-
Notifications
You must be signed in to change notification settings - Fork 0
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
1 parent
5a1f6cc
commit fa84b16
Showing
1 changed file
with
119 additions
and
2 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,2 +1,119 @@ | ||
# devonChurch | ||
eggs-benedict | ||
# Eggs Benedict 🥚🥓🍞🍽 | ||
|
||
## What 👋 | ||
|
||
A simple utility that limits the impact of functions the demand high CPU load. | ||
|
||
## Why 🤷♀️ | ||
|
||
Functionality that demands intense CPU load can create a poor user experience as their session can hang and become unresponsive. | ||
|
||
There are _**throttle**_ and _**debounce**_ solutions that help to alleviate the repercussions of CPU intensive UI. _Eggs Benedict_ incorporates these methodologies into a light weight _**React**_ and _**Vanilla JS**_ abstraction. | ||
|
||
--- | ||
|
||
_Eggs Benedict_ was original designed to help with the various complex calculations associated with the [Avocado](https://github.com/devonChurch/avocado) application. The library has since been enhanced into a simple developer API that can yield some powerful results. | ||
|
||
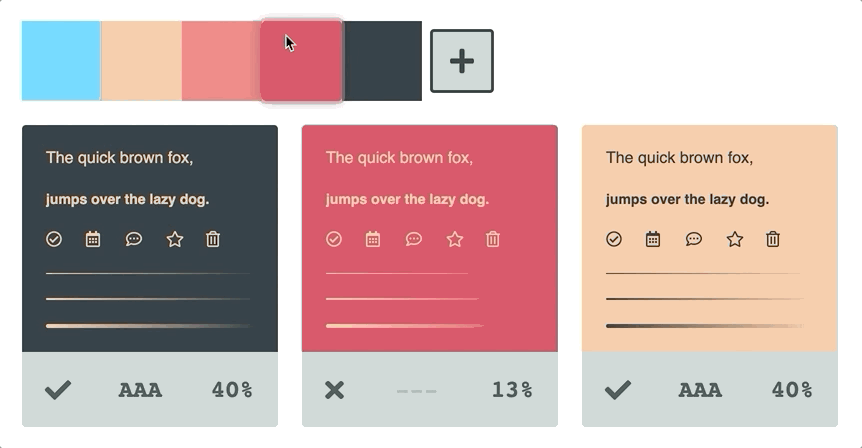 | ||
|
||
## How 💡 | ||
|
||
This library uses [Request Animation Frame](https://developer.mozilla.org/en-US/docs/Web/API/window/requestAnimationFrame) to implement _**throttle**_ and _**debounce**_ solutions simultaneously. This provides the real time updates of a _throttler_ while ensuring that the UI does not fall out of sync with a _debouncer_. | ||
|
||
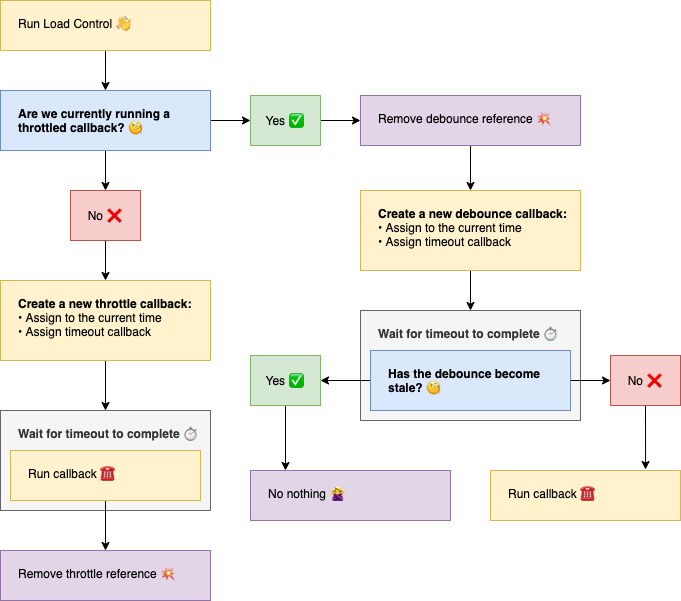 | ||
|
||
## Options ⚙️ | ||
|
||
_Eggs Benedict_ offers some light configuration for custom _**throttle**_ and _**debounce**_ delays. | ||
|
||
``` | ||
{ | ||
throttleDelay: 0, // Default. | ||
debounceDelay: 100 // Default. | ||
} | ||
``` | ||
|
||
Take a look at the [interactive CodeSandbox](https://codesandbox.io/s/eager-torvalds-th3lz) to see configuration examples and their correlation with different CPU loads. | ||
|
||
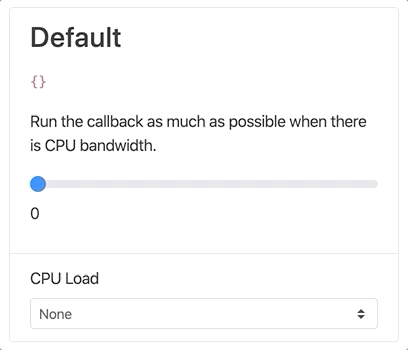 | ||
|
||
## Typescript 👌 | ||
|
||
_...TBC_ | ||
|
||
## Examples 📝 | ||
|
||
### React Hooks | ||
|
||
