-
Notifications
You must be signed in to change notification settings - Fork 0
2. HTML
Deepak Ramalingam edited this page Feb 18, 2023
·
7 revisions
We will use HTML to layout the skeleton of the pages of our website.
HTML, also known as Hypertext Markup Language is a markup language that allows us to define the structure of our webpages. Basically, we can define what should be on the page (headers, paragraphs, input fields, buttons, etc.)
Good resource to learn HTML: https://www.w3schools.com/html/default.asp
Our application will feature 2 pages: a page to view movie ratings and a page to rate a movie.
Thus, we will create three new files:
-
index.html
for our page to view movie ratings -
rate-movie.html
for our page to rate a movie
<!DOCTYPE html>
<html>
<head>
<!-- Title of Webpage (appears in tab name) -->
<title>Spicy Tomatoes</title>
</head>
<body>
<!-- Navbar -->
<div>
<img width="50px" height="50px" src="https://d35lsekltq0ycz.cloudfront.net/web-arcade/tomato-transparent.png"/>
<h1>Spicy Tomatoes</h1>
</div>
<!-- Write Review Button -->
<a href="rate-movie.html">Review Movie</a>
<!-- List of Ratings for Movies -->
<div>
<!-- Example Movie Rating -->
<div>
<p>Movie Name 1</p>
<p>Review 1</p>
</div>
<!-- Example Movie Rating -->
<div>
<p>Movie Name 2</p>
<p>Review 2</p>
</div>
<!-- Example Movie Rating -->
<div>
<p>Movie Name 3</p>
<p>Review 3</p>
</div>
</div>
</body>
</html>
Notes:
- The "head" tag defines metadata for the website (stuff that appears in places like the preview of our website in a Google search result)
