-
Notifications
You must be signed in to change notification settings - Fork 267
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
Showing
50 changed files
with
303 additions
and
2,213 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,23 +1,23 @@ | ||
"""Mesh smoothing with two different methods""" | ||
from vedo import Plotter, dataurl | ||
|
||
vp = Plotter(N=3) | ||
plt = Plotter(N=3) | ||
|
||
# Load a mesh and show it | ||
vol = vp.load(dataurl+"embryo.tif") | ||
vol = plt.load(dataurl+"embryo.tif") | ||
m0 = vol.isosurface().normalize().lw(0.1).c("violet") | ||
vp.show(m0, __doc__, at=0) | ||
plt.show(m0, __doc__+"\nOriginal Mesh:", at=0) | ||
|
||
# Adjust mesh using Laplacian smoothing | ||
m1 = m0.clone().smoothLaplacian(niter=20, relaxfact=0.1, edgeAngle=15, featureAngle=60) | ||
m1.color("pink").legend("laplacian") | ||
vp.show(m1, at=1) | ||
m1.color("pink") | ||
plt.show(m1, "Laplacian smoothing", at=1, viewup='z') | ||
|
||
# Adjust mesh using a windowed sinc function interpolation kernel | ||
m2 = m0.clone().smoothWSinc(niter=15, passBand=0.1, edgeAngle=15, featureAngle=60) | ||
m2.color("lg").legend("window sinc") | ||
vp.show(m2, at=2) | ||
m2 = m0.clone().smoothWSinc(niter=20, passBand=0.1, edgeAngle=15, featureAngle=60) | ||
m2.color("lg") | ||
plt.show(m2, "WindowSinc smoothing", at=2) | ||
|
||
vp.backgroundColor([0.8, 1, 1], at=0) # set first renderer color | ||
plt.backgroundColor([0.8, 1, 1], at=0) # set first renderer color | ||
|
||
vp.show(zoom=1.4, interactive=True) | ||
plt.show(zoom=1.4, interactive=True) |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,17 +1,24 @@ | ||
"""Click a sphere to highlight it""" | ||
from vedo import Sphere, Plotter | ||
from vedo import Text2D, Sphere, Plotter | ||
import numpy as np | ||
|
||
pts = np.random.rand(30,2)*20 | ||
spheres = [Sphere().pos(p).color('k5') for p in pts] | ||
spheres = [] | ||
for i in range(25): | ||
p = np.random.rand(2) | ||
s = Sphere(r=0.05).pos(p).color('k5') | ||
s.name = f"sphere nr.{i} at {p}" | ||
spheres.append(s) | ||
|
||
def func(evt): | ||
if not evt.actor: return | ||
sil = evt.actor.silhouette().lineWidth(6).c('red5') | ||
msg.text("You clicked: "+evt.actor.name) | ||
plt.remove(silcont.pop()).add(sil) | ||
silcont.append(sil) | ||
|
||
silcont = [None] | ||
msg = Text2D("", pos="bottom-center", c='k', bg='r9', alpha=0.8) | ||
|
||
plt = Plotter(axes=1, bg='black') | ||
plt.addCallback('mouse click', func) | ||
plt.show(spheres, __doc__, zoom=1.2) | ||
plt.show(spheres, msg, __doc__, zoom=1.2) |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,28 +1,31 @@ | ||
"""Visualize scalar values interactively | ||
by hovering the mouse on a mesh | ||
Press c to clear""" | ||
Press c to clear the path""" | ||
from vedo import * | ||
|
||
def func(evt): ### called every time the mouse moves! | ||
if not evt.actor: return # no hits, return. (NB: evt is a dictionary) | ||
pt = evt.picked3d # 3d coords of picked point under mouse | ||
def func(evt): ### called every time the mouse moves! | ||
if not evt.actor: return # no hits, return. (NB: evt is a dictionary) | ||
pt = evt.picked3d # 3d coords of picked point under mouse | ||
|
||
pid = evt.actor.closestPoint(pt, returnPointId=True) | ||
txt = f"Point: {precision(pt[:2],2)}\n" \ | ||
f"Height: {precision(arr[pid],3)}\n"\ | ||