[In this example](https://codesandbox.io/s/determined-khayyam-ku303) we are using the _**React Hooks**_ import to run some CPU heavy work when the _Range_ `<input />` changes its value. | ||
|
||
```javascript | ||
import React from "react"; | ||
import { useLoadControl } from "eggs-benedict/hooks"; | ||
|
||
export default function App() { | ||
const [count, setCount] = React.useState(0); | ||
const heavyCpuLoad = (value) => { | ||
/** | ||
* CPU HEAVY WORK HERE! | ||
* - - - - - - - - - - - | ||
* Maybe some complex calculations based on the Range <input /> value 🤓 | ||
*/ | ||
setCount(value); | ||
}; | ||
const setLoadControlCount = useLoadControl(heavyCpuLoad); | ||
const handleChange = (event) => | ||
setLoadControlCount(event.currentTarget.value); | ||
|
||
return ( | ||
<> | ||
<input type="range" value={count} onChange={handleChange} /> | ||
<p>{count}</p> | ||
</> | ||
); | ||
} | ||
``` | ||
|
||
### Vanilla JS | ||
|
||
[In this example](https://codesandbox.io/s/hidden-fast-bsvpe) we are using a _**Vanilla JS**_ implementation inside a _**React**_ `useEffect` scaffold. When the user scrolls the `window` we run a CPU heavy callback. | ||
|
||
```javascript | ||
import React from "react"; | ||
import { LoadControl } from "eggs-benedict"; | ||
|
||
export default function App() { | ||
const [scroll, setScroll] = React.useState(0); | ||
React.useEffect(() => { | ||
const heavyCpuLoad = (event) => { | ||
/** | ||
* CPU HEAVY WORK HERE! | ||
* - - - - - - - - - - - | ||
* Maybe slow DOM heavy math to move some elements around 🤓 | ||
*/ | ||
setScroll(event.currentTarget.scrollY); | ||
}; | ||
const [scrollLoadControl, cleanUpScrollLoadControl] = LoadControl( | ||
heavyCpuLoad | ||
); | ||
window.addEventListener("scroll", scrollLoadControl); | ||
|
||
/** | ||
* Remember to remove the Eggs Benedict instance when unxmounting your | ||
* <Component /> 👍 | ||
*/ | ||
return cleanUpScrollLoadControl; | ||
}, []); | ||
return ( | ||
<> | ||
<p style={{ position: "fixed" }}>{scroll}</p> | ||
<div | ||
style={{ | ||
height: "200vh", | ||
backgroundImage: "linear-gradient(to bottom, #58FFC7, #2D8165)", | ||
}} | ||
/> | ||
</> | ||
); | ||
} | ||
``` |