- The "body" tag defines the actual content of our website
- The "img" tag is for drawing an image referenced by the "src" attribute
- The "h1" tag is for header-styled text
- The "Navbar" does not look like a Navbar yet because we have to add styling to it (using CSS)
- We can use "div" tags to encapsulate elements together which will be useful when we add stylings later
- The "a" tag is a link and the "href" attribute of the tag allows us to define the page to go to when clicking on the link
- The list of movies is hardcoded, but later we will use JavaScript to query them from a database via a third-party API
<!DOCTYPE html>
<html>
<head>
<!-- Title of Webpage (appears in tab name) -->
<title>Spicy Tomatoes</title>
</head>
<body>
<!-- Navbar -->
<div>
<img width="50px" height="50px" src="https://d35lsekltq0ycz.cloudfront.net/web-arcade/tomato-transparent.png"/>
<h1>Spicy Tomatoes</h1>
</div>
<!-- Form for Typing and Submitting Review -->
<div>
<input placeholder="movie name" type="text"/>
<input placeholder="review" type="text"/>
<button>Submit</button>
</div>
<!-- Continue to Ratings Page Button -->
<a href="index.html">Back</a>
</body>
</html>
Notes:
- The input fields are used for inputting text
- The button can call a function later to create a new movie review in our database by invoking a third-party API
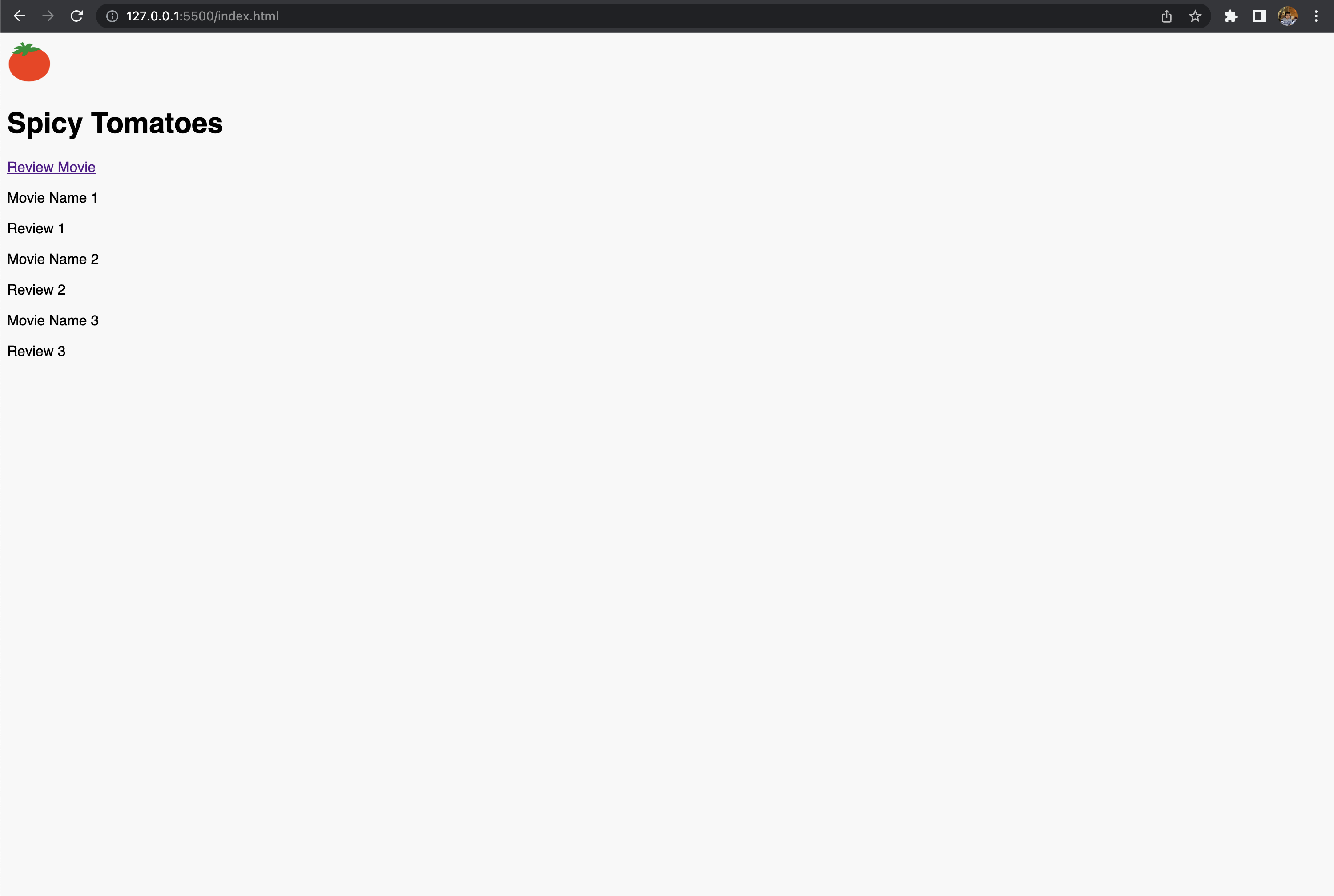
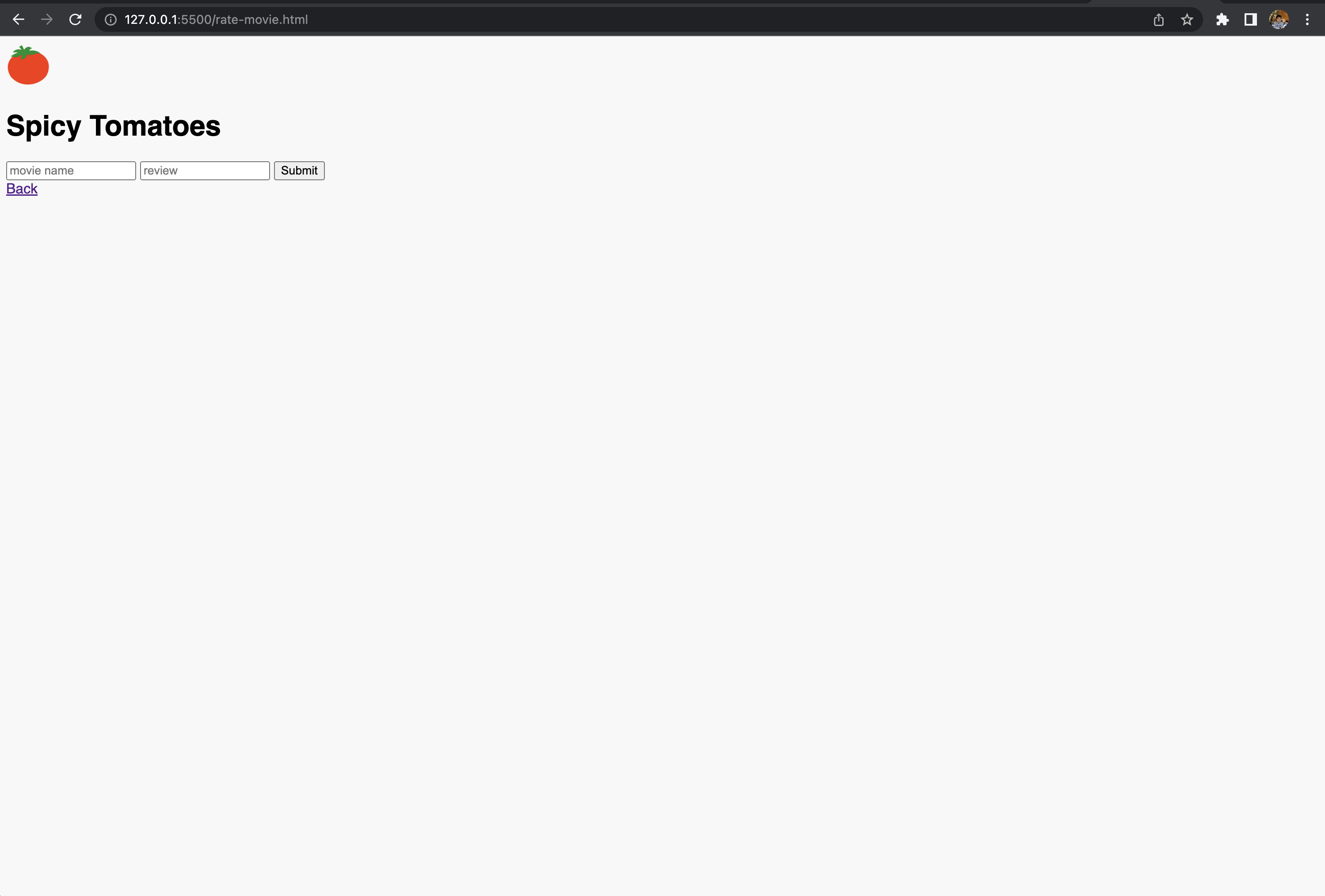