forked from bevyengine/bevy
-
Notifications
You must be signed in to change notification settings - Fork 1
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
Add
RenderTarget::TextureView
(bevyengine#8042)
# Objective We can currently set `camera.target` to either an `Image` or `Window`. For OpenXR & WebXR we need to be able to render to a `TextureView`. This partially addresses bevyengine#115 as with the addition we can create internal and external xr crates. ## Solution A `TextureView` item is added to the `RenderTarget` enum. It holds an id which is looked up by a `ManualTextureViews` resource, much like how `Assets<Image>` works. I believe this approach was first used by @kcking in their [xr fork](https://github.com/kcking/bevy/blob/eb39afd51bcbab38de6efbeeb0646e01e2ce4766/crates/bevy_render/src/camera/camera.rs#L322). The only change is that a `u32` is used to index the textures as `FromReflect` does not support `uuid` and I don't know how to implement that. --- ## Changelog ### Added Render: Added `RenderTarget::TextureView` as a `camera.target` option, enabling rendering directly to a `TextureView`. ## Migration Guide References to the `RenderTarget` enum will need to handle the additional field, ie in `match` statements. --- ## Comments - The [wgpu work](gfx-rs/wgpu@c039a74) done by @expenses allows us to create framebuffer texture views from `wgpu v0.15, bevy 0.10`. - I got the WebXR techniques from the [xr fork](https://github.com/dekuraan/xr-bevy) by @dekuraan. - I have tested this with a wip [external webxr crate](https://github.com/mrchantey/forky/blob/018e22bb06b7542419db95f5332c7684931c9c95/crates/bevy_webxr/src/bevy_utils/xr_render.rs#L50) on an Oculus Quest 2. 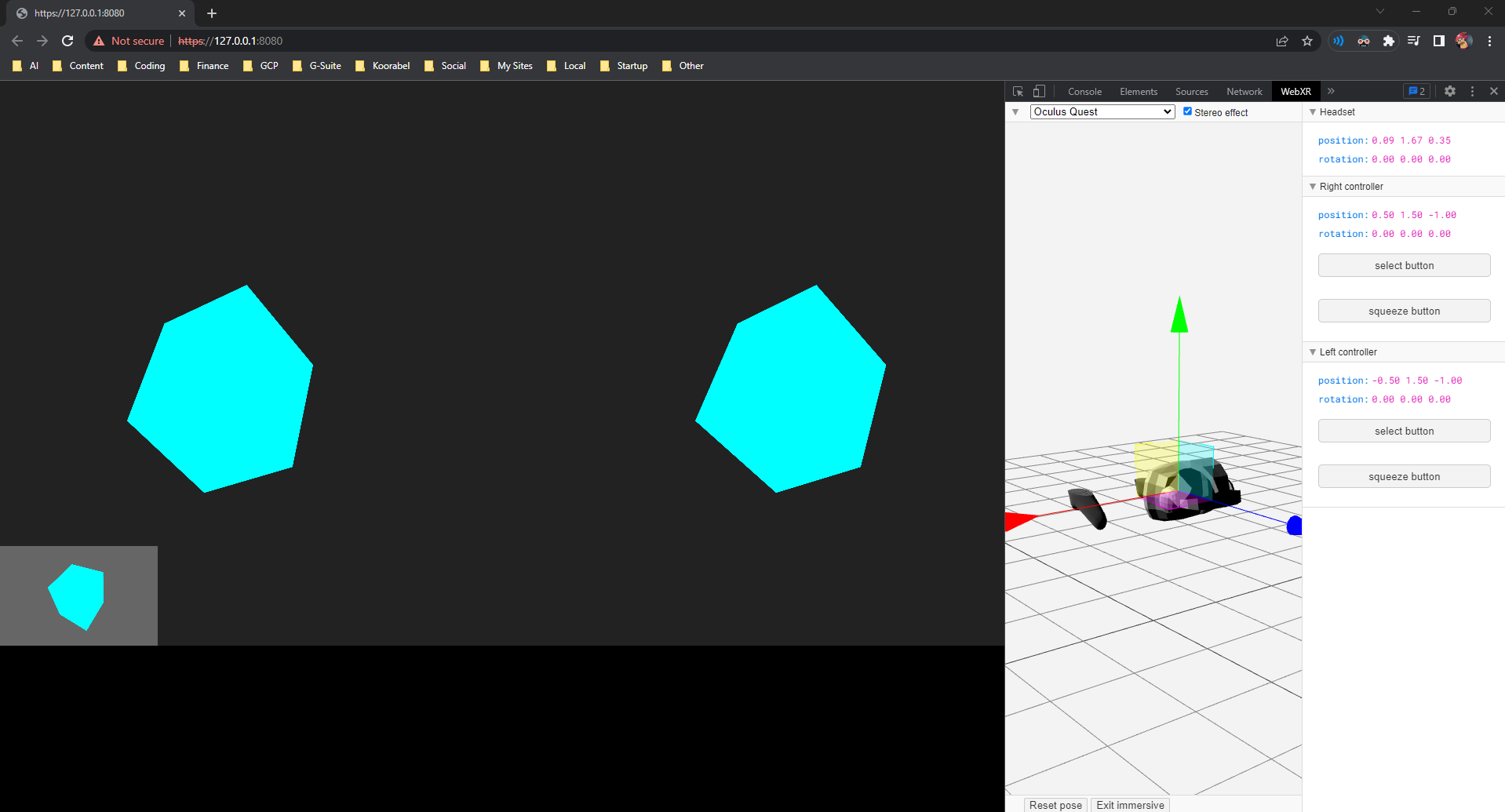 --------- Co-authored-by: Carter Anderson <[email protected]> Co-authored-by: Paul Hansen <[email protected]>
- Loading branch information
Showing
4 changed files
with
108 additions
and