txt = f"Point: {precision(pt[:2] ,2)}\n" \ | ||
f"Height: {precision(arr[pid],3)}\n" \ | ||
f"Ground speed: {precision(evt.speed3d*100,2)}" | ||
arw = Arrow(pt - evt.delta3d, pt, s=0.001, c='orange5') | ||
vig = evt.actor.vignette(txt, point=pt, offset=(0.4,0.6), | ||
s=0.04, c='k', font="VictorMono").followCamera() | ||
msg = Text2D(txt, pos='bottom-left', font="VictorMono") | ||
if len(plt.actors) > 3: | ||
plt.remove(plt.actors[-2:]) # remove the old vig and msg | ||
plt.add([arw, vig, msg]) # add Arrow and the new vig and msg | ||
msg.text(txt) # update text message | ||
if len(plt.actors)>3: | ||
plt.pop() # remove the old vignette | ||
plt.add([arw, vig]) # add Arrow and the new vig | ||
|
||
hil = ParametricShape('RandomHills').cmap('terrain').addScalarBar() | ||
arr = hil.getPointArray("Scalars") | ||
|
||
plt = Plotter(axes=1, bg2='lightblue') | ||
plt.addCallback('MouseMove', func) # the callback function | ||
plt.addCallback('KeyPress', lambda e: plt.remove(plt.actors[2:], render=True)) | ||
plt.show(hil, __doc__, viewup='z') | ||
plt.addCallback('mouse moving', func) # the callback function | ||
plt.addCallback('keyboard', lambda e: plt.remove(plt.actors[3:], render=True)) | ||
|
||
msg = Text2D("", pos='bottom-left', font="VictorMono") | ||
|
||
plt.show(hil, msg, __doc__, viewup='z') |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,15 +1,17 @@ | ||
"""Normal jpg/png pictures can be loaded, | ||
cropped, rotated and positioned in 3D | ||
""" | ||
from vedo import Plotter, dataurl | ||
cropped, rotated and positioned in 3D.""" | ||
from vedo import Plotter, Picture, dataurl | ||
|
||
vp = Plotter(axes=3) | ||
plt = Plotter(axes=7) | ||
|
||
pic = Picture(dataurl+"images/dog.jpg") | ||
|
||
for i in range(5): | ||
p = vp.load(dataurl+"images/dog.jpg") # returns Picture | ||
p.crop(bottom=0.2) # crop 20% | ||
p.scale(1-i/10.0).alpha(0.8) # picture can be scaled in size | ||
p.rotateX(20*i).pos(0,0,30*i) # (can concatenate methods) | ||
p = pic.clone() | ||
p.crop(bottom=0.20) # crop 20% from bottom | ||
p.scale(1-i/10.0).rotateX(20*i).z(30*i) | ||
p.alpha(0.8) | ||
plt += p | ||
|
||
vp += __doc__ | ||
vp.show() | ||
plt += __doc__ | ||
plt.show() |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,14 +1,16 @@ | ||
# Jupyter Notebooks | ||
|
||
Here you will find a set of notebooks. | ||
It allows to embed a redering window into a jupyter notebook via | ||
Here you will find a set of notebooks. | ||
It allows to embed a redering window into a jupyter notebook via | ||
[K3D](https://github.com/K3D-tools/K3D-jupyter) | ||
(or optionally with `itkwidgets` or [panel](https://github.com/pyviz/panel)). | ||
(or optionally with `itkwidgets`, `ipyvtk` or [panel](https://github.com/pyviz/panel)). | ||
|
||
On top of this `K3D` allows to export an interactive | ||
[snapshot](https://vedo.embl.es/examples/K3D_snapshot.html) | ||
of the rendered scene. | ||
|
||
`jupyter notebook examples/notebooks/basic/embryo.ipynb` | ||
### note that notebook support (except for `ipyvtk`) is rather limited and not all functionalities are available, in particular: | ||
|
||
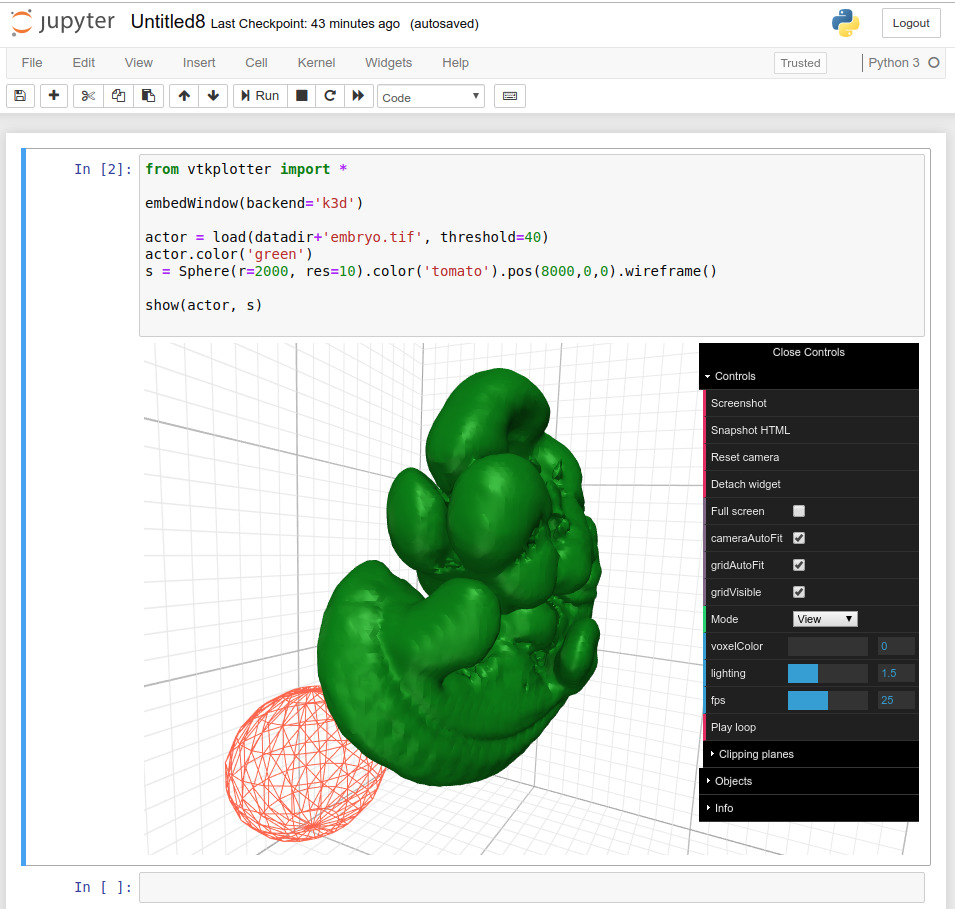 | ||
- axes are very basic | ||
- text2d is not available | ||
- limited possibilities for scene interaction |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Oops, something went wrong.