/04-misc-topics/01-link-resolver.md b/docs/04-misc-topics/01-link-resolver.md
new file mode 100644
index 0000000..e13143b
--- /dev/null
+++ b/docs/04-misc-topics/01-link-resolver.md
@@ -0,0 +1,51 @@
+# Link Resolver
+
+When working with field types such as [Link](../03-templating/10-link-content-relationship.md) or [Rich Text](../03-templating/13-rich-text-and-title.md), the prismic.io Java kit will need to generate links to documents within your website.
+
+> **Before Reading**
+>
+> Since routing is specific to your site, you will need to define your Link Resolver and provide it to some of the methods used on the fields.
+
+## Adding the Link Resolver
+
+If you are incorporating prismic.io into your existing Java project or using one of the Java starter projects, you will need to create a Link Resolver.
+
+Here is an example that shows how to add a simple Link Resolver in your controller.
+
+```
+public static LinkResolver linkResolver = new LinkResolver(){
+ public String resolve(Fragment.DocumentLink link) {
+
+ // Get the link type
+ String type = link.getType();
+
+ // URL for the category type
+ if (type.equals("category")) {
+ return "/category/" + link.getUid();
+ }
+
+ // URL for the product type
+ if (type.equals("product")) {
+ return "/product/" + link.getId();
+ }
+
+ // Default case for all other types
+ return "/";
+ }
+};
+```
+
+A Link Resolver is provided in the [Java Spring MVC starter project](https://github.com/prismicio/java-springmvc-starter), but you may need to adapt it or write your own depending on how you've built your website app.
+
+## Accessible attributes
+
+When creating your link resolver function, you will have access to certain attributes of the linked document.
+
+| Property | Description |
+| --------------------------------------------------------- | ------------------------------------------------------- |
+| link.getId() string |
The document id
|
+| link.getUid() string |
The user-friendly unique id
|
+| link.getType() string |
The custom type of the document
|
+| link.getTags() array |
Array of the document tags
|
+| link.getLang() string |
The language code of the document
|
+| link.isBroken() boolean |
Boolean that states if the link is broken or not
|
diff --git a/docs/04-misc-topics/02-previews-and-the-prismic-toolbar.md b/docs/04-misc-topics/02-previews-and-the-prismic-toolbar.md
new file mode 100644
index 0000000..92827e5
--- /dev/null
+++ b/docs/04-misc-topics/02-previews-and-the-prismic-toolbar.md
@@ -0,0 +1,90 @@
+# Previews & the Prismic Toolbar
+
+When working in the writing room, you can preview new content on your website without having to publish the document, you can set up one or more websites where the content can be previewed. This allows you to have a production server, staging server, development server, etc. Discover how to [handle multiple environments in Prismic](https://intercom.help/prismicio/prismic-io-basics/using-multiple-environments-of-one-prismic-repository).
+The Toolbar allows you to edit your pages. In your website, just click the button, select the part of the page that they want to edit, and you'll be redirected to the appropriate page in the Writing Room.
+
+> **Preview Activation for Older Repos**
+>
+> The current preview toolbar is enabled by default on all new Prismic repos. However, if you're on an older repo, you might be on older version of the toolbar. If your preview script `src` URL (described in Step 2, below) includes `new=true`, then your preview functionality includes an edit button. If the `src` does not include `new=true` and you would like the new preview toolbar, please [contact us via the Prismic forum](https://community.prismic.io/t/feature-activations-graphql-integration-fields-etc/847) so we can activate it for you.
+
+## 1. Configure Previews
+
+In your repository, navigate to **Settings > Previews**. Here you can add the configuration for a new preview, just add:
+
+- **The Site Name**: The name of the current site your configuring.
+- **Domain URL**: You can add the URL of your live website or a localhost domain, such as: http://localhost:8000.
+- **Link Resolver (optional) **: In order to be taken directly to the page you are previewing instead of the homepage, add a Link Resolver which is an endpoint on your server that takes a Prismic document and returns the url for that document. More details on step _3. Add a Link Resolver endpoint._
+
+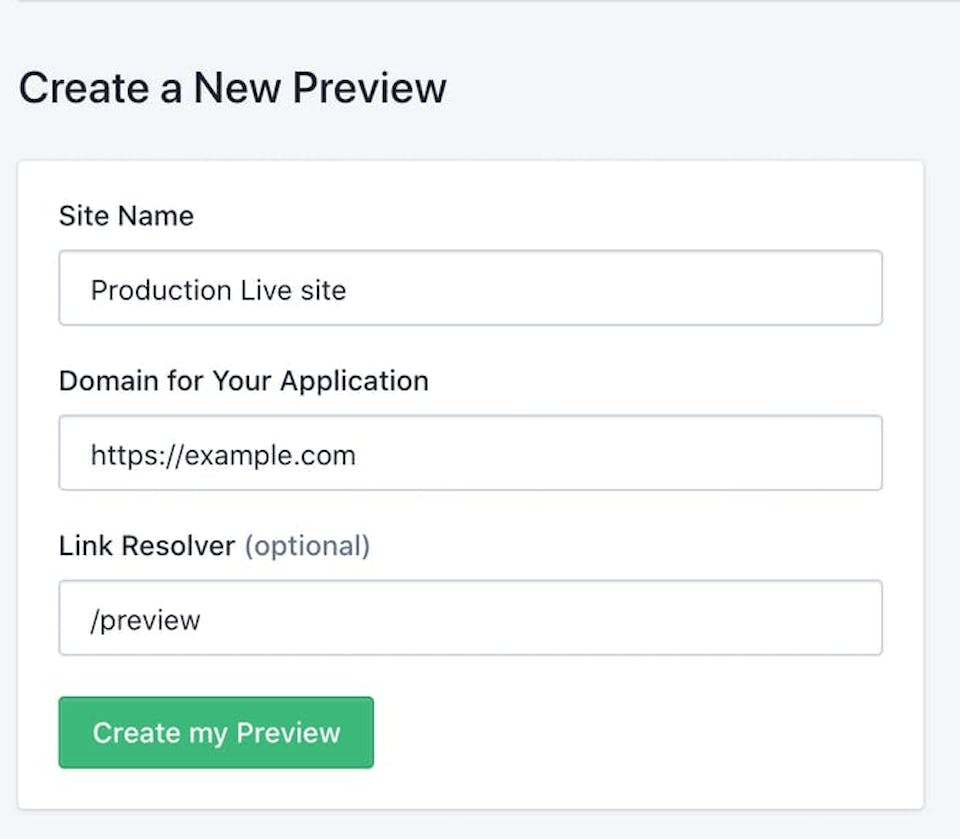
+
+## 2. Include the Prismic Toolbar javascript file
+
+You will need to include the Prismic toolbar script **on every page of your website including your 404 page.**
+
+You can find the correct script in your repository **Settings** section, under the **Previews** tab.
+
+**Settings > Previews > Script**
+
+```
+