8 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,64 @@ | ||
use crate::extract_resource::ExtractResource; | ||
use crate::render_resource::TextureView; | ||
use crate::texture::BevyDefault; | ||
use bevy_ecs::system::Resource; | ||
use bevy_ecs::{prelude::Component, reflect::ReflectComponent}; | ||
use bevy_math::UVec2; | ||
use bevy_reflect::prelude::*; | ||
use bevy_reflect::FromReflect; | ||
use bevy_utils::HashMap; | ||
use wgpu::TextureFormat; | ||
|
||
/// A unique id that corresponds to a specific [`ManualTextureView`] in the [`ManualTextureViews`] collection. | ||
#[derive( | ||
Default, | ||
Debug, | ||
Clone, | ||
Copy, | ||
PartialEq, | ||
Eq, | ||
Hash, | ||
PartialOrd, | ||
Ord, | ||
Component, | ||
Reflect, | ||
FromReflect, | ||
)] | ||
#[reflect(Component, Default)] | ||
pub struct ManualTextureViewHandle(pub u32); | ||
|
||
/// A manually managed [`TextureView`] for use as a [`crate::camera::RenderTarget`]. | ||
#[derive(Debug, Clone, Component)] | ||
pub struct ManualTextureView { | ||
pub texture_view: TextureView, | ||
pub size: UVec2, | ||
pub format: TextureFormat, | ||
} | ||
|
||
impl ManualTextureView { | ||
pub fn with_default_format(texture_view: TextureView, size: UVec2) -> Self { | ||
Self { | ||
texture_view, | ||
size, | ||
format: TextureFormat::bevy_default(), | ||
} | ||
} | ||
} | ||
|
||
/// Stores manually managed [`ManualTextureView`]s for use as a [`crate::camera::RenderTarget`]. | ||
#[derive(Default, Clone, Resource, ExtractResource)] | ||
pub struct ManualTextureViews(HashMap<ManualTextureViewHandle, ManualTextureView>); | ||
|
||
impl std::ops::Deref for ManualTextureViews { | ||
type Target = HashMap<ManualTextureViewHandle, ManualTextureView>; | ||
|
||
fn deref(&self) -> &Self::Target { | ||
&self.0 | ||
} | ||
} | ||
|
||
impl std::ops::DerefMut for ManualTextureViews { | ||
fn deref_mut(&mut self) -> &mut Self::Target { | ||
&mut self.0 | ||
} | